Race car Lap Simulation#
Things you’ll learn through this example
Optimizing trajectories for cyclic events (such as race car laps)
Linking a phase with itself so it is ensured that start and end states are identical
Using an independent variable other than time
The race track problem
The race track problem is different from other dymos example problems in that it is a cyclic problem. The solution must be such that the start and end states are identical. The problem for this example is given a race track shape, what is the optimum values for the controls such that the time elapsed to complete the track is a minimum.
Background of the problem#
This example is based on the work of Pieter de Buck The goal of his problem was to find an optimal trajectory for the race car with active aerodynamic and four wheel drive controls.
Pieter posted an issue to the Dymos repository asking how to use dymos for cyclic events such as this. His solution involved using an iterative solution, which worked but dymos includes a feature that allows for simple and more efficient solution. This example shows how to handle this situation using simple dymos calls without iteration.
If you are interested, Pieter and Adeline Shin have created an interactive visualization using his version of the code.
While Pieter’s code includes several actual race tracks shapes, for simplicity and speed of solution, this dymos example uses an oval track. If you are interested in trying other race tracks, they are defined in the tracks.py file.
Optimizing trajectories for cyclic events#
For trajectory optimization of cyclic events (such as race car laps), there needs to be a way that ensure that the start and end states are identical.
In this race car example, the states and controls such as velocity, thrust, and steering angle need to be the same at the start and finish.
Linking the controls is not really needed since it generally follows from the linked states.
There is a simple way to link the states of cyclic events in dymos.
You can use the Trajectory.link_phases
functionality to “link” a
phase with itself. In this example this is accomplished with the following code which links all 7 state
variables.
traj.link_phases(phases=['phase0', 'phase0'], vars=['V','n','alpha','omega','lambda','ax','ay'], locs=('final', 'initial'))
This code causes the value of V
at the end of phase0
(‘final’) to be linked via constraint
to the value of V
at the beginning (‘initial’) of phase0
.
This call does the same for the other 6 state variables as well.
See the Trajectory API page
for more information about the link_phases
method.
Using an independent variable other than time#
In all the other dymos examples, time is the independent variable. In this race car example, the independent variable is the arc length along the track. Integration is done from the start of the track to the end. The “typical” equations of motion are computed, so the rate of change of x wrt time (\(\frac{dx}{dt}\)).
By dividing the rates of change w.r.t. time by (\(\frac{ds}{dt}\)), the rates of change of the states w.r.t. track arclength traversed are computed.
Definition of the problem#
Here are some figures which help define the problem.
This table describes the controls, states, and constraints. The symbols match up with the variable names in the code shown at the bottom of this page.
Table 1. Optimization problem formulation#
Symbol |
Lower |
Upper |
Units |
||
---|---|---|---|---|---|
Minimize |
Time |
t |
s |
||
With respect to |
|||||
Controls |
Front left thrust |
\(T_{fl}\) |
|||
Front right thrust |
\(T_{fr}\) |
||||
Rear left thrust |
\(T_{rl}\) |
||||
Rear right thrust |
\(T_{rr}\) |
||||
Rear wing flap angle |
\(\gamma\) |
0 |
50 |
deg |
|
Steering angle |
\(\delta\) |
rad |
|||
States |
Speed |
V |
\(ms^{-1}\) |
||
Longitudinal acceleration |
\(a_x\) |
\(ms^{-2}\) |
|||
Lateral acceleration |
\(a_y\) |
\(ms^{-2}\) |
|||
Normal distance to centerline |
n |
-4 |
4 |
m |
|
Angle relative to centerline |
\(\alpha\) |
rad |
|||
Slip angle |
\(\lambda\) |
rad |
|||
Yaw rate |
\(\Omega\) |
\(rad^{-1}\) |
|||
Subject to |
Front left adherence |
\(c_{fl}\) |
0 |
1 |
|
Front right adherence |
\(c_{fr}\) |
0 |
1 |
||
Rear left adherence |
\(c_{rl}\) |
0 |
1 |
||
Rear right adherence |
\(c_{rr}\) |
0 |
1 |
||
Front left power |
\(P_{fl}\) |
75 |
kW |
||
Front right power |
\(P_{fr}\) |
75 |
kW |
||
Rear left power |
\(P_{rl}\) |
75 |
kW |
||
Rear right power |
\(P_{rr}\) |
75 |
kW |
||
Periodic state constraints |
|||||
System dynamics |
|||||
Track Layout |
The next figure defines the vehicle parameters. Again, the symbols match up with the variable names in the code.
Table 2. Vehicle parameters#
Parameter |
Value |
Units |
Description |
---|---|---|---|
\(M\) |
1184 |
\(kg\) |
Vehicle mass |
\(a\) |
1.404 |
\(m\) |
CoG to front axle distance |
\(b\) |
1.356 |
\(m\) |
CoG to rear axle distance |
\(t_w\) |
0.807 |
\(m\) |
Half track width |
\(h\) |
0.4 |
\(m\) |
CoG height |
\(I_z\) |
1775 |
\(kg\ m^{2}\) |
Yaw inertia |
\(\beta\) |
0.62 |
- |
Brake balance |
\(\chi\) |
0.5 |
- |
Roll stiffness |
\(\rho\) |
1.2 |
\(kg\ m^{-3}\) |
Air density |
\(\mu_{0}^{x}\) |
1.68 |
- |
Longitudinal base friction coefficient |
\(\mu_{0}^{y}\) |
1.68 |
- |
Lateral base friction coefficient |
\(K_{\mu}\) |
-0.5 |
- |
Tire load sensitivity |
\(K_{\lambda}\) |
44 |
- |
Tire lateral stiffness |
\(\tau_{a_{x}}\) |
0.2 |
\(s\) |
Longitudinal load transfer time constant |
\(\tau_{a_{y}}\) |
0.2 |
\(s\) |
Lateral load transfer time constant |
\(S_{w}\) |
0.8 |
\(m^{2}\) |
Wing planform area |
\(CoP\) |
1.404 |
\(m\) |
Center of pressure to front axle distance |
There is also a figure which describes the states that govern the vehicle position relative to the track.
Here is the code to solve this problem:
Show code cell outputs
import openmdao.api as om
from .carODE import CarODE
from .tireODE import TireODE
from .normalForceODE import NormalForceODE
from .accelerationODE import AccelerationODE
from .tireConstraintODE import TireConstraintODE
from .timeODE import TimeODE
from .timeAdder import TimeAdder
from .curvature import Curvature
class CombinedODE(om.Group):
def initialize(self):
self.options.declare("num_nodes", types=int,
desc="Number of nodes to be evaluated in the RHS")
def setup(self):
nn = self.options["num_nodes"]
self.add_subsystem(name="normal", subsys=NormalForceODE(num_nodes=nn),
promotes_inputs=["ax", "ay", "V"],
promotes_outputs=["N_fr", "N_fl", "N_rr", "N_rl"], )
self.add_subsystem(name="tire", subsys=TireODE(num_nodes=nn),
promotes_inputs=["lambda", "omega", "V", "N_fr", "N_fl", "N_rr", "N_rl",
"thrust", "delta", ],
promotes_outputs=["S_fr", "S_fl", "S_rr", "S_rl", "F_fr", "F_fl", "F_rr",
"F_rl", ], )
self.add_subsystem(name="curv", subsys=Curvature(num_nodes=nn))
self.connect("curv.kappa", "car.kappa")
self.add_subsystem(name="car", subsys=CarODE(num_nodes=nn),
promotes_inputs=["n", "alpha", "V", "lambda", "omega", "S_fr", "S_fl",
"S_rr", "S_rl", "F_fr", "F_fl", "F_rr", "F_rl",
"delta", ],
promotes_outputs=["sdot", "ndot", "alphadot", "omegadot", "Vdot",
"lambdadot", "power", ], )
self.add_subsystem(name="accel", subsys=AccelerationODE(num_nodes=nn),
promotes_inputs=["V", "lambda", "omega", "Vdot", "lambdadot", "ax",
"ay"], promotes_outputs=["axdot", "aydot"], )
self.add_subsystem(name="tireconstraint", subsys=TireConstraintODE(num_nodes=nn),
promotes_inputs=["S_fr", "S_fl", "S_rr", "S_rl", "F_fr", "F_fl", "F_rr",
"F_rl", "N_fr", "N_fl", "N_rr", "N_rl", ],
promotes_outputs=["c_rr", "c_rl", "c_fr", "c_fl"], )
self.add_subsystem(name="time", subsys=TimeODE(num_nodes=nn),
promotes_inputs=["ndot", "sdot", "omegadot", "lambdadot", "Vdot",
"axdot", "aydot", "alphadot", ],
promotes_outputs=["dn_ds", "dV_ds", "domega_ds", "dlambda_ds",
"dalpha_ds", "dax_ds", "day_ds", ], )
self.add_subsystem(name="timeAdder", subsys=TimeAdder(num_nodes=nn),
promotes_inputs=["sdot"], promotes_outputs=["dt_ds"], )
import numpy as np
import openmdao.api as om
import dymos as dm
import matplotlib.pyplot as plt
import matplotlib as mpl
from dymos.examples.racecar.combinedODE import CombinedODE
from dymos.examples.racecar.spline import (get_spline, get_track_points, get_gate_normals,
reverse_transform_gates, set_gate_displacements,
transform_gates, )
from dymos.examples.racecar.linewidthhelper import linewidth_from_data_units
from dymos.examples.racecar.tracks import ovaltrack # track curvature imports
# change track here and in curvature.py. Tracks are defined in tracks.py
track = ovaltrack
# generate nodes along the centerline for curvature calculation (different
# than collocation nodes)
points = get_track_points(track)
# fit the centerline spline.
finespline, gates, gatesd, curv, slope = get_spline(points, s=0.0)
# by default 10000 points
s_final = track.get_total_length()
# Define the OpenMDAO problem
p = om.Problem(model=om.Group())
# Define a Trajectory object
traj = dm.Trajectory()
p.model.add_subsystem('traj', subsys=traj)
# Define a Dymos Phase object with GaussLobatto Transcription
phase = dm.Phase(ode_class=CombinedODE,
transcription=dm.GaussLobatto(num_segments=50, order=3, compressed=True))
traj.add_phase(name='phase0', phase=phase)
# Set the time options, in this problem we perform a change of variables. So 'time' is
# actually 's' (distance along the track centerline)
# This is done to fix the collocation nodes in space, which saves us the calculation of
# the rate of change of curvature.
# The state equations are written with respect to time, the variable change occurs in
# timeODE.py
# Here we're using set_integ_var_options, which is just an alias for set_time_options.
phase.set_integ_var_options(fix_initial=True, fix_duration=True, duration_val=s_final,
targets=['curv.s'], units='m', duration_ref=s_final,
duration_ref0=10, name='s')
# Define states
phase.add_state('t', fix_initial=True, fix_final=False, units='s', lower=0,
rate_source='dt_ds', ref=100) # time
phase.add_state('n', fix_initial=False, fix_final=False, units='m', upper=4.0, lower=-4.0,
rate_source='dn_ds', targets=['n'],
ref=4.0) # normal distance to centerline. The bounds on n define the
# width of the track
phase.add_state('V', fix_initial=False, fix_final=False, units='m/s', ref=40, ref0=5,
rate_source='dV_ds', targets=['V']) # velocity
phase.add_state('alpha', fix_initial=False, fix_final=False, units='rad',
rate_source='dalpha_ds', targets=['alpha'],
ref=0.15) # vehicle heading angle with respect to centerline
phase.add_state('lambda', fix_initial=False, fix_final=False, units='rad',
rate_source='dlambda_ds', targets=['lambda'],
ref=0.01) # vehicle slip angle, or angle between the axis of the vehicle
# and velocity vector (all cars drift a little)
phase.add_state('omega', fix_initial=False, fix_final=False, units='rad/s',
rate_source='domega_ds', targets=['omega'], ref=0.3) # yaw rate
phase.add_state('ax', fix_initial=False, fix_final=False, units='m/s**2',
rate_source='dax_ds', targets=['ax'], ref=8) # longitudinal acceleration
phase.add_state('ay', fix_initial=False, fix_final=False, units='m/s**2',
rate_source='day_ds', targets=['ay'], ref=8) # lateral acceleration
# Define Controls
phase.add_control(name='delta', units='rad', lower=None, upper=None, fix_initial=False,
fix_final=False, ref=0.04, rate_continuity=True) # steering angle
phase.add_control(name='thrust', units=None, fix_initial=False, fix_final=False, rate_continuity=True)
# the thrust controls the longitudinal force of the rear tires and is positive while accelerating, negative while braking
# Performance Constraints
pmax = 960000 # W
phase.add_path_constraint('power', upper=pmax, ref=100000) # engine power limit
# The following four constraints are the tire friction limits, with 'rr' designating the
# rear right wheel etc. This limit is computed in tireConstraintODE.py
phase.add_path_constraint('c_rr', upper=1)
phase.add_path_constraint('c_rl', upper=1)
phase.add_path_constraint('c_fr', upper=1)
phase.add_path_constraint('c_fl', upper=1)
# Some of the vehicle design parameters are available to set here. Other parameters can
# be found in their respective ODE files.
phase.add_parameter('M', val=800.0, units='kg', opt=False,
targets=['car.M', 'tire.M', 'tireconstraint.M', 'normal.M'],
static_target=True) # vehicle mass
phase.add_parameter('beta', val=0.62, units=None, opt=False, targets=['tire.beta'],
static_target=True) # brake bias
phase.add_parameter('CoP', val=1.6, units='m', opt=False, targets=['normal.CoP'],
static_target=True) # center of pressure location
phase.add_parameter('h', val=0.3, units='m', opt=False, targets=['normal.h'],
static_target=True) # center of gravity height
phase.add_parameter('chi', val=0.5, units=None, opt=False, targets=['normal.chi'],
static_target=True) # roll stiffness
phase.add_parameter('ClA', val=4.0, units='m**2', opt=False, targets=['normal.ClA'],
static_target=True) # downforce coefficient*area
phase.add_parameter('CdA', val=2.0, units='m**2', opt=False, targets=['car.CdA'],
static_target=True) # drag coefficient*area
# Minimize final time.
# note that we use the 'state' time instead of Dymos 'time'
phase.add_objective('t', loc='final')
# Add output timeseries
phase.add_timeseries_output('*')
phase.add_timeseries_output('t', output_name='time')
# Link the states at the start and end of the phase in order to ensure a continous lap
traj.link_phases(phases=['phase0', 'phase0'],
vars=['V', 'n', 'alpha', 'omega', 'lambda', 'ax', 'ay'],
locs=('final', 'initial'))
# Set the driver. IPOPT or SNOPT are recommended but SLSQP might work.
p.driver = om.pyOptSparseDriver(optimizer='IPOPT')
p.driver.opt_settings['mu_init'] = 1e-3
p.driver.opt_settings['max_iter'] = 500
p.driver.opt_settings['acceptable_tol'] = 1e-3
p.driver.opt_settings['constr_viol_tol'] = 1e-3
p.driver.opt_settings['compl_inf_tol'] = 1e-3
p.driver.opt_settings['acceptable_iter'] = 0
p.driver.opt_settings['tol'] = 1e-3
p.driver.opt_settings['print_level'] = 0
p.driver.opt_settings['nlp_scaling_method'] = 'gradient-based' # for faster convergence
p.driver.opt_settings['alpha_for_y'] = 'safer-min-dual-infeas'
p.driver.opt_settings['mu_strategy'] = 'monotone'
p.driver.opt_settings['bound_mult_init_method'] = 'mu-based'
p.driver.options['print_results'] = False
# Allow OpenMDAO to automatically determine our sparsity pattern.
# Doing so can significant speed up the execution of Dymos.
p.driver.declare_coloring()
# Setup the problem
p.setup(check=True) # force_alloc_complex=True
# Now that the OpenMDAO problem is setup, we can set the values of the states.
# States
# Nonzero velocity to avoid division by zero errors
phase.set_state_val('V', 20.0, units='m/s')
# All other states start at 0
phase.set_state_val('lambda', 0.0, units='rad')
phase.set_state_val('omega', 0.0, units='rad/s')
phase.set_state_val('alpha', 0.0, units='rad')
phase.set_state_val('ax', 0.0, units='m/s**2')
phase.set_state_val('ay', 0.0, units='m/s**2')
phase.set_state_val('n', 0.0, units='m')
# initial guess for what the final time should be
phase.set_state_val('t', [0.0, 100.0], units='s')
# Controls
# a small amount of thrust can speed up convergence
phase.set_control_val('delta', 0.0, units='rad')
phase.set_control_val('thrust', 0.1, units=None)
dm.run_problem(p, run_driver=True)
print('Optimization finished')
# Get optimized time series
n = p.get_val('traj.phase0.timeseries.n')
s = p.get_val('traj.phase0.timeseries.s')
V = p.get_val('traj.phase0.timeseries.V')
thrust = p.get_val('traj.phase0.timeseries.thrust')
delta = p.get_val('traj.phase0.timeseries.delta')
power = p.get_val('traj.phase0.timeseries.power', units='W')
/usr/share/miniconda/envs/test/lib/python3.11/site-packages/dymos/phase/phase.py:2337: UserWarning: Phase time options have no effect because fix_duration=True or input_duration=True for phase 'traj.phases.phase0': duration_ref, duration_ref0
warnings.warn(f'Phase time options have no effect because fix_duration=True '
INFO: checking out_of_order...
INFO: out_of_order check complete (0.001112 sec).
INFO: checking system...
INFO: system check complete (0.000042 sec).
INFO: checking solvers...
INFO: solvers check complete (0.000355 sec).
INFO: checking dup_inputs...
INFO: dup_inputs check complete (0.000303 sec).
INFO: checking missing_recorders...
INFO: missing_recorders check complete (0.000002 sec).
INFO: checking unserializable_options...
INFO: unserializable_options check complete (0.001977 sec).
INFO: checking comp_has_no_outputs...
INFO: comp_has_no_outputs check complete (0.000087 sec).
INFO: checking auto_ivc_warnings...
INFO: auto_ivc_warnings check complete (0.000003 sec).
INFO: checking out_of_order...
INFO: out_of_order check complete (0.000524 sec).
INFO: checking system...
INFO: system check complete (0.000035 sec).
INFO: checking solvers...
INFO: solvers check complete (0.000195 sec).
INFO: checking dup_inputs...
INFO: dup_inputs check complete (0.000290 sec).
INFO: checking missing_recorders...
INFO: missing_recorders check complete (0.000002 sec).
INFO: checking unserializable_options...
INFO: unserializable_options check complete (0.002228 sec).
INFO: checking comp_has_no_outputs...
INFO: comp_has_no_outputs check complete (0.000110 sec).
INFO: checking auto_ivc_warnings...
INFO: auto_ivc_warnings check complete (0.000002 sec).
INFO: checking out_of_order...
INFO: out_of_order check complete (0.000461 sec).
INFO: checking system...
INFO: system check complete (0.000036 sec).
INFO: checking solvers...
INFO: solvers check complete (0.000302 sec).
INFO: checking dup_inputs...
INFO: dup_inputs check complete (0.000565 sec).
INFO: checking missing_recorders...
INFO: missing_recorders check complete (0.000002 sec).
INFO: checking unserializable_options...
INFO: unserializable_options check complete (0.002070 sec).
INFO: checking comp_has_no_outputs...
INFO: comp_has_no_outputs check complete (0.000109 sec).
INFO: checking auto_ivc_warnings...
INFO: auto_ivc_warnings check complete (0.000003 sec).
Jacobian shape: (1256, 609) (2.08% nonzero)
FWD solves: 20 REV solves: 0
Total colors vs. total size: 20 vs 609 (96.72% improvement)
Sparsity computed using tolerance: 1e-25.
Dense total jacobian for Problem 'problem' was computed 3 times.
Time to compute sparsity: 8.2658 sec
Time to compute coloring: 1.2410 sec
Memory to compute coloring: -7.1328 MB
Coloring created on: 2025-04-10 15:43:39
/usr/share/miniconda/envs/test/lib/python3.11/site-packages/openmdao/core/total_jac.py:1730: DerivativesWarning:Constraints or objectives [('traj.phase0.c_fr[path]', inds=[(0, 0), (1, 0), (2, 0), (3, 0), (4, 0), (5, 0), (6, 0), (7, 0), (8, 0), (9, 0), (10, 0), (11, 0), (12, 0), (13, 0), (14, 0), (15, 0), (16, 0), (17, 0), (18, 0), (19, 0), (20, 0), (21, 0), (22, 0), (23, 0), (24, 0), (25, 0), (26, 0), (27, 0), (28, 0), (29, 0), (30, 0), (31, 0), (32, 0), (33, 0), (34, 0), (35, 0), (36, 0), (37, 0), (38, 0), (39, 0), (40, 0), (41, 0), (42, 0), (43, 0), (44, 0), (45, 0), (46, 0), (47, 0), (48, 0), (49, 0), (50, 0), (51, 0), (52, 0), (53, 0), (54, 0), (55, 0), (56, 0), (57, 0), (58, 0), (59, 0), (60, 0), (61, 0), (62, 0), (63, 0), (64, 0), (65, 0), (66, 0), (67, 0), (68, 0), (69, 0), (70, 0), (71, 0), (72, 0), (73, 0), (74, 0), (75, 0), (76, 0), (77, 0), (78, 0), (79, 0), (80, 0), (81, 0), (82, 0), (83, 0), (84, 0), (85, 0), (86, 0), (87, 0), (88, 0), (89, 0), (90, 0), (91, 0), (92, 0), (93, 0), (94, 0), (95, 0), (96, 0), (97, 0), (98, 0), (99, 0), (100, 0), (101, 0), (102, 0), (103, 0), (104, 0), (105, 0), (106, 0), (107, 0), (108, 0), (109, 0), (110, 0), (111, 0), (112, 0), (113, 0), (114, 0), (115, 0), (116, 0), (117, 0), (118, 0), (119, 0), (120, 0), (121, 0), (122, 0), (123, 0), (124, 0), (125, 0), (126, 0), (127, 0), (128, 0), (129, 0), (130, 0), (131, 0), (132, 0), (133, 0), (134, 0), (135, 0), (136, 0), (137, 0), (138, 0), (139, 0), (140, 0), (141, 0), (142, 0), (143, 0), (144, 0), (145, 0), (146, 0), (147, 0), (148, 0), (149, 0)]), ('traj.phase0.c_fl[path]', inds=[(0, 0), (1, 0), (2, 0), (3, 0), (4, 0), (5, 0), (6, 0), (7, 0), (8, 0), (9, 0), (10, 0), (11, 0), (12, 0), (13, 0), (14, 0), (15, 0), (16, 0), (17, 0), (18, 0), (19, 0), (20, 0), (21, 0), (22, 0), (23, 0), (24, 0), (25, 0), (26, 0), (27, 0), (28, 0), (29, 0), (30, 0), (31, 0), (32, 0), (33, 0), (34, 0), (35, 0), (36, 0), (37, 0), (38, 0), (39, 0), (40, 0), (41, 0), (42, 0), (43, 0), (44, 0), (45, 0), (46, 0), (47, 0), (48, 0), (49, 0), (50, 0), (51, 0), (52, 0), (53, 0), (54, 0), (55, 0), (56, 0), (57, 0), (58, 0), (59, 0), (60, 0), (61, 0), (62, 0), (63, 0), (64, 0), (65, 0), (66, 0), (67, 0), (68, 0), (69, 0), (70, 0), (71, 0), (72, 0), (73, 0), (74, 0), (75, 0), (76, 0), (77, 0), (78, 0), (79, 0), (80, 0), (81, 0), (82, 0), (83, 0), (84, 0), (85, 0), (86, 0), (87, 0), (88, 0), (89, 0), (90, 0), (91, 0), (92, 0), (93, 0), (94, 0), (95, 0), (96, 0), (97, 0), (98, 0), (99, 0), (100, 0), (101, 0), (102, 0), (103, 0), (104, 0), (105, 0), (106, 0), (107, 0), (108, 0), (109, 0), (110, 0), (111, 0), (112, 0), (113, 0), (114, 0), (115, 0), (116, 0), (117, 0), (118, 0), (119, 0), (120, 0), (121, 0), (122, 0), (123, 0), (124, 0), (125, 0), (126, 0), (127, 0), (128, 0), (129, 0), (130, 0), (131, 0), (132, 0), (133, 0), (134, 0), (135, 0), (136, 0), (137, 0), (138, 0), (139, 0), (140, 0), (141, 0), (142, 0), (143, 0), (144, 0), (145, 0), (146, 0), (147, 0), (148, 0), (149, 0)])] cannot be impacted by the design variables of the problem.
Optimization finished
# Get optimized time series
n = p.get_val('traj.phase0.timeseries.n')
s = p.get_val('traj.phase0.timeseries.s')
V = p.get_val('traj.phase0.timeseries.V')
thrust = p.get_val('traj.phase0.timeseries.thrust')
delta = p.get_val('traj.phase0.timeseries.delta')
power = p.get_val('traj.phase0.timeseries.power', units='W')
# We know the optimal distance from the centerline (n). To transform this into the racing
# line we fit a spline to the displaced points. This will let us plot the racing line in
# x/y coordinates
normals = get_gate_normals(finespline, slope)
newgates = []
newnormals = []
newn = []
for i in range(len(n)):
index = ((s[i] / s_final) * np.array(finespline).shape[1]).astype(
int) # interpolation to find the appropriate index
if index[0] == np.array(finespline).shape[1]:
index[0] = np.array(finespline).shape[1] - 1
if i > 0 and s[i] == s[i - 1]:
continue
else:
newgates.append([finespline[0][index[0]], finespline[1][index[0]]])
newnormals.append(normals[index[0]])
newn.append(n[i][0])
newgates = reverse_transform_gates(newgates)
displaced_gates = set_gate_displacements(newn, newgates, newnormals)
displaced_gates = np.array((transform_gates(displaced_gates)))
npoints = 1000
# fit the racing line spline to npoints
displaced_spline, gates, gatesd, curv, slope = get_spline(displaced_gates, 1 / npoints, 0)
plt.rcParams.update({'font.size': 12})
def plot_track_with_data(state, s):
# this function plots the track
state = np.array(state)[:, 0]
s = np.array(s)[:, 0]
s_new = np.linspace(0, s_final, npoints)
# Colormap and norm of the track plot
cmap = mpl.colormaps['viridis']
norm = mpl.colors.Normalize(vmin=np.amin(state), vmax=np.amax(state))
fig, ax = plt.subplots(figsize=(15, 6))
# establishes the figure axis limits needed for plotting the track below
plt.plot(displaced_spline[0], displaced_spline[1], linewidth=0.1, solid_capstyle="butt")
plt.axis('equal')
# the linewidth is set in order to match the width of the track
plt.plot(finespline[0], finespline[1], 'k',
linewidth=linewidth_from_data_units(8.5, ax),
solid_capstyle="butt")
plt.plot(finespline[0], finespline[1], 'w', linewidth=linewidth_from_data_units(8, ax),
solid_capstyle="butt") # 8 is the width, and the 8.5 wide line draws 'kerbs'
plt.xlabel('x (m)')
plt.ylabel('y (m)')
# plot spline with color
for i in range(1, len(displaced_spline[0])):
s_spline = s_new[i]
index_greater = np.argwhere(s >= s_spline)[0][0]
index_less = np.argwhere(s < s_spline)[-1][0]
x = s_spline
xp = np.array([s[index_less], s[index_greater]])
fp = np.array([state[index_less], state[index_greater]])
interp_state = np.interp(x, xp,
fp) # interpolate the given state to calculate the color
# calculate the appropriate color
state_color = norm(interp_state)
color = cmap(state_color)
color = mpl.colors.to_hex(color)
# the track plot consists of thousands of tiny lines:
point = [displaced_spline[0][i], displaced_spline[1][i]]
prevpoint = [displaced_spline[0][i - 1], displaced_spline[1][i - 1]]
if i <= 5 or i == len(displaced_spline[0]) - 1:
plt.plot([point[0], prevpoint[0]], [point[1], prevpoint[1]], color,
linewidth=linewidth_from_data_units(1.5, ax), solid_capstyle="butt",
antialiased=True)
else:
plt.plot([point[0], prevpoint[0]], [point[1], prevpoint[1]], color,
linewidth=linewidth_from_data_units(1.5, ax),
solid_capstyle="projecting", antialiased=True)
clb = plt.colorbar(mpl.cm.ScalarMappable(norm=norm, cmap=cmap), fraction=0.02,
ax=ax, pad=0.04) # add colorbar
if np.array_equal(state, V[:, 0]):
clb.set_label('Velocity (m/s)')
elif np.array_equal(state, thrust[:, 0]):
clb.set_label('Thrust')
elif np.array_equal(state, delta[:, 0]):
clb.set_label('Delta')
plt.tight_layout()
plt.grid()
# Create the plots
plot_track_with_data(V, s)
plot_track_with_data(thrust, s)
plot_track_with_data(delta, s)
# Plot the main vehicle telemetry
fig, axes = plt.subplots(nrows=4, ncols=1, figsize=(15, 8))
# Velocity vs s
axes[0].plot(s,
p.get_val('traj.phase0.timeseries.V'), label='solution')
axes[0].set_xlabel('s (m)')
axes[0].set_ylabel('V (m/s)')
axes[0].grid()
axes[0].set_xlim(0, s_final)
# n vs s
axes[1].plot(s,
p.get_val('traj.phase0.timeseries.n', units='m'), label='solution')
axes[1].set_xlabel('s (m)')
axes[1].set_ylabel('n (m)')
axes[1].grid()
axes[1].set_xlim(0, s_final)
# throttle vs s
axes[2].plot(s, thrust)
axes[2].set_xlabel('s (m)')
axes[2].set_ylabel('thrust')
axes[2].grid()
axes[2].set_xlim(0, s_final)
# delta vs s
axes[3].plot(s,
p.get_val('traj.phase0.timeseries.delta', units=None),
label='solution')
axes[3].set_xlabel('s (m)')
axes[3].set_ylabel('delta')
axes[3].grid()
axes[3].set_xlim(0, s_final)
plt.tight_layout()
# Performance constraint plot. Tire friction and power constraints
fig, axes = plt.subplots(nrows=1, ncols=1, figsize=(15, 4))
plt.subplots_adjust(right=0.82, bottom=0.14, top=0.97, left=0.07)
axes.plot(s,
p.get_val('traj.phase0.timeseries.c_fl', units=None), label='c_fl')
axes.plot(s,
p.get_val('traj.phase0.timeseries.c_fr', units=None), label='c_fr')
axes.plot(s,
p.get_val('traj.phase0.timeseries.c_rl', units=None), label='c_rl')
axes.plot(s,
p.get_val('traj.phase0.timeseries.c_rr', units=None), label='c_rr')
axes.plot(s, power / pmax, label='Power')
axes.legend(bbox_to_anchor=(1.04, 0.5), loc="center left")
axes.set_xlabel('s (m)')
axes.set_ylabel('Performance constraints')
axes.grid()
axes.set_xlim(0, s_final)
plt.show()
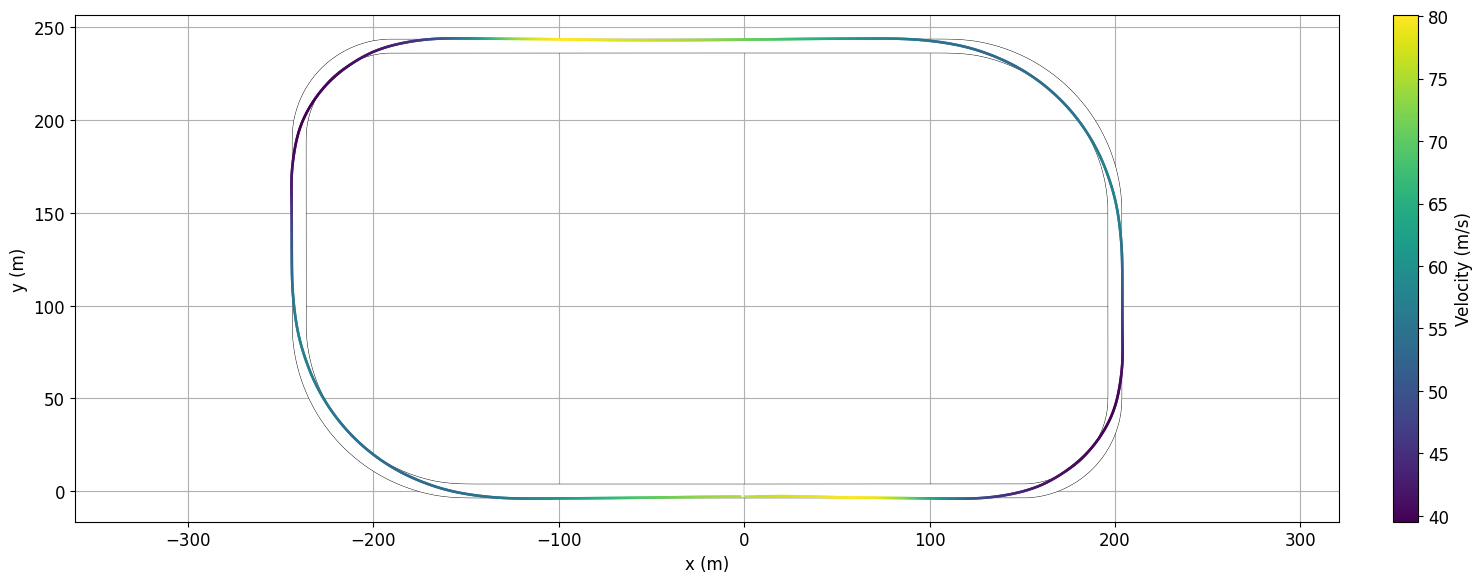
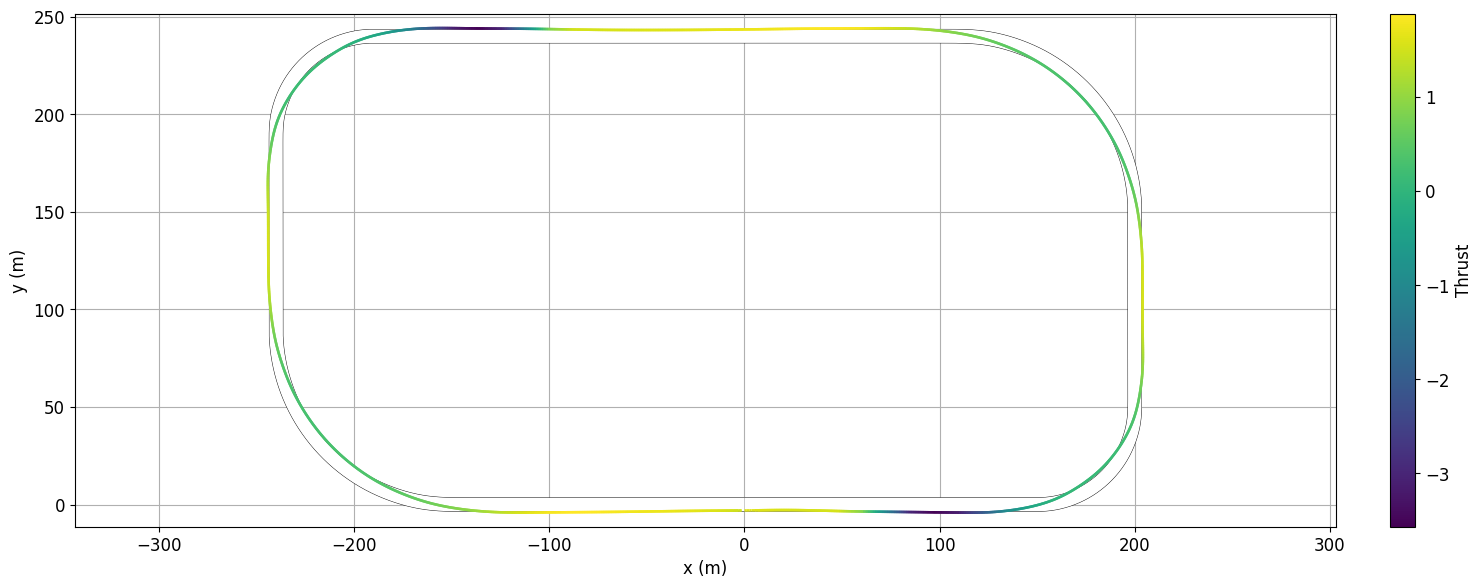
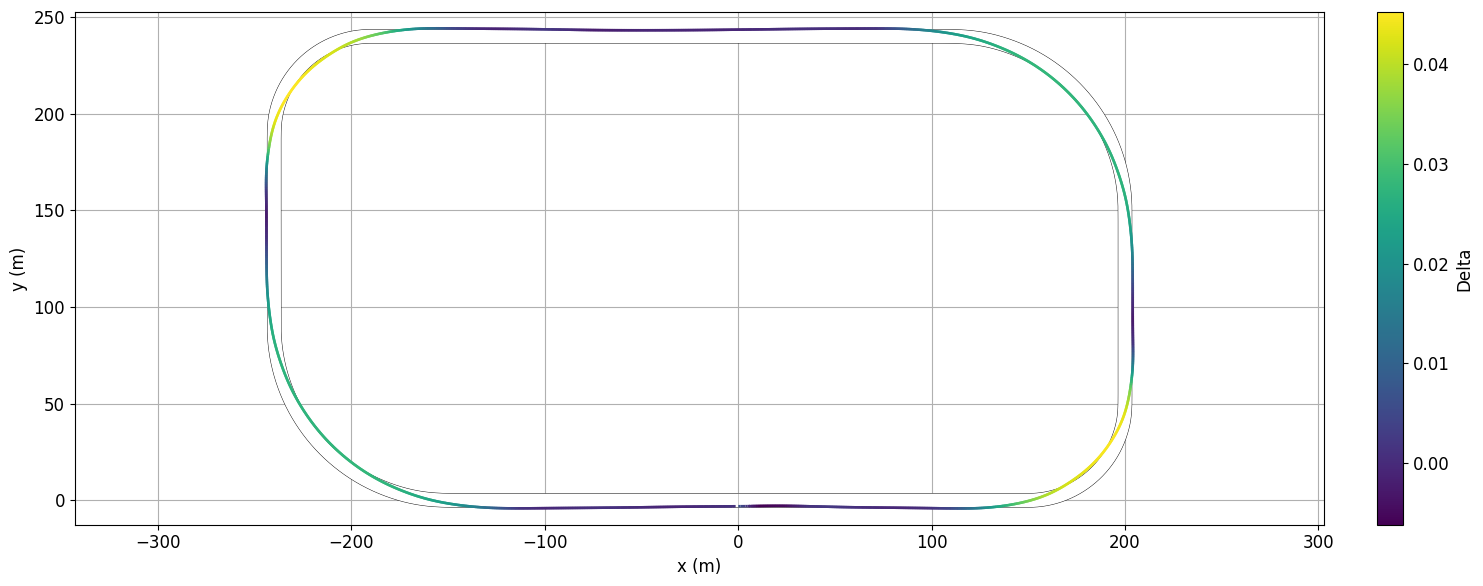
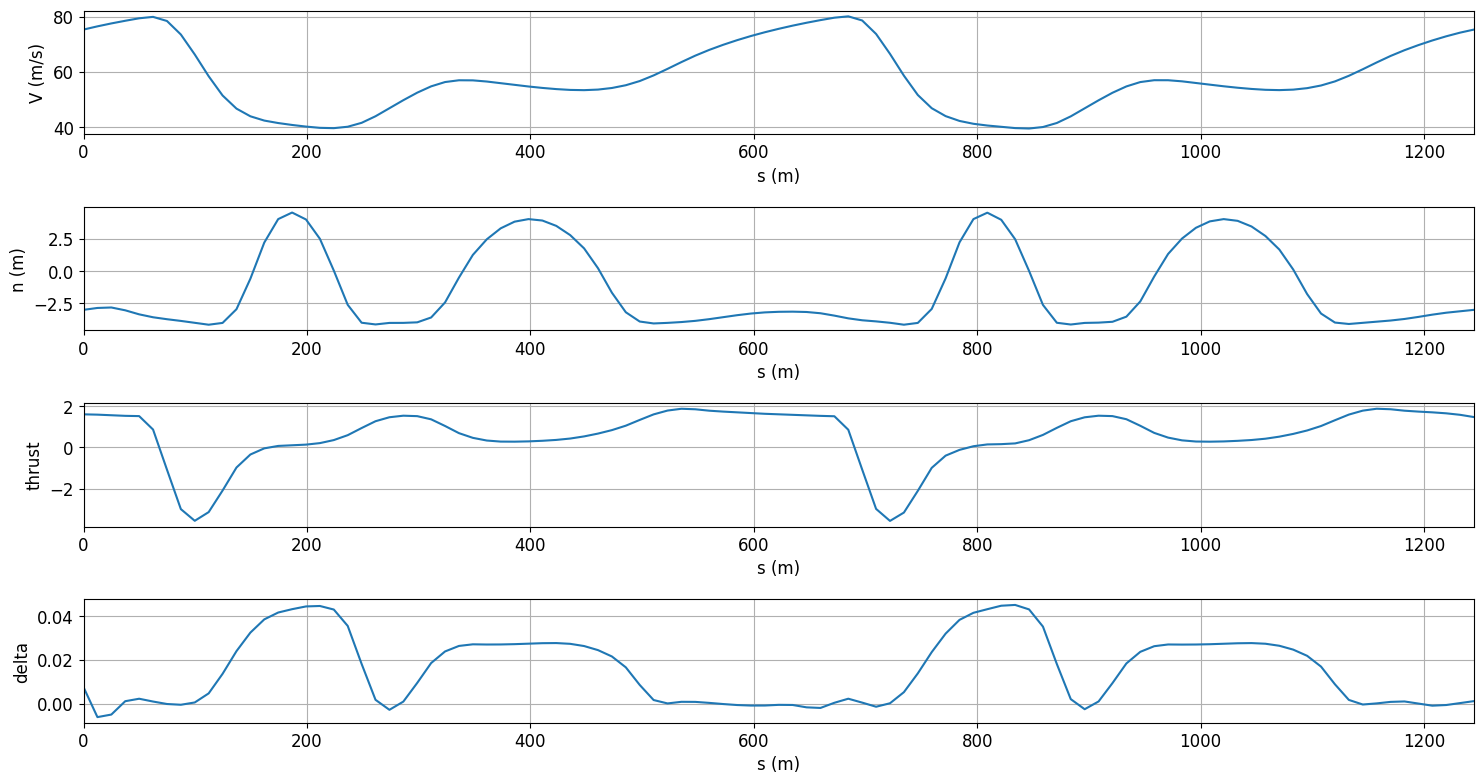
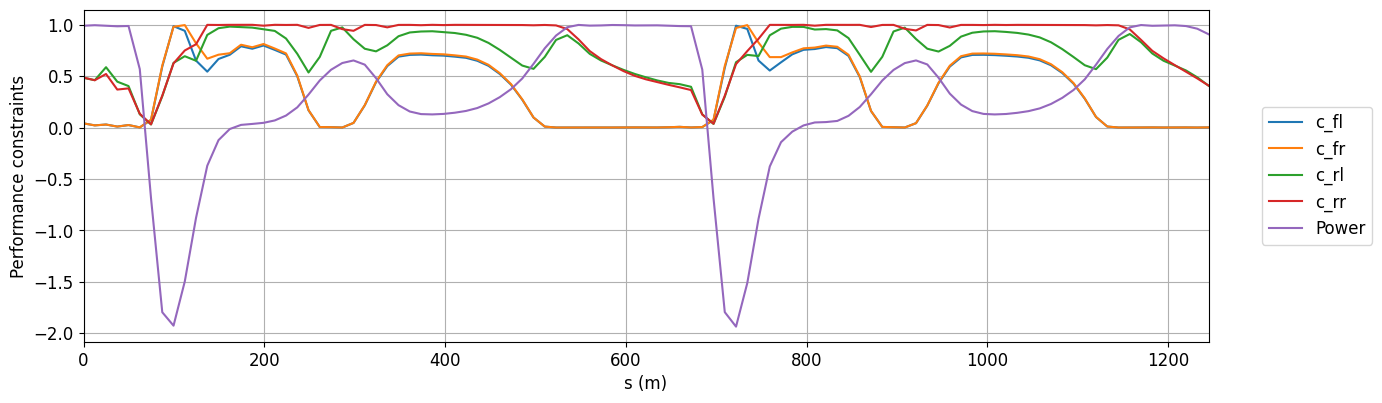
print(s)
[[ 0. ]
[ 12.44822972]
[ 24.89645943]
[ 24.89645943]
[ 37.34468915]
[ 49.79291886]
[ 49.79291886]
[ 62.24114858]
[ 74.68937829]
[ 74.68937829]
[ 87.13760801]
[ 99.58583772]
[ 99.58583772]
[ 112.03406744]
[ 124.48229715]
[ 124.48229715]
[ 136.93052687]
[ 149.37875658]
[ 149.37875658]
[ 161.8269863 ]
[ 174.27521601]
[ 174.27521601]
[ 186.72344573]
[ 199.17167544]
[ 199.17167544]
[ 211.61990516]
[ 224.06813487]
[ 224.06813487]
[ 236.51636459]
[ 248.9645943 ]
[ 248.9645943 ]
[ 261.41282402]
[ 273.86105373]
[ 273.86105373]
[ 286.30928345]
[ 298.75751316]
[ 298.75751316]
[ 311.20574288]
[ 323.65397259]
[ 323.65397259]
[ 336.10220231]
[ 348.55043202]
[ 348.55043202]
[ 360.99866174]
[ 373.44689145]
[ 373.44689145]
[ 385.89512117]
[ 398.34335088]
[ 398.34335088]
[ 410.7915806 ]
[ 423.23981031]
[ 423.23981031]
[ 435.68804003]
[ 448.13626974]
[ 448.13626974]
[ 460.58449946]
[ 473.03272917]
[ 473.03272917]
[ 485.48095889]
[ 497.9291886 ]
[ 497.9291886 ]
[ 510.37741832]
[ 522.82564803]
[ 522.82564803]
[ 535.27387775]
[ 547.72210746]
[ 547.72210746]
[ 560.17033718]
[ 572.61856689]
[ 572.61856689]
[ 585.06679661]
[ 597.51502632]
[ 597.51502632]
[ 609.96325604]
[ 622.41148575]
[ 622.41148575]
[ 634.85971547]
[ 647.30794518]
[ 647.30794518]
[ 659.7561749 ]
[ 672.20440461]
[ 672.20440461]
[ 684.65263433]
[ 697.10086404]
[ 697.10086404]
[ 709.54909376]
[ 721.99732347]
[ 721.99732347]
[ 734.44555319]
[ 746.8937829 ]
[ 746.8937829 ]
[ 759.34201262]
[ 771.79024233]
[ 771.79024233]
[ 784.23847205]
[ 796.68670176]
[ 796.68670176]
[ 809.13493148]
[ 821.58316119]
[ 821.58316119]
[ 834.03139091]
[ 846.47962062]
[ 846.47962062]
[ 858.92785034]
[ 871.37608005]
[ 871.37608005]
[ 883.82430977]
[ 896.27253948]
[ 896.27253948]
[ 908.7207692 ]
[ 921.16899891]
[ 921.16899891]
[ 933.61722863]
[ 946.06545834]
[ 946.06545834]
[ 958.51368806]
[ 970.96191777]
[ 970.96191777]
[ 983.41014749]
[ 995.8583772 ]
[ 995.8583772 ]
[1008.30660692]
[1020.75483663]
[1020.75483663]
[1033.20306635]
[1045.65129606]
[1045.65129606]
[1058.09952578]
[1070.54775549]
[1070.54775549]
[1082.99598521]
[1095.44421492]
[1095.44421492]
[1107.89244464]
[1120.34067435]
[1120.34067435]
[1132.78890407]
[1145.23713378]
[1145.23713378]
[1157.6853635 ]
[1170.13359321]
[1170.13359321]
[1182.58182293]
[1195.03005264]
[1195.03005264]
[1207.47828236]
[1219.92651207]
[1219.92651207]
[1232.37474179]
[1244.8229715 ]]