Water Rocket#
Author: Bernardo Bahia Monteiro (bbahia@umich.edu)
In this example, we will optimize a water rocket for range and height at the apogee, using design variables that are easily modifiable just before launch: the empty mass, the initial water volume and the launch angle. This example builds on multi-phase cannonball ane is adapted from Optimization of a Water Rocket in OpenMDAO/Dymos [BM20].
Nomenclature#
Symbol |
definition |
---|---|
\(v_\text{out}\) |
water exit speed at the nozzle |
\(A_\text{out}\) |
nozzle area |
\(V_w\) |
water volume in the rocket |
\(p\) |
pressure in the rocket |
\(p_a\) |
ambient pressure |
\(\dot{\,}\) |
time derivative |
\(k\) |
polytropic constant |
\(V_b\) |
internal volume of the rocket |
\(\rho_w\) |
water density |
\(T\) |
thrust |
\(q\) |
dynamic pressure |
\(S\) |
cross sectional area |
\((\cdot)_0\) |
value of \((\cdot)\) at \(t=0\) |
\(t\) |
time |
Problem Formulation#
A natural objective function for a water rocket is the maximum height achieved by the rocket during flight, or the horizontal distance it travels, i.e. its range. The design of a water rocket is somewhat constrained by the soda bottle used as its engine. This means that the volume available for water and air is fixed, the initial launch pressure is limited by the bottle’s strength (since the pressure is directly related to the energy available for the rocket, it is easy to see that it should be as high as possible) and the nozzle throat area is also fixed. Given these manufacturing constraints, the design variables we are left with are the empty mass (it can be easily changed through adding ballast), the water volume at the launch, and the launch angle. With this considerations in mind, a natural formulation for the water rocket problem is
Model#
The water rocket model is divided into three basic components: a water engine, responsible for modelling the fluid dynamics inside the rocket and returning its thrust; the aerodynamics, responsible for calculating the atmospheric drag of the rocket; and the equations of motion, responsible for propagating the rocket’s trajectory in time, using Newton’s laws and the forces provided by the other two components.
In order to integrate these three basic components, some additional interfacing components are necessary: an atmospheric model to provide values of ambient pressure for the water engine and air density to the calculation of the dynamic pressure for the aerodynamic model, and a component that calculates the instantaneous mass of the rocket by summing the water mass with the rocket’s empty mass. The high level layout of this model is shown in below.
atmos
, dynamic_pressure
, aero
and eom
are the same models used in multi-phase cannonball.
The remaining components are discussed below.
Warning
The eom
component has a singularity in the flight path angle derivative when the flight speed is zero.
This happens because the rotational dynamics are not modelled.
This can cause convergence problems if the launch velocity is set to zero or the launch angle is set to \(90^\circ\)
Note
Since the range of altitudes achieved by the water rocket is very small (100m), the air density and pressure are practically constant, thus the use of an atmospheric model is not necessary. However, using it makes it easier to reuse code from multi-phase cannonball.
Water engine#
The water engine is modelled by assuming that the air expansion in the rocket follows an adiabatic process and the water flow is incompressible and inviscid, i.e. it follows Bernoulli’s equation. We also make the following simplifying assumptions:
The thrust developed after the water is depleted is negligible
The area inside the bottle is much smaller than the nozzle area
The inertial forces do not affect the fluid dynamics inside the bottle
This simplified modelling can be found in Prusa[@Prusa2000]. A more rigorous formulation, which drops all these simplifying assumptions can be found in Wheeler[@Wheeler2002], Gommes[@Gommes2010], and Barria-Perotti[@BarrioPerotti2010].
The first assumption leads to an underestimation of the rocket performance, since the air left in the bottle after it is out of water is known to generate appreciable thrust[@Thorncroft2009]. This simplified model, however, produces physically meaningful results.
There are two states in this dynamical model, the water volume in the rocket \(V_w\) and the gauge pressure inside the rocket \(p\). The constitutive equations and the N2 diagram showing the model organization are shown below.
Constitutive equations of the water engine model#
Component |
Equation |
---|---|
water_exhaust_speed |
\(v_\text{out} = \sqrt{2(p-p_a)/\rho_w}\) |
water_flow_rate |
\(\dot{V}_w = -v_\text{out} A_\text{out}\) |
pressure_rate |
\(\dot{p} = kp\frac{\dot{V_w}}{(V_b-V_w)}\) |
water_thrust |
\(T = (\rho_w v_\text{out})(v_\text{out}A_\text{out})\) |
import numpy as np
import matplotlib as mpl
import matplotlib.pyplot as plt
import openmdao.api as om
import dymos as dm
class WaterEngine(om.Group):
"""
Computes thrust and water flow for a water.
Simplifications:
- the pressure due to the water column in the non inertial frame (i.e.
under a+g acceleration) is insignificant compared to the air pressure
- the water does not have appreciable speed inside the bottle
"""
def initialize(self):
self.options.declare('num_nodes', types=int)
def setup(self):
nn = self.options['num_nodes']
self.add_subsystem(name='water_exhaust_speed',
subsys=_WaterExhaustSpeed(num_nodes=nn),
promotes=['p', 'p_a', 'rho_w'])
self.add_subsystem(name='water_flow_rate',
subsys=_WaterFlowRate(num_nodes=nn),
promotes=['A_out', 'Vdot'])
self.add_subsystem(name='pressure_rate',
subsys=_PressureRate(num_nodes=nn),
promotes=['p', 'k', 'V_b', 'V_w', 'Vdot', 'pdot'])
self.add_subsystem(name='water_thrust',
subsys=_WaterThrust(num_nodes=nn),
promotes=['rho_w', 'A_out', 'F'])
self.set_input_defaults('A_out', val=np.pi*13e-3**2/4*np.ones(nn))
self.set_input_defaults('p', val=6.5e5*np.ones(nn))
self.connect('water_exhaust_speed.v_out', 'water_flow_rate.v_out')
self.connect('water_exhaust_speed.v_out', 'water_thrust.v_out')
class _WaterExhaustSpeed(om.ExplicitComponent):
def initialize(self):
self.options.declare('num_nodes', types=int)
def setup(self):
nn = self.options['num_nodes']
self.add_input(name='rho_w', val=1e3*np.ones(nn), desc='water density', units='kg/m**3')
self.add_input(name='p', val=6.5e5*np.ones(nn), desc='air pressure', units='N/m**2') # 5.5bar = 80 psi
self.add_input(name='p_a', val=1.01e5*np.ones(nn), desc='air pressure', units='N/m**2')
self.add_output(name='v_out', shape=(nn,), desc='water exhaust speed', units='m/s')
ar = np.arange(nn)
self.declare_partials(of='*', wrt='*', rows=ar, cols=ar)
def compute(self, inputs, outputs):
p = inputs['p']
p_a = inputs['p_a']
rho_w = inputs['rho_w']
outputs['v_out'] = np.sqrt(2*(p-p_a)/rho_w)
def compute_partials(self, inputs, partials):
p = inputs['p']
p_a = inputs['p_a']
rho_w = inputs['rho_w']
v_out = np.sqrt(2*(p-p_a)/rho_w)
partials['v_out', 'p'] = 1/v_out/rho_w
partials['v_out', 'p_a'] = -1/v_out/rho_w
partials['v_out', 'rho_w'] = 1/v_out*(-(p-p_a)/rho_w**2)
class _PressureRate(om.ExplicitComponent):
def initialize(self):
self.options.declare('num_nodes', types=int)
def setup(self):
nn = self.options['num_nodes']
self.add_input(name='p', val=np.ones(nn), desc='air pressure', units='N/m**2')
self.add_input(name='k', val=1.4*np.ones(nn), desc='polytropic coefficient for expansion', units=None)
self.add_input(name='V_b', val=2e-3*np.ones(nn), desc='bottle volume', units='m**3')
self.add_input(name='V_w', val=1e-3*np.ones(nn), desc='water volume', units='m**3')
self.add_input(name='Vdot', shape=(nn,), desc='water flow', units='m**3/s')
self.add_output(name='pdot', shape=(nn,), desc='pressure derivative', units='N/m**2/s')
ar = np.arange(nn)
self.declare_partials(of='*', wrt='*', rows=ar, cols=ar)
def compute(self, inputs, outputs):
p = inputs['p']
k = inputs['k']
V_b = inputs['V_b']
V_w = inputs['V_w']
Vdot = inputs['Vdot']
pdot = p*k*Vdot/(V_b-V_w)
outputs['pdot'] = pdot
def compute_partials(self, inputs, partials):
p = inputs['p']
k = inputs['k']
V_b = inputs['V_b']
V_w = inputs['V_w']
Vdot = inputs['Vdot']
partials['pdot', 'p'] = k*Vdot/(V_b-V_w)
partials['pdot', 'k'] = p*Vdot/(V_b-V_w)
partials['pdot', 'V_b'] = -p*k*Vdot/(V_b-V_w)**2
partials['pdot', 'V_w'] = p*k*Vdot/(V_b-V_w)**2
partials['pdot', 'Vdot'] = p*k/(V_b-V_w)
class _WaterFlowRate(om.ExplicitComponent):
""" Computer water flow rate"""
def initialize(self):
self.options.declare('num_nodes', types=int)
def setup(self):
nn = self.options['num_nodes']
self.add_input(name='A_out', val=np.ones(nn), desc='nozzle outlet area', units='m**2')
self.add_input(name='v_out', val=np.zeros(nn), desc='water exhaust speed', units='m/s')
self.add_output(name='Vdot', shape=(nn,), desc='water flow', units='m**3/s')
ar = np.arange(nn)
self.declare_partials(of='*', wrt='*', rows=ar, cols=ar)
def compute(self, inputs, outputs):
A_out = inputs['A_out']
v_out = inputs['v_out']
outputs['Vdot'] = -v_out*A_out
def compute_partials(self, inputs, partials):
A_out = inputs['A_out']
v_out = inputs['v_out']
partials['Vdot', 'A_out'] = -v_out
partials['Vdot', 'v_out'] = -A_out
The _MassAdder
component calculates the rocket’s instantaneous mass by
summing the water mass with the rockets empty mass, i.e.
class _MassAdder(om.ExplicitComponent):
def initialize(self):
self.options.declare('num_nodes', types=int)
def setup(self):
nn = self.options['num_nodes']
self.add_input('m_empty', val=np.zeros(nn), desc='empty mass', units='kg')
self.add_input('V_w', val=1e-3*np.ones(nn), desc='water volume', units='m**3')
self.add_input('rho_w', val=1e3*np.ones(nn), desc="water density", units='kg/m**3')
self.add_output('m', val=np.zeros(nn), desc='total mass', units='kg')
ar = np.arange(nn)
self.declare_partials('*', '*', cols=ar, rows=ar)
def compute(self, inputs, outputs):
outputs['m'] = inputs['m_empty'] + inputs['rho_w']*inputs['V_w']
def compute_partials(self, inputs, jacobian):
jacobian['m', 'm_empty'] = 1
jacobian['m', 'rho_w'] = inputs['V_w']
jacobian['m', 'V_w'] = inputs['rho_w']
Now these components are joined in a single group
display_source('dymos.examples.water_rocket.water_propulsion_ode.WaterPropulsionODE')
class WaterPropulsionODE(om.Group):
def initialize(self):
self.options.declare('num_nodes', types=int)
self.options.declare('ballistic', types=bool, default=False,
desc='If True, neglect propulsion system.')
def setup(self):
nn = self.options['num_nodes']
self.add_subsystem(name='atmos',
subsys=USatm1976Comp(num_nodes=nn))
if not self.options['ballistic']:
self.add_subsystem(name='water_engine',
subsys=WaterEngine(num_nodes=nn),
promotes_inputs=['rho_w'])
self.add_subsystem(name='mass_adder',
subsys=_MassAdder(num_nodes=nn),
promotes_inputs=['rho_w'])
self.add_subsystem(name='dynamic_pressure',
subsys=DynamicPressureComp(num_nodes=nn))
self.add_subsystem(name='aero',
subsys=LiftDragForceComp(num_nodes=nn))
self.add_subsystem(name='eom',
subsys=FlightPathEOM2D(num_nodes=nn))
self.connect('atmos.rho', 'dynamic_pressure.rho')
self.connect('dynamic_pressure.q', 'aero.q')
self.connect('aero.f_drag', 'eom.D')
self.connect('aero.f_lift', 'eom.L')
if not self.options['ballistic']:
self.connect('atmos.pres', 'water_engine.p_a')
self.connect('water_engine.F', 'eom.T')
self.connect('mass_adder.m', 'eom.m')
Phases#
The flight of the water rocket is split in three distinct phases: propelled ascent, ballistic ascent and ballistic descent. If the simplification of no thrust without water were lifted, there would be an extra “air propelled ascent” phase between the propelled ascent and ballistic ascent phases.
Propelled ascent: is the flight phase where the rocket still has water inside, and hence it is producing thrust. The thrust is given by the water engine model, and fed into the flight dynamic equations. It starts at launch and finishes when the water is depleted, i.e. \(V_w=0\).
Ballistic ascent: is the flight phase where the rocket is ascending (\(\gamma>0\)) but produces no thrust. This phase begins at the end of thepropelled ascent phase and ends at the apogee, defined by \(\gamma=0\).
Descent: is the phase where the rocket is descending without thrust. It begins at the end of the ballistic ascent phase and ends with ground impact, i.e. \(h=0\).
def new_propelled_ascent_phase(transcription):
propelled_ascent = dm.Phase(ode_class=WaterPropulsionODE,
transcription=transcription)
# All initial states except flight path angle and water volume are fixed
# Final flight path angle is fixed (we will set it to zero so that the phase ends at apogee)
# Final water volume is fixed (we will set it to zero so that phase ends when bottle empties)
propelled_ascent.set_time_options(
fix_initial=True, duration_bounds=(0.001, 0.5), duration_ref=0.1, units='s')
propelled_ascent.add_state('r', units='m', rate_source='eom.r_dot',
fix_initial=True, fix_final=False, ref=100.0, defect_ref=100.0)
propelled_ascent.add_state('h', units='m', rate_source='eom.h_dot', targets=['atmos.h'],
fix_initial=True, fix_final=False, ref=10.0, defect_ref=10.0)
propelled_ascent.add_state('gam', units='deg', rate_source='eom.gam_dot', targets=['eom.gam'],
fix_initial=False, fix_final=False, lower=0, upper=85.0, ref=90)
propelled_ascent.add_state('v', units='m/s', rate_source='eom.v_dot', targets=['dynamic_pressure.v', 'eom.v'],
fix_initial=True, fix_final=False, ref=100, defect_ref=100, lower=0.01)
propelled_ascent.add_state('p', units='bar', rate_source='water_engine.pdot',
targets=['water_engine.p'], fix_initial=True, fix_final=False,
lower=1.02)
propelled_ascent.add_state('V_w', units='L', rate_source='water_engine.Vdot',
targets=['water_engine.V_w', 'mass_adder.V_w'],
fix_initial=False, fix_final=True, ref=10., defect_ref=0.1,
lower=0)
propelled_ascent.add_parameter(
'S', targets=['aero.S'], units='m**2')
propelled_ascent.add_parameter(
'm_empty', targets=['mass_adder.m_empty'], units='kg')
propelled_ascent.add_parameter(
'V_b', targets=['water_engine.V_b'], units='m**3')
propelled_ascent.add_timeseries_output('water_engine.F', 'T', units='N')
return propelled_ascent
def new_ballistic_ascent_phase(transcription):
ballistic_ascent = dm.Phase(ode_class=WaterPropulsionODE, transcription=transcription,
ode_init_kwargs={'ballistic': True})
# All initial states are free (they will be linked to the final stages of propelled_ascent).
# Final flight path angle is fixed (we will set it to zero so that the phase ends at apogee)
ballistic_ascent.set_time_options(
fix_initial=False, initial_bounds=(0.001, 1), duration_bounds=(0.001, 10),
duration_ref=1, units='s')
ballistic_ascent.add_state('r', units='m', rate_source='eom.r_dot', fix_initial=False,
fix_final=False, ref=1.0, defect_ref=1.0)
ballistic_ascent.add_state('h', units='m', rate_source='eom.h_dot', targets=['atmos.h'],
fix_initial=False, fix_final=False, ref=1.0)
ballistic_ascent.add_state('gam', units='deg', rate_source='eom.gam_dot', targets=['eom.gam'],
fix_initial=False, fix_final=True, upper=89, ref=90)
ballistic_ascent.add_state('v', units='m/s', rate_source='eom.v_dot',
targets=['dynamic_pressure.v', 'eom.v'], fix_initial=False,
fix_final=False, ref=1.)
ballistic_ascent.add_parameter('S', targets=['aero.S'], units='m**2')
ballistic_ascent.add_parameter('m_empty', targets=['eom.m'], units='kg')
return ballistic_ascent
def new_descent_phase(transcription):
descent = dm.Phase(ode_class=WaterPropulsionODE, transcription=transcription,
ode_init_kwargs={'ballistic': True})
# All initial states and time are free (they will be linked to the final states of ballistic_ascent).
# Final altitude is fixed (we will set it to zero so that the phase ends at ground impact)
descent.set_time_options(initial_bounds=(.5, 100), duration_bounds=(.5, 100),
duration_ref=10, units='s')
descent.add_state('r', units='m', rate_source='eom.r_dot', fix_initial=False, fix_final=False)
descent.add_state('h', units='m', rate_source='eom.h_dot', targets=['atmos.h'],
fix_initial=False, fix_final=True)
descent.add_state('gam', units='deg', rate_source='eom.gam_dot', targets=['eom.gam'],
fix_initial=False, fix_final=False)
descent.add_state('v', units='m/s', rate_source='eom.v_dot',
targets=['dynamic_pressure.v', 'eom.v'],
fix_initial=False, fix_final=False,
ref=1)
descent.add_parameter('S', targets=['aero.S'], units='m**2')
descent.add_parameter('mass', targets=['eom.m'], units='kg')
return descent
Model parameters#
The model requires a few constant parameters. The values used are shown in the following table.
Values for parameters in the water rocket model
Parameter |
Value |
Unit |
Reference |
---|---|---|---|
\(C_D\) |
0.3450 |
- |
|
\(S\) |
\(\pi 106^2/4\) |
\(mm^2\) |
|
\(k\) |
1.2 |
- |
|
\(A_\text{out}\) |
\(\pi22^2/4\) |
\(mm^2\) |
[air13] |
\(V_b\) |
2 |
L |
|
\(\rho_w\) |
1000 |
\(kg/m^3\) |
|
\(p_0\) |
6.5 |
bar |
|
\(v_0\) |
0.1 |
\(m/s\) |
|
\(h_0\) |
0 |
\(m\) |
|
\(r_0\) |
0 |
\(m\) |
Values for the bottle volume \(V_b\), its cross-sectional area \(S\) and the nozzle area \(A_\text{out}\) are determined by the soda bottle that makes the rocket primary structure, and thus are not easily modifiable by the designer. The polytropic coefficient \(k\) is a function of the moist air characteristics inside the rocket. The initial speed \(v_0\) must be set to a value higher than zero, otherwise the flight dynamic equations become singular. This issue arises from the angular dynamics of the rocket not being modelled. The drag coefficient \(C_D\) is sensitive to the aerodynamic design, but can be optimized by a single discipline analysis. The initial pressure \(p_0\) should be maximized in order to obtain the maximum range or height for the rocket. It is limited by the structural properties of the bottle, which are modifiable by the designer, since the bottle needs to be available commercially. Finally, the starting point of the rocket is set to the origin.
Putting it all together#
The different phases must be combined in a single trajectory, and linked in a sequence. Here we also define the design variables.
display_source('dymos.examples.water_rocket.phases.new_water_rocket_trajectory')
def new_water_rocket_trajectory(objective):
tx_prop = dm.Radau(num_segments=20, order=3, compressed=True)
tx_bal = dm.Radau(num_segments=10, order=3, compressed=True)
tx_desc = dm.Radau(num_segments=10, order=3, compressed=True)
traj = dm.Trajectory()
# Add phases to trajectory
propelled_ascent = traj.add_phase('propelled_ascent', new_propelled_ascent_phase(tx_prop))
ballistic_ascent = traj.add_phase('ballistic_ascent', new_ballistic_ascent_phase(tx_bal))
descent = traj.add_phase('descent', new_descent_phase(tx_desc))
# Link phases
traj.link_phases(phases=['propelled_ascent', 'ballistic_ascent'], vars=['*'])
traj.link_phases(phases=['ballistic_ascent', 'descent'], vars=['*'])
# Set objective function
if objective == 'height':
ballistic_ascent.add_objective('h', loc='final', ref=-1.0)
elif objective == 'range':
descent.add_objective('r', loc='final', ref=-1.0)
else:
raise ValueError(f"objective='{objective}' is not defined. Try using 'height' or 'range'")
# Add design parameters to the trajectory.
traj.add_parameter('CD',
targets={'propelled_ascent': ['aero.CD'],
'ballistic_ascent': ['aero.CD'],
'descent': ['aero.CD']},
val=0.3450, units=None, opt=False)
traj.add_parameter('CL',
targets={'propelled_ascent': ['aero.CL'],
'ballistic_ascent': ['aero.CL'],
'descent': ['aero.CL']},
val=0.0, units=None, opt=False)
traj.add_parameter('T',
targets={'ballistic_ascent': ['eom.T'],
'descent': ['eom.T']},
val=0.0, units='N', opt=False)
traj.add_parameter('alpha',
targets={'propelled_ascent': ['eom.alpha'],
'ballistic_ascent': ['eom.alpha'],
'descent': ['eom.alpha']},
val=0.0, units='deg', opt=False)
traj.add_parameter('m_empty', units='kg', val=0.15,
targets={'propelled_ascent': 'm_empty',
'ballistic_ascent': 'm_empty',
'descent': 'mass'},
lower=0.001, upper=1, ref=0.1,
opt=False)
traj.add_parameter('V_b', units='m**3', val=2e-3,
targets={'propelled_ascent': 'V_b'},
opt=False)
traj.add_parameter('S', units='m**2', val=np.pi*106e-3**2/4, opt=False)
traj.add_parameter('A_out', units='m**2', val=np.pi*22e-3**2/4.,
targets={'propelled_ascent': ['water_engine.A_out']},
opt=False)
traj.add_parameter('k', units=None, val=1.2, opt=False,
targets={'propelled_ascent': ['water_engine.k']})
# Make parameter m_empty a design variable
traj.set_parameter_options('m_empty', opt=True)
return traj, {'propelled_ascent': propelled_ascent,
'ballistic_ascent': ballistic_ascent,
'descent': descent}
Helper Functions to Access the Results#
from collections import namedtuple
def summarize_results(water_rocket_problem):
p = water_rocket_problem
Entry = namedtuple('Entry', 'value unit')
summary = {
'Launch angle': Entry(p.get_val('traj.propelled_ascent.timeseries.gam', units='deg')[0, 0], 'deg'),
'Flight angle at end of propulsion': Entry(p.get_val('traj.propelled_ascent.timeseries.gam',
units='deg')[-1, 0], 'deg'),
'Empty mass': Entry(p.get_val('traj.parameters:m_empty', units='kg')[0], 'kg'),
'Water volume': Entry(p.get_val('traj.propelled_ascent.timeseries.V_w', 'L')[0, 0], 'L'),
'Maximum range': Entry(p.get_val('traj.descent.timeseries.r', units='m')[-1, 0], 'm'),
'Maximum height': Entry(p.get_val('traj.ballistic_ascent.timeseries.h', units='m')[-1, 0], 'm'),
'Maximum velocity': Entry(p.get_val('traj.propelled_ascent.timeseries.v', units='m/s')[-1, 0], 'm/s'),
}
return summary
colors={'pa': 'tab:blue', 'ba': 'tab:orange', 'd': 'tab:green'}
def plot_propelled_ascent(p, exp_out):
fig, ax = plt.subplots(2, 2, sharex=True, figsize=(12, 6))
t_imp = p.get_val('traj.propelled_ascent.timeseries.time', 's')
t_exp = exp_out.get_val('traj.propelled_ascent.timeseries.time', 's')
c = colors['pa']
ax[0,0].plot(t_imp, p.get_val('traj.propelled_ascent.timeseries.p', 'bar'), '.', color=c)
ax[0,0].plot(t_exp, exp_out.get_val('traj.propelled_ascent.timeseries.p', 'bar'), '-', color=c)
ax[0,0].set_ylabel('p (bar)')
ax[0,0].set_ylim(bottom=0)
ax[1,0].plot(t_imp, p.get_val('traj.propelled_ascent.timeseries.V_w', 'L'), '.', color=c)
ax[1,0].plot(t_exp, exp_out.get_val('traj.propelled_ascent.timeseries.V_w', 'L'), '-', color=c)
ax[1,0].set_ylabel('$V_w$ (L)')
ax[0,1].plot(t_imp, p.get_val('traj.propelled_ascent.timeseries.T', 'N'), '.', color=c)
ax[0,1].plot(t_exp, exp_out.get_val('traj.propelled_ascent.timeseries.T', 'N'), '-', color=c)
ax[0,1].set_ylabel('T (N)')
ax[0,1].set_ylim(bottom=0)
ax[1,1].plot(t_imp, p.get_val('traj.propelled_ascent.timeseries.v', 'm/s'), '.', color=c)
ax[1,1].plot(t_exp, exp_out.get_val('traj.propelled_ascent.timeseries.v', 'm/s'), '-', color=c)
ax[1,1].set_ylabel('v (m/s)')
ax[1,1].set_ylim(bottom=0)
ax[1,0].set_xlabel('t (s)')
ax[1,1].set_xlabel('t (s)')
for i in range(4):
ax.ravel()[i].grid(True, alpha=0.2)
fig.tight_layout()
def plot_states(p, exp_out, legend_loc='right', legend_ncol=3):
fig, axes = plt.subplots(nrows=2, ncols=2, figsize=(12, 4), sharex=True)
states = ['r', 'h', 'v', 'gam']
units = ['m', 'm', 'm/s', 'deg']
phases = ['propelled_ascent', 'ballistic_ascent', 'descent']
time_imp = {'ballistic_ascent': p.get_val('traj.ballistic_ascent.timeseries.time'),
'propelled_ascent': p.get_val('traj.propelled_ascent.timeseries.time'),
'descent': p.get_val('traj.descent.timeseries.time')}
time_exp = {'ballistic_ascent': exp_out.get_val('traj.ballistic_ascent.timeseries.time'),
'propelled_ascent': exp_out.get_val('traj.propelled_ascent.timeseries.time'),
'descent': exp_out.get_val('traj.descent.timeseries.time')}
x_imp = {phase: {state: p.get_val(f"traj.{phase}.timeseries.{state}", unit)
for state, unit in zip(states, units)
}
for phase in phases
}
x_exp = {phase: {state: exp_out.get_val(f"traj.{phase}.timeseries.{state}", unit)
for state, unit in zip(states, units)
}
for phase in phases
}
for i, (state, unit) in enumerate(zip(states, units)):
axes.ravel()[i].set_ylabel(f"{state} ({unit})" if state != 'gam' else f'$\gamma$ ({unit})')
axes.ravel()[i].plot(time_imp['propelled_ascent'], x_imp['propelled_ascent'][state], '.', color=colors['pa'])
axes.ravel()[i].plot(time_imp['ballistic_ascent'], x_imp['ballistic_ascent'][state], '.', color=colors['ba'])
axes.ravel()[i].plot(time_imp['descent'], x_imp['descent'][state], '.', color=colors['d'])
h1, = axes.ravel()[i].plot(time_exp['propelled_ascent'], x_exp['propelled_ascent'][state], '-', color=colors['pa'], label='Propelled Ascent')
h2, = axes.ravel()[i].plot(time_exp['ballistic_ascent'], x_exp['ballistic_ascent'][state], '-', color=colors['ba'], label='Ballistic Ascent')
h3, = axes.ravel()[i].plot(time_exp['descent'], x_exp['descent'][state], '-', color=colors['d'], label='Descent')
if state == 'gam':
axes.ravel()[i].yaxis.set_major_locator(mpl.ticker.MaxNLocator(nbins='auto', steps=[1, 1.5, 3, 4.5, 6, 9, 10]))
axes.ravel()[i].set_yticks(np.arange(-90, 91, 45))
axes.ravel()[i].grid(True, alpha=0.2)
axes[1, 0].set_xlabel('t (s)')
axes[1, 1].set_xlabel('t (s)')
plt.figlegend(handles=[h1, h2, h3], loc=legend_loc, ncol=legend_ncol)
fig.tight_layout()
def plot_trajectory(p, exp_out, legend_loc='center right'):
fig, axes = plt.subplots(nrows=1, ncols=1, figsize=(8, 6))
r_imp = {'ballistic_ascent': p.get_val('traj.ballistic_ascent.timeseries.r'),
'propelled_ascent': p.get_val('traj.propelled_ascent.timeseries.r'),
'descent': p.get_val('traj.descent.timeseries.r')}
r_exp = {'ballistic_ascent': exp_out.get_val('traj.ballistic_ascent.timeseries.r'),
'propelled_ascent': exp_out.get_val('traj.propelled_ascent.timeseries.r'),
'descent': exp_out.get_val('traj.descent.timeseries.r')}
h_imp = {'ballistic_ascent': p.get_val('traj.ballistic_ascent.timeseries.h'),
'propelled_ascent': p.get_val('traj.propelled_ascent.timeseries.h'),
'descent': p.get_val('traj.descent.timeseries.h')}
h_exp = {'ballistic_ascent': exp_out.get_val('traj.ballistic_ascent.timeseries.h'),
'propelled_ascent': exp_out.get_val('traj.propelled_ascent.timeseries.h'),
'descent': exp_out.get_val('traj.descent.timeseries.h')}
axes.plot(r_imp['propelled_ascent'], h_imp['propelled_ascent'], 'o', color=colors['pa'])
axes.plot(r_imp['ballistic_ascent'], h_imp['ballistic_ascent'], 'o', color=colors['ba'])
axes.plot(r_imp['descent'], h_imp['descent'], 'o', color=colors['d'])
h1, = axes.plot(r_exp['propelled_ascent'], h_exp['propelled_ascent'], '-', color=colors['pa'], label='Propelled Ascent')
h2, = axes.plot(r_exp['ballistic_ascent'], h_exp['ballistic_ascent'], '-', color=colors['ba'], label='Ballistic Ascent')
h3, = axes.plot(r_exp['descent'], h_exp['descent'], '-', color=colors['d'], label='Descent')
axes.set_xlabel('r (m)')
axes.set_ylabel('h (m)')
axes.set_aspect('equal', 'box')
plt.figlegend(handles=[h1, h2, h3], loc=legend_loc)
axes.grid(alpha=0.2)
fig.tight_layout()
Optimizing for Height#
from dymos.examples.water_rocket.phases import new_water_rocket_trajectory, set_sane_initial_guesses
p = om.Problem(model=om.Group())
traj, phases = new_water_rocket_trajectory(objective='height')
traj = p.model.add_subsystem('traj', traj)
p.driver = om.pyOptSparseDriver(optimizer='IPOPT', print_results=False)
p.driver.opt_settings['print_level'] = 5
p.driver.opt_settings['max_iter'] = 1000
p.driver.opt_settings['mu_strategy'] = 'adaptive'
p.driver.opt_settings['nlp_scaling_method'] = 'gradient-based' # for faster convergence
p.driver.opt_settings['alpha_for_y'] = 'safer-min-dual-infeas'
p.driver.declare_coloring(tol=1.0E-12)
# Finish Problem Setup
p.model.linear_solver = om.DirectSolver()
p.setup()
set_sane_initial_guesses(phases)
dm.run_problem(p, run_driver=True, simulate=True)
summary = summarize_results(p)
for key, entry in summary.items():
print(f'{key}: {entry.value:6.4f} {entry.unit}')
sol_out = om.CaseReader(p.get_outputs_dir() / 'dymos_solution.db').get_case('final')
sim_out = om.CaseReader(traj.sim_prob.get_outputs_dir() / 'dymos_simulation.db').get_case('final')
'rhs_checking' is disabled for 'DirectSolver in <model> <class Group>' but that solver has redundant adjoint solves. If it is expensive to compute derivatives for this solver, turning on 'rhs_checking' may improve performance.
Jacobian shape: (611, 613) (1.23% nonzero)
FWD solves: 20 REV solves: 0
Total colors vs. total size: 20 vs 613 (96.74% improvement)
Sparsity computed using tolerance: 1e-12.
Dense total jacobian for Problem 'problem' was computed 3 times.
Time to compute sparsity: 0.5285 sec
Time to compute coloring: 0.8072 sec
Memory to compute coloring: -2.3438 MB
Coloring created on: 2025-04-10 15:45:51
List of user-set options:
Name Value used
alpha_for_y = safer-min-dual-infeas yes
file_print_level = 5 yes
hessian_approximation = limited-memory yes
linear_solver = mumps yes
max_iter = 1000 yes
mu_strategy = adaptive yes
nlp_scaling_method = gradient-based yes
output_file = /home/runner/work/dymos/dymos/docs/dymos_book/examples/water_rocket/problem_out/IPOPT.out yes
print_level = 5 yes
print_user_options = yes yes
sb = yes yes
This is Ipopt version 3.14.12, running with linear solver MUMPS 5.2.1.
Number of nonzeros in equality constraint Jacobian...: 103007
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 613
variables with only lower bounds: 180
variables with lower and upper bounds: 67
variables with only upper bounds: 30
Total number of equality constraints.................: 610
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 -1.0000000e+02 9.00e+01 4.22e-01 0.0 0.00e+00 - 0.00e+00 0.00e+00 0
1 -7.0820124e+01 2.49e+01 4.15e+03 -5.8 4.98e+01 - 1.84e-01 7.23e-01h 1
2 -4.4787630e+01 8.88e+00 6.71e+04 0.6 4.13e+01 - 1.00e+00 9.82e-01h 1
3 -4.9302111e+01 7.38e+01 2.80e+06 1.6 6.63e+01 - 1.00e+00 3.21e-01f 1
4 -3.9326926e+01 4.90e+01 3.99e+05 1.9 3.77e+01 - 3.43e-01 1.00e+00h 1
5 -3.2035130e+01 3.01e+01 9.92e+04 1.9 1.77e+01 - 3.95e-01 1.00e+00f 1
6 -2.9180468e+01 2.35e+01 1.84e+04 1.9 1.71e+01 - 9.02e-01 1.00e+00f 1
7 -2.7622916e+01 3.67e+00 2.00e+04 1.9 3.93e+01 - 3.16e-01 1.00e+00f 1
8 -2.7696393e+01 2.36e+01 1.23e+04 1.4 4.58e+01 - 9.99e-01 1.00e+00h 1
9 -2.7003630e+01 1.31e+00 1.97e+03 0.4 1.00e+01 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
10 -2.7332373e+01 1.07e-01 3.06e+02 -0.6 1.88e+00 - 1.00e+00 1.00e+00h 1
11 -3.0084122e+01 2.12e-02 2.34e+02 -1.7 2.75e+00 - 1.00e+00 1.00e+00f 1
12 -3.3530125e+01 3.18e-02 2.73e+02 -2.7 3.45e+00 - 1.00e+00 1.00e+00f 1
13 -3.7138105e+01 5.50e-02 2.99e+02 -3.7 3.61e+00 - 1.00e+00 1.00e+00f 1
14 -3.7191722e+01 4.35e-03 4.19e+00 -4.9 1.38e-01 - 1.00e+00 1.00e+00h 1
15 -3.7653940e+01 6.04e-03 1.38e+00 -6.4 6.84e-01 - 1.00e+00 1.00e+00f 1
16 -6.5062744e+01 2.89e+00 7.22e+00 -6.0 1.08e+02 - 1.00e+00 2.53e-01f 1
17 -3.0667545e+01 1.19e+00 2.15e+01 -5.6 2.34e+01 - 4.59e-01 1.00e+00H 1
18 -5.4939845e+01 1.74e+00 1.51e+01 -5.9 5.46e+01 - 4.90e-01 4.45e-01f 1
19 -3.6682013e+00 8.75e-01 1.58e+01 -5.9 6.18e+01 - 6.49e-02 9.16e-01H 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
20 -1.9149207e+01 1.24e+00 1.61e+01 -5.9 4.23e+01 - 1.00e+00 4.67e-01f 1
21 -2.0323233e+01 8.97e-01 2.45e+01 -5.9 1.27e+01 - 2.14e-06 2.77e-01h 2
22 -2.1420905e+01 5.46e-01 4.15e+02 -5.9 5.12e+00 - 3.59e-01 3.92e-01h 2
23 -2.2677185e+01 3.85e-01 8.27e+02 -5.9 3.68e+00 - 6.63e-01 1.00e+00h 1
24 -2.2735365e+01 5.53e-02 1.37e+01 -5.9 3.10e-01 - 1.00e+00 1.00e+00h 1
25 -2.2756534e+01 3.18e-03 8.61e-02 -5.9 5.70e-02 - 9.58e-01 1.00e+00h 1
26 -2.4981336e+01 8.75e-02 3.07e+00 -6.4 2.22e+00 - 1.00e+00 1.00e+00f 1
27 -4.3309621e+01 1.41e+00 1.58e+01 -6.5 5.42e+03 - 1.92e-03 3.38e-03f 1
28 -7.9044007e+01 1.89e+02 1.69e+03 -5.5 2.28e+02 - 1.00e+00 1.57e-01f 1
29 -1.5126320e+02 1.52e+02 1.03e+03 -3.8 7.22e+01 - 2.92e-02 1.00e+00f 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
30 -1.3521832e+02 7.58e+01 8.16e+02 -2.9 5.90e+01 - 1.00e+00 6.68e-01h 1
31 -5.7371497e+01 1.93e+01 2.30e+02 -1.6 7.78e+01 - 1.00e+00 1.00e+00h 1
32 -5.7732631e+01 1.34e+01 2.13e+02 -1.8 1.12e+02 - 1.99e-01 3.20e-01h 1
33 -3.3210650e+01 6.50e+01 2.39e+02 -1.8 4.28e+01 - 3.05e-01 1.00e+00h 1
34 -3.2588028e+01 7.90e+00 5.73e+01 -1.8 1.17e+01 - 4.37e-01 1.00e+00h 1
35 -3.3620576e+01 7.72e-01 5.19e+01 -1.8 2.16e+00 - 6.48e-01 1.00e+00h 1
36 -4.2336652e+01 4.82e+00 5.51e+01 -1.8 8.72e+00 - 1.00e+00 1.00e+00f 1
37 -5.3051428e+01 4.61e+00 4.57e+01 -2.5 1.07e+01 - 7.74e-01 1.00e+00f 1
38 -5.1223827e+01 4.41e-01 1.99e+02 -3.4 4.17e+00 - 1.00e+00 1.00e+00h 1
39 -5.6128917e+01 1.28e+00 1.96e+02 -3.3 6.17e+00 - 1.00e+00 1.00e+00f 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
40 -5.8662021e+01 1.79e-01 2.72e+01 -3.8 2.53e+00 - 1.00e+00 1.00e+00h 1
41 -5.5517957e+01 2.50e-01 7.91e+00 -2.9 4.65e+00 - 1.00e+00 6.52e-01H 1
42 -5.3728763e+01 6.51e-03 9.46e-01 -3.0 1.79e+00 - 1.00e+00 1.00e+00h 1
43 -5.3419698e+01 4.76e-03 2.86e+00 -4.5 6.36e-01 - 1.00e+00 1.00e+00h 1
44 -5.3477302e+01 1.35e-03 5.82e-01 -6.1 6.54e-02 - 1.00e+00 1.00e+00h 1
45 -5.3412273e+01 6.95e-04 2.79e-01 -6.4 7.34e-02 - 1.00e+00 1.00e+00h 1
46 -5.3381366e+01 1.56e-04 4.94e-02 -8.3 3.09e-02 - 1.00e+00 1.00e+00h 1
47 -5.3389483e+01 2.02e-05 7.89e-02 -10.1 8.12e-03 - 1.00e+00 1.00e+00h 1
48 -5.3388306e+01 1.89e-05 2.01e-03 -11.0 3.83e-03 - 1.00e+00 1.00e+00h 1
49 -5.3387459e+01 1.26e-05 1.16e-03 -11.0 8.47e-04 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
50 -5.3388067e+01 1.68e-06 5.09e-04 -11.0 6.08e-04 - 1.00e+00 1.00e+00h 1
51 -5.3387971e+01 1.88e-07 7.05e-05 -11.0 9.55e-05 - 1.00e+00 1.00e+00h 1
52 -5.3387977e+01 3.14e-07 1.33e-03 -11.0 9.28e-05 - 1.00e+00 1.00e+00h 1
53 -5.3387991e+01 3.17e-07 1.86e-06 -11.0 7.91e-05 - 1.00e+00 1.00e+00h 1
54 -5.3387976e+01 4.13e-08 3.35e-09 -11.0 1.52e-05 - 1.00e+00 1.00e+00h 1
55 -5.3387979e+01 6.41e-09 2.02e-08 -11.0 2.35e-06 - 1.00e+00 1.00e+00h 1
56 -5.3387979e+01 1.61e-08 1.01e-08 -11.0 2.99e-07 - 1.00e+00 1.00e+00h 1
57 -5.3387978e+01 7.12e-09 1.69e-09 -11.0 7.53e-07 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 57
(scaled) (unscaled)
Objective...............: -5.3387978099370649e+01 -5.3387978099370649e+01
Dual infeasibility......: 1.6926743340306416e-09 1.6926743340306416e-09
Constraint violation....: 7.1150076961575379e-09 7.1150076961575379e-09
Variable bound violation: 9.9994695856864269e-09 9.9994695856864269e-09
Complementarity.........: 1.0000138534512874e-11 1.0000138534512874e-11
Overall NLP error.......: 7.1150076961575379e-09 7.1150076961575379e-09
Number of objective function evaluations = 68
Number of objective gradient evaluations = 58
Number of equality constraint evaluations = 68
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 58
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 0
Total seconds in IPOPT = 6.345
EXIT: Optimal Solution Found.
Simulating trajectory traj
Done simulating trajectory traj
Launch angle: 85.0000 deg
Flight angle at end of propulsion: 83.9875 deg
Empty mass: 0.1449 kg
Water volume: 0.9839 L
Maximum range: 16.2942 m
Maximum height: 53.3880 m
Maximum velocity: 46.1508 m/s
Maximum Height Solution: Propulsive Phase#
plot_propelled_ascent(sol_out, sim_out)
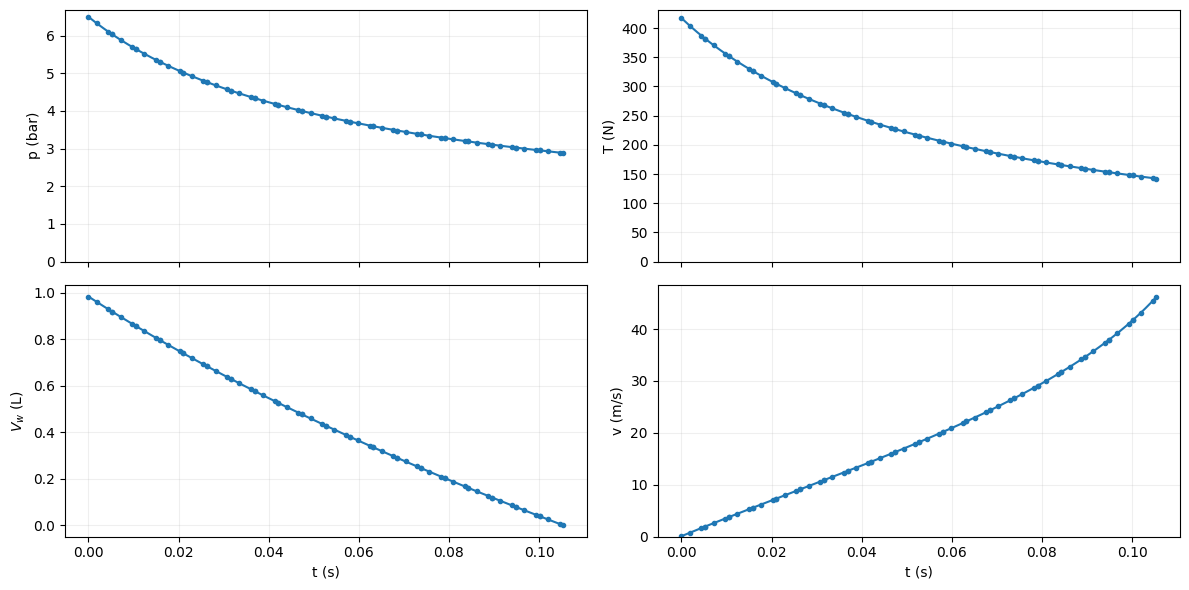
Maximum Height Solution: Height vs. Range#
Note that the equations of motion used here are singular in vertical flight, so the launch angle (the initial flight path angle) was limited to 85 degrees.
plot_trajectory(sol_out, sim_out, legend_loc='center right')
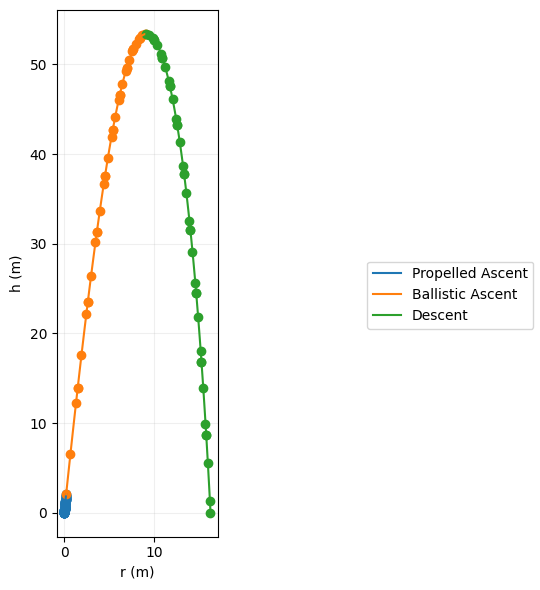
Maximum Height Solution: State History#
plot_states(sol_out, sim_out, legend_loc='lower center', legend_ncol=3)
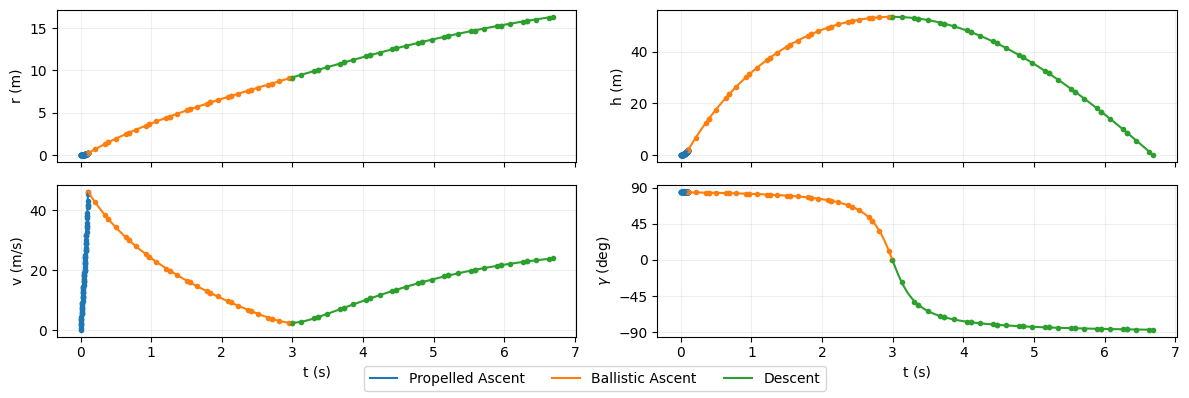
Optimizing for Range#
from dymos.examples.water_rocket.phases import new_water_rocket_trajectory, set_sane_initial_guesses
p = om.Problem(model=om.Group())
traj, phases = new_water_rocket_trajectory(objective='range')
traj = p.model.add_subsystem('traj', traj)
p.driver = om.pyOptSparseDriver(optimizer='IPOPT')
p.driver.opt_settings['print_level'] = 5
p.driver.opt_settings['max_iter'] = 1000
p.driver.opt_settings['mu_strategy'] = 'adaptive'
p.driver.opt_settings['nlp_scaling_method'] = 'gradient-based' # for faster convergence
p.driver.opt_settings['alpha_for_y'] = 'safer-min-dual-infeas'
p.driver.declare_coloring(tol=1.0E-12)
# Finish Problem Setup
p.model.linear_solver = om.DirectSolver()
p.setup()
set_sane_initial_guesses(phases)
dm.run_problem(p, run_driver=True, simulate=True)
summary = summarize_results(p)
for key, entry in summary.items():
print(f'{key}: {entry.value:6.4f} {entry.unit}')
sol_out = om.CaseReader(p.get_outputs_dir() / 'dymos_solution.db').get_case('final')
sim_out = om.CaseReader(traj.sim_prob.get_outputs_dir() / 'dymos_simulation.db').get_case('final')
'rhs_checking' is disabled for 'DirectSolver in <model> <class Group>' but that solver has redundant adjoint solves. If it is expensive to compute derivatives for this solver, turning on 'rhs_checking' may improve performance.
Jacobian shape: (611, 613) (1.23% nonzero)
FWD solves: 20 REV solves: 0
Total colors vs. total size: 20 vs 613 (96.74% improvement)
Sparsity computed using tolerance: 1e-12.
Dense total jacobian for Problem 'problem6' was computed 3 times.
Time to compute sparsity: 0.5215 sec
Time to compute coloring: 0.8194 sec
Memory to compute coloring: 0.0000 MB
Coloring created on: 2025-04-10 15:46:03
List of user-set options:
Name Value used
alpha_for_y = safer-min-dual-infeas yes
file_print_level = 5 yes
hessian_approximation = limited-memory yes
linear_solver = mumps yes
max_iter = 1000 yes
mu_strategy = adaptive yes
nlp_scaling_method = gradient-based yes
output_file = /home/runner/work/dymos/dymos/docs/dymos_book/examples/water_rocket/problem6_out/IPOPT.out yes
print_level = 5 yes
print_user_options = yes yes
sb = yes yes
This is Ipopt version 3.14.12, running with linear solver MUMPS 5.2.1.
Number of nonzeros in equality constraint Jacobian...: 103007
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 613
variables with only lower bounds: 180
variables with lower and upper bounds: 67
variables with only upper bounds: 30
Total number of equality constraints.................: 610
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 -2.0000000e+01 9.00e+01 3.60e-01 0.0 0.00e+00 - 0.00e+00 0.00e+00 0
1 -1.3188387e+01 2.49e+01 6.36e+03 -5.8 4.98e+01 - 1.85e-01 7.23e-01h 1
2 -3.5089577e+01 7.07e+00 2.76e+04 0.6 4.19e+01 - 1.00e+00 7.63e-01h 1
3 -3.5976015e+01 6.87e+01 1.55e+06 1.8 6.90e+01 - 1.00e+00 3.48e-01f 1
4 -5.5522114e+01 1.36e+02 1.39e+05 2.3 6.51e+01 - 6.72e-01 1.00e+00f 1
5 -4.3438267e+01 5.27e+01 8.03e+04 2.3 2.98e+01 - 1.00e+00 1.00e+00f 1
6 -3.7806064e+01 1.10e+02 2.36e+05 2.1 4.99e+01 - 9.72e-01 1.00e+00f 1
7 -5.6571012e+01 1.16e+02 6.64e+04 2.6 4.77e+01 - 1.00e+00 4.78e-01h 1
8 -6.9028277e+01 2.97e+01 1.13e+05 2.4 3.16e+01 - 9.42e-01 1.00e+00h 1
9 -3.7879082e+01 1.64e+00 2.40e+04 3.2 3.11e+01 - 5.60e-01 1.00e+00f 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
10 -5.3052215e+01 8.81e-01 1.21e+06 2.5 1.52e+01 - 9.82e-01 1.00e+00f 1
11 -5.2073297e+01 4.41e-03 1.48e+04 0.9 9.79e-01 - 1.00e+00 1.00e+00f 1
12 -6.1190692e+01 1.18e+00 2.98e+02 0.4 9.12e+00 - 1.00e+00 1.00e+00f 1
13 -7.0770515e+01 1.06e+00 4.12e+02 -0.8 9.58e+00 - 1.00e+00 1.00e+00f 1
14 -7.8653577e+01 7.43e-01 1.87e+02 -1.4 7.88e+00 - 1.00e+00 1.00e+00f 1
15 -8.2020155e+01 3.05e-01 3.01e+01 -2.5 4.33e+00 - 1.00e+00 1.00e+00f 1
16 -8.3490933e+01 1.42e-01 3.49e+01 -3.3 2.99e+00 - 1.00e+00 1.00e+00h 1
17 -8.4810499e+01 1.85e-01 4.24e+01 -3.6 1.93e+00 - 1.00e+00 1.00e+00h 1
18 -8.5174582e+01 4.51e-01 1.28e+02 -3.9 9.23e+00 - 1.00e+00 1.00e+00h 1
19 -8.4976003e+01 2.51e-02 2.77e+01 -4.6 3.97e+00 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
20 -8.6314900e+01 5.24e-02 2.10e+01 -5.2 5.93e+00 - 1.00e+00 1.00e+00f 1
21 -8.2646846e+01 4.62e-02 1.80e+01 -5.9 3.67e+00 - 1.00e+00 1.00e+00h 1
22 -8.6622097e+01 7.78e-02 3.98e+01 -6.0 4.92e+00 - 1.00e+00 1.00e+00f 1
23 -8.9263527e+01 1.58e-01 1.65e+01 -6.5 3.44e+00 - 1.00e+00 1.00e+00f 1
24 -7.9904100e+01 7.83e-02 4.89e+01 -7.1 1.43e+01 - 8.74e-01 1.00e+00H 1
25 -8.9203642e+01 3.89e-01 1.56e+01 -7.1 1.02e+01 - 1.00e+00 1.00e+00f 1
26 -8.5063228e+01 3.17e-02 1.76e+01 -7.1 4.78e+00 - 1.00e+00 1.00e+00h 1
27 -8.5899719e+01 1.08e-02 3.37e+00 -8.4 1.32e+00 - 1.00e+00 1.00e+00h 1
28 -8.4985862e+01 8.78e-04 3.35e+00 -9.9 9.14e-01 - 1.00e+00 1.00e+00h 1
29 -8.5061347e+01 6.79e-04 4.50e-01 -11.0 3.11e-01 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
30 -8.5098262e+01 2.30e-03 2.81e+00 -11.0 1.67e-01 - 1.00e+00 1.00e+00h 1
31 -8.5259423e+01 2.73e-03 9.01e-02 -11.0 1.61e-01 - 1.00e+00 1.00e+00h 1
32 -8.5063573e+01 7.54e-04 2.18e-01 -11.0 1.96e-01 - 1.00e+00 1.00e+00h 1
33 -8.5121746e+01 4.72e-04 1.02e-01 -11.0 5.82e-02 -4.0 1.00e+00 1.00e+00h 1
34 -8.5087361e+01 1.45e-04 9.09e-02 -11.0 3.44e-02 - 1.00e+00 1.00e+00h 1
35 -8.5098518e+01 3.63e-04 4.81e-01 -11.0 7.02e-02 - 1.00e+00 1.00e+00h 1
36 -8.5123296e+01 4.24e-04 4.41e-02 -11.0 7.29e-02 - 1.00e+00 1.00e+00h 1
37 -8.5065136e+01 3.40e-05 3.69e-01 -11.0 8.29e-02 - 1.00e+00 1.00e+00H 1
38 -8.5069201e+01 3.28e-04 2.45e-01 -11.0 7.87e-02 - 1.00e+00 1.00e+00h 1
39 -8.5091462e+01 1.56e-03 1.76e+00 -11.0 1.17e-01 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
40 -8.5199138e+01 1.69e-03 1.17e-01 -11.0 1.08e-01 - 1.00e+00 1.00e+00h 1
41 -8.5078090e+01 3.15e-04 1.48e-01 -11.0 1.21e-01 - 1.00e+00 1.00e+00h 1
42 -8.5103655e+01 1.18e-04 7.75e-03 -11.0 2.56e-02 - 1.00e+00 1.00e+00h 1
43 -8.5122256e+01 3.20e-04 1.92e-01 -11.0 9.19e-02 - 1.00e+00 1.00e+00H 1
44 -8.5099796e+01 6.33e-05 1.88e-03 -11.0 2.81e-02 - 1.00e+00 1.00e+00h 1
45 -8.5096183e+01 1.19e-05 9.41e-03 -11.0 3.61e-03 - 1.00e+00 1.00e+00h 1
46 -8.5097107e+01 1.06e-05 5.50e-03 -11.0 1.66e-03 - 1.00e+00 1.00e+00h 1
47 -8.5096350e+01 6.82e-06 2.07e-03 -11.0 7.57e-04 - 1.00e+00 1.00e+00h 1
48 -8.5096843e+01 3.13e-06 3.20e-03 -11.0 4.93e-04 - 1.00e+00 1.00e+00h 1
49 -8.5096612e+01 1.75e-06 1.30e-04 -11.0 2.31e-04 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
50 -8.5096740e+01 2.86e-07 1.43e-04 -11.0 1.28e-04 - 1.00e+00 1.00e+00h 1
51r-8.5096740e+01 2.86e-07 9.99e+02 -6.5 0.00e+00 - 0.00e+00 4.77e-07R 22
52r-8.5096729e+01 6.07e-08 1.15e-02 -9.0 1.15e-02 - 1.00e+00 9.90e-04f 1
53 -8.5096719e+01 3.47e-06 1.10e-03 -11.0 4.10e-04 -4.5 1.00e+00 1.00e+00s 22
54 -8.5096482e+01 3.72e-06 3.92e-04 -11.0 4.23e-04 - 1.00e+00 1.00e+00s 22
55 -8.5096744e+01 3.79e-07 1.94e-04 -11.0 2.62e-04 - 1.00e+00 0.00e+00S 22
56 -8.5097253e+01 5.93e-06 9.24e-03 -11.0 9.51e-04 - 1.00e+00 1.00e+00H 1
57 -8.5097642e+01 1.59e-05 1.45e-04 -11.0 1.78e-03 - 1.00e+00 1.00e+00h 1
58 -8.5096539e+01 3.43e-06 4.85e-03 -11.0 1.10e-03 - 1.00e+00 1.00e+00h 1
59 -8.5096810e+01 2.65e-06 2.40e-03 -11.0 3.40e-04 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
60 -8.5096620e+01 1.49e-06 6.17e-05 -11.0 1.91e-04 - 1.00e+00 1.00e+00h 1
61 -8.5096728e+01 3.66e-07 1.01e-04 -11.0 1.09e-04 - 1.00e+00 1.00e+00h 1
62 -8.5096738e+01 5.50e-08 9.81e-07 -11.0 6.21e-05 - 1.00e+00 1.00e+00H 1
63 -8.5096739e+01 4.86e-07 8.23e-06 -11.0 5.10e-05 - 1.00e+00 1.00e+00h 1
64 -8.5096706e+01 5.02e-08 2.75e-07 -11.0 3.33e-05 - 1.00e+00 1.00e+00h 1
65 -8.5096713e+01 2.14e-08 1.28e-08 -11.0 1.62e-05 - 1.00e+00 1.00e+00H 1
66 -8.5096711e+01 2.14e-09 8.22e-09 -11.0 1.90e-06 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 66
(scaled) (unscaled)
Objective...............: -8.5096710893053995e+01 -8.5096710893053995e+01
Dual infeasibility......: 8.2222963030657457e-09 8.2222963030657457e-09
Constraint violation....: 2.1436326930837837e-09 2.1436326930837837e-09
Variable bound violation: 0.0000000000000000e+00 0.0000000000000000e+00
Complementarity.........: 1.0000000000000001e-11 1.0000000000000001e-11
Overall NLP error.......: 8.2222963030657457e-09 8.2222963030657457e-09
Number of objective function evaluations = 124
Number of objective gradient evaluations = 67
Number of equality constraint evaluations = 124
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 68
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 0
Total seconds in IPOPT = 7.211
EXIT: Optimal Solution Found.
Optimization Problem -- Optimization using pyOpt_sparse
================================================================================
Objective Function: _objfunc
Solution:
--------------------------------------------------------------------------------
Total Time: 7.2121
User Objective Time : 0.4044
User Sensitivity Time : 3.8419
Interface Time : 1.1460
Opt Solver Time: 1.8199
Calls to Objective Function : 125
Calls to Sens Function : 69
Objectives
Index Name Value
0 traj.descent.states:r -8.509671E+01
Variables (c - continuous, i - integer, d - discrete)
Index Name Type Lower Bound Value Upper Bound Status
0 traj.parameters:m_empty_0 c 1.000000E-02 1.891068E+00 1.000000E+01
1 traj.ballistic_ascent.t_initial_0 c 1.000000E-03 1.127359E-01 1.000000E+00
2 traj.ballistic_ascent.t_duration_0 c 1.000000E-03 1.928639E+00 1.000000E+01
3 traj.ballistic_ascent.states:r_0 c -1.000000E+21 1.609883E+00 1.000000E+21
4 traj.ballistic_ascent.states:r_1 c -1.000000E+21 3.796505E+00 1.000000E+21
5 traj.ballistic_ascent.states:r_2 c -1.000000E+21 6.719126E+00 1.000000E+21
6 traj.ballistic_ascent.states:r_3 c -1.000000E+21 7.622675E+00 1.000000E+21
7 traj.ballistic_ascent.states:r_4 c -1.000000E+21 9.654936E+00 1.000000E+21
8 traj.ballistic_ascent.states:r_5 c -1.000000E+21 1.237962E+01 1.000000E+21
9 traj.ballistic_ascent.states:r_6 c -1.000000E+21 1.322386E+01 1.000000E+21
10 traj.ballistic_ascent.states:r_7 c -1.000000E+21 1.512589E+01 1.000000E+21
11 traj.ballistic_ascent.states:r_8 c -1.000000E+21 1.768270E+01 1.000000E+21
12 traj.ballistic_ascent.states:r_9 c -1.000000E+21 1.847644E+01 1.000000E+21
13 traj.ballistic_ascent.states:r_10 c -1.000000E+21 2.026728E+01 1.000000E+21
14 traj.ballistic_ascent.states:r_11 c -1.000000E+21 2.268007E+01 1.000000E+21
15 traj.ballistic_ascent.states:r_12 c -1.000000E+21 2.343033E+01 1.000000E+21
16 traj.ballistic_ascent.states:r_13 c -1.000000E+21 2.512518E+01 1.000000E+21
17 traj.ballistic_ascent.states:r_14 c -1.000000E+21 2.741311E+01 1.000000E+21
18 traj.ballistic_ascent.states:r_15 c -1.000000E+21 2.812555E+01 1.000000E+21
19 traj.ballistic_ascent.states:r_16 c -1.000000E+21 2.973668E+01 1.000000E+21
20 traj.ballistic_ascent.states:r_17 c -1.000000E+21 3.191524E+01 1.000000E+21
21 traj.ballistic_ascent.states:r_18 c -1.000000E+21 3.259445E+01 1.000000E+21
22 traj.ballistic_ascent.states:r_19 c -1.000000E+21 3.413183E+01 1.000000E+21
23 traj.ballistic_ascent.states:r_20 c -1.000000E+21 3.621365E+01 1.000000E+21
24 traj.ballistic_ascent.states:r_21 c -1.000000E+21 3.686338E+01 1.000000E+21
25 traj.ballistic_ascent.states:r_22 c -1.000000E+21 3.833514E+01 1.000000E+21
26 traj.ballistic_ascent.states:r_23 c -1.000000E+21 4.033049E+01 1.000000E+21
27 traj.ballistic_ascent.states:r_24 c -1.000000E+21 4.095376E+01 1.000000E+21
28 traj.ballistic_ascent.states:r_25 c -1.000000E+21 4.236652E+01 1.000000E+21
29 traj.ballistic_ascent.states:r_26 c -1.000000E+21 4.428375E+01 1.000000E+21
30 traj.ballistic_ascent.states:r_27 c -1.000000E+21 4.488304E+01 1.000000E+21
31 traj.ballistic_ascent.states:r_28 c -1.000000E+21 4.624213E+01 1.000000E+21
32 traj.ballistic_ascent.states:r_29 c -1.000000E+21 4.808798E+01 1.000000E+21
33 traj.ballistic_ascent.states:r_30 c -1.000000E+21 4.866527E+01 1.000000E+21
34 traj.ballistic_ascent.states:h_0 c -1.000000E+21 1.305634E+00 1.000000E+21
35 traj.ballistic_ascent.states:h_1 c -1.000000E+21 3.015243E+00 1.000000E+21
36 traj.ballistic_ascent.states:h_2 c -1.000000E+21 5.226005E+00 1.000000E+21
37 traj.ballistic_ascent.states:h_3 c -1.000000E+21 5.891426E+00 1.000000E+21
38 traj.ballistic_ascent.states:h_4 c -1.000000E+21 7.355373E+00 1.000000E+21
39 traj.ballistic_ascent.states:h_5 c -1.000000E+21 9.243708E+00 1.000000E+21
40 traj.ballistic_ascent.states:h_6 c -1.000000E+21 9.810733E+00 1.000000E+21
41 traj.ballistic_ascent.states:h_7 c -1.000000E+21 1.105548E+01 1.000000E+21
42 traj.ballistic_ascent.states:h_8 c -1.000000E+21 1.265423E+01 1.000000E+21
43 traj.ballistic_ascent.states:h_9 c -1.000000E+21 1.313246E+01 1.000000E+21
44 traj.ballistic_ascent.states:h_10 c -1.000000E+21 1.417867E+01 1.000000E+21
45 traj.ballistic_ascent.states:h_11 c -1.000000E+21 1.551366E+01 1.000000E+21
46 traj.ballistic_ascent.states:h_12 c -1.000000E+21 1.591067E+01 1.000000E+21
47 traj.ballistic_ascent.states:h_13 c -1.000000E+21 1.677474E+01 1.000000E+21
48 traj.ballistic_ascent.states:h_14 c -1.000000E+21 1.786652E+01 1.000000E+21
49 traj.ballistic_ascent.states:h_15 c -1.000000E+21 1.818839E+01 1.000000E+21
50 traj.ballistic_ascent.states:h_16 c -1.000000E+21 1.888344E+01 1.000000E+21
51 traj.ballistic_ascent.states:h_17 c -1.000000E+21 1.974857E+01 1.000000E+21
52 traj.ballistic_ascent.states:h_18 c -1.000000E+21 2.000017E+01 1.000000E+21
53 traj.ballistic_ascent.states:h_19 c -1.000000E+21 2.053684E+01 1.000000E+21
54 traj.ballistic_ascent.states:h_20 c -1.000000E+21 2.118879E+01 1.000000E+21
55 traj.ballistic_ascent.states:h_21 c -1.000000E+21 2.137413E+01 1.000000E+21
56 traj.ballistic_ascent.states:h_22 c -1.000000E+21 2.176113E+01 1.000000E+21
57 traj.ballistic_ascent.states:h_23 c -1.000000E+21 2.221099E+01 1.000000E+21
58 traj.ballistic_ascent.states:h_24 c -1.000000E+21 2.233338E+01 1.000000E+21
59 traj.ballistic_ascent.states:h_25 c -1.000000E+21 2.257793E+01 1.000000E+21
60 traj.ballistic_ascent.states:h_26 c -1.000000E+21 2.283496E+01 1.000000E+21
61 traj.ballistic_ascent.states:h_27 c -1.000000E+21 2.289716E+01 1.000000E+21
62 traj.ballistic_ascent.states:h_28 c -1.000000E+21 2.300536E+01 1.000000E+21
63 traj.ballistic_ascent.states:h_29 c -1.000000E+21 2.307744E+01 1.000000E+21
64 traj.ballistic_ascent.states:h_30 c -1.000000E+21 2.308183E+01 1.000000E+21
65 traj.ballistic_ascent.states:gam_0 c -1.111111E+19 4.265399E-01 9.888889E-01
66 traj.ballistic_ascent.states:gam_1 c -1.111111E+19 4.182297E-01 9.888889E-01
67 traj.ballistic_ascent.states:gam_2 c -1.111111E+19 4.060968E-01 9.888889E-01
68 traj.ballistic_ascent.states:gam_3 c -1.111111E+19 4.020906E-01 9.888889E-01
69 traj.ballistic_ascent.states:gam_4 c -1.111111E+19 3.926055E-01 9.888889E-01
70 traj.ballistic_ascent.states:gam_5 c -1.111111E+19 3.787840E-01 9.888889E-01
71 traj.ballistic_ascent.states:gam_6 c -1.111111E+19 3.742266E-01 9.888889E-01
72 traj.ballistic_ascent.states:gam_7 c -1.111111E+19 3.634492E-01 9.888889E-01
73 traj.ballistic_ascent.states:gam_8 c -1.111111E+19 3.477750E-01 9.888889E-01
74 traj.ballistic_ascent.states:gam_9 c -1.111111E+19 3.426144E-01 9.888889E-01
75 traj.ballistic_ascent.states:gam_10 c -1.111111E+19 3.304257E-01 9.888889E-01
76 traj.ballistic_ascent.states:gam_11 c -1.111111E+19 3.127375E-01 9.888889E-01
77 traj.ballistic_ascent.states:gam_12 c -1.111111E+19 3.069240E-01 9.888889E-01
78 traj.ballistic_ascent.states:gam_13 c -1.111111E+19 2.932134E-01 9.888889E-01
79 traj.ballistic_ascent.states:gam_14 c -1.111111E+19 2.733693E-01 9.888889E-01
80 traj.ballistic_ascent.states:gam_15 c -1.111111E+19 2.668611E-01 9.888889E-01
81 traj.ballistic_ascent.states:gam_16 c -1.111111E+19 2.515410E-01 9.888889E-01
82 traj.ballistic_ascent.states:gam_17 c -1.111111E+19 2.294415E-01 9.888889E-01
83 traj.ballistic_ascent.states:gam_18 c -1.111111E+19 2.222134E-01 9.888889E-01
84 traj.ballistic_ascent.states:gam_19 c -1.111111E+19 2.052394E-01 9.888889E-01
85 traj.ballistic_ascent.states:gam_20 c -1.111111E+19 1.808587E-01 9.888889E-01
86 traj.ballistic_ascent.states:gam_21 c -1.111111E+19 1.729123E-01 9.888889E-01
87 traj.ballistic_ascent.states:gam_22 c -1.111111E+19 1.543078E-01 9.888889E-01
88 traj.ballistic_ascent.states:gam_23 c -1.111111E+19 1.277288E-01 9.888889E-01
89 traj.ballistic_ascent.states:gam_24 c -1.111111E+19 1.191038E-01 9.888889E-01
90 traj.ballistic_ascent.states:gam_25 c -1.111111E+19 9.898556E-02 9.888889E-01
91 traj.ballistic_ascent.states:gam_26 c -1.111111E+19 7.043261E-02 9.888889E-01
92 traj.ballistic_ascent.states:gam_27 c -1.111111E+19 6.121588E-02 9.888889E-01
93 traj.ballistic_ascent.states:gam_28 c -1.111111E+19 3.981308E-02 9.888889E-01
94 traj.ballistic_ascent.states:gam_29 c -1.111111E+19 9.670357E-03 9.888889E-01
95 traj.ballistic_ascent.states:v_0 c -1.000000E+21 4.130291E+01 1.000000E+21
96 traj.ballistic_ascent.states:v_1 c -1.000000E+21 3.977982E+01 1.000000E+21
97 traj.ballistic_ascent.states:v_2 c -1.000000E+21 3.781946E+01 1.000000E+21
98 traj.ballistic_ascent.states:v_3 c -1.000000E+21 3.723047E+01 1.000000E+21
99 traj.ballistic_ascent.states:v_4 c -1.000000E+21 3.593495E+01 1.000000E+21
100 traj.ballistic_ascent.states:v_5 c -1.000000E+21 3.426083E+01 1.000000E+21
101 traj.ballistic_ascent.states:v_6 c -1.000000E+21 3.375652E+01 1.000000E+21
102 traj.ballistic_ascent.states:v_7 c -1.000000E+21 3.264529E+01 1.000000E+21
103 traj.ballistic_ascent.states:v_8 c -1.000000E+21 3.120589E+01 1.000000E+21
104 traj.ballistic_ascent.states:v_9 c -1.000000E+21 3.077167E+01 1.000000E+21
105 traj.ballistic_ascent.states:v_10 c -1.000000E+21 2.981417E+01 1.000000E+21
106 traj.ballistic_ascent.states:v_11 c -1.000000E+21 2.857299E+01 1.000000E+21
107 traj.ballistic_ascent.states:v_12 c -1.000000E+21 2.819855E+01 1.000000E+21
108 traj.ballistic_ascent.states:v_13 c -1.000000E+21 2.737310E+01 1.000000E+21
109 traj.ballistic_ascent.states:v_14 c -1.000000E+21 2.630434E+01 1.000000E+21
110 traj.ballistic_ascent.states:v_15 c -1.000000E+21 2.598238E+01 1.000000E+21
111 traj.ballistic_ascent.states:v_16 c -1.000000E+21 2.527374E+01 1.000000E+21
112 traj.ballistic_ascent.states:v_17 c -1.000000E+21 2.435930E+01 1.000000E+21
113 traj.ballistic_ascent.states:v_18 c -1.000000E+21 2.408474E+01 1.000000E+21
114 traj.ballistic_ascent.states:v_19 c -1.000000E+21 2.348227E+01 1.000000E+21
115 traj.ballistic_ascent.states:v_20 c -1.000000E+21 2.270963E+01 1.000000E+21
116 traj.ballistic_ascent.states:v_21 c -1.000000E+21 2.247894E+01 1.000000E+21
117 traj.ballistic_ascent.states:v_22 c -1.000000E+21 2.197533E+01 1.000000E+21
118 traj.ballistic_ascent.states:v_23 c -1.000000E+21 2.133578E+01 1.000000E+21
119 traj.ballistic_ascent.states:v_24 c -1.000000E+21 2.114650E+01 1.000000E+21
120 traj.ballistic_ascent.states:v_25 c -1.000000E+21 2.073654E+01 1.000000E+21
121 traj.ballistic_ascent.states:v_26 c -1.000000E+21 2.022371E+01 1.000000E+21
122 traj.ballistic_ascent.states:v_27 c -1.000000E+21 2.007398E+01 1.000000E+21
123 traj.ballistic_ascent.states:v_28 c -1.000000E+21 1.975357E+01 1.000000E+21
124 traj.ballistic_ascent.states:v_29 c -1.000000E+21 1.936202E+01 1.000000E+21
125 traj.ballistic_ascent.states:v_30 c -1.000000E+21 1.925012E+01 1.000000E+21
126 traj.descent.t_initial_0 c 5.000000E-01 2.041375E+00 1.000000E+02
127 traj.descent.t_duration_0 c 5.000000E-02 2.327564E-01 1.000000E+01
128 traj.descent.states:r_0 c -1.000000E+21 4.866527E+01 1.000000E+21
129 traj.descent.states:r_1 c -1.000000E+21 5.024379E+01 1.000000E+21
130 traj.descent.states:r_2 c -1.000000E+21 5.238226E+01 1.000000E+21
131 traj.descent.states:r_3 c -1.000000E+21 5.304978E+01 1.000000E+21
132 traj.descent.states:r_4 c -1.000000E+21 5.456188E+01 1.000000E+21
133 traj.descent.states:r_5 c -1.000000E+21 5.661146E+01 1.000000E+21
134 traj.descent.states:r_6 c -1.000000E+21 5.725144E+01 1.000000E+21
135 traj.descent.states:r_7 c -1.000000E+21 5.870144E+01 1.000000E+21
136 traj.descent.states:r_8 c -1.000000E+21 6.066730E+01 1.000000E+21
137 traj.descent.states:r_9 c -1.000000E+21 6.128121E+01 1.000000E+21
138 traj.descent.states:r_10 c -1.000000E+21 6.267218E+01 1.000000E+21
139 traj.descent.states:r_11 c -1.000000E+21 6.455796E+01 1.000000E+21
140 traj.descent.states:r_12 c -1.000000E+21 6.514681E+01 1.000000E+21
141 traj.descent.states:r_13 c -1.000000E+21 6.648085E+01 1.000000E+21
142 traj.descent.states:r_14 c -1.000000E+21 6.828900E+01 1.000000E+21
143 traj.descent.states:r_15 c -1.000000E+21 6.885346E+01 1.000000E+21
144 traj.descent.states:r_16 c -1.000000E+21 7.013198E+01 1.000000E+21
145 traj.descent.states:r_17 c -1.000000E+21 7.186414E+01 1.000000E+21
146 traj.descent.states:r_18 c -1.000000E+21 7.240468E+01 1.000000E+21
147 traj.descent.states:r_19 c -1.000000E+21 7.362863E+01 1.000000E+21
148 traj.descent.states:r_20 c -1.000000E+21 7.528593E+01 1.000000E+21
149 traj.descent.states:r_21 c -1.000000E+21 7.580287E+01 1.000000E+21
150 traj.descent.states:r_22 c -1.000000E+21 7.697294E+01 1.000000E+21
151 traj.descent.states:r_23 c -1.000000E+21 7.855623E+01 1.000000E+21
152 traj.descent.states:r_24 c -1.000000E+21 7.904983E+01 1.000000E+21
153 traj.descent.states:r_25 c -1.000000E+21 8.016660E+01 1.000000E+21
154 traj.descent.states:r_26 c -1.000000E+21 8.167668E+01 1.000000E+21
155 traj.descent.states:r_27 c -1.000000E+21 8.214720E+01 1.000000E+21
156 traj.descent.states:r_28 c -1.000000E+21 8.321126E+01 1.000000E+21
157 traj.descent.states:r_29 c -1.000000E+21 8.464899E+01 1.000000E+21
158 traj.descent.states:r_30 c -1.000000E+21 8.509671E+01 1.000000E+21
159 traj.descent.states:h_0 c -1.000000E+21 2.308183E+01 1.000000E+21
160 traj.descent.states:h_1 c -1.000000E+21 2.304852E+01 1.000000E+21
161 traj.descent.states:h_2 c -1.000000E+21 2.289447E+01 1.000000E+21
162 traj.descent.states:h_3 c -1.000000E+21 2.281998E+01 1.000000E+21
163 traj.descent.states:h_4 c -1.000000E+21 2.260341E+01 1.000000E+21
164 traj.descent.states:h_5 c -1.000000E+21 2.220091E+01 1.000000E+21
165 traj.descent.states:h_6 c -1.000000E+21 2.204882E+01 1.000000E+21
166 traj.descent.states:h_7 c -1.000000E+21 2.165640E+01 1.000000E+21
167 traj.descent.states:h_8 c -1.000000E+21 2.101542E+01 1.000000E+21
168 traj.descent.states:h_9 c -1.000000E+21 2.078883E+01 1.000000E+21
169 traj.descent.states:h_10 c -1.000000E+21 2.022762E+01 1.000000E+21
170 traj.descent.states:h_11 c -1.000000E+21 1.935782E+01 1.000000E+21
171 traj.descent.states:h_12 c -1.000000E+21 1.905980E+01 1.000000E+21
172 traj.descent.states:h_13 c -1.000000E+21 1.833682E+01 1.000000E+21
173 traj.descent.states:h_14 c -1.000000E+21 1.724797E+01 1.000000E+21
174 traj.descent.states:h_15 c -1.000000E+21 1.688164E+01 1.000000E+21
175 traj.descent.states:h_16 c -1.000000E+21 1.600408E+01 1.000000E+21
176 traj.descent.states:h_17 c -1.000000E+21 1.470626E+01 1.000000E+21
177 traj.descent.states:h_18 c -1.000000E+21 1.427484E+01 1.000000E+21
178 traj.descent.states:h_19 c -1.000000E+21 1.325019E+01 1.000000E+21
179 traj.descent.states:h_20 c -1.000000E+21 1.175388E+01 1.000000E+21
180 traj.descent.states:h_21 c -1.000000E+21 1.126075E+01 1.000000E+21
181 traj.descent.states:h_22 c -1.000000E+21 1.009678E+01 1.000000E+21
182 traj.descent.states:h_23 c -1.000000E+21 8.412916E+00 1.000000E+21
183 traj.descent.states:h_24 c -1.000000E+21 7.861557E+00 1.000000E+21
184 traj.descent.states:h_25 c -1.000000E+21 6.566341E+00 1.000000E+21
185 traj.descent.states:h_26 c -1.000000E+21 4.706168E+00 1.000000E+21
186 traj.descent.states:h_27 c -1.000000E+21 4.100175E+00 1.000000E+21
187 traj.descent.states:h_28 c -1.000000E+21 2.681974E+00 1.000000E+21
188 traj.descent.states:h_29 c -1.000000E+21 6.569790E-01 1.000000E+21
189 traj.descent.states:gam_0 c -1.000000E+21 -4.203342E-32 1.000000E+21
190 traj.descent.states:gam_1 c -1.000000E+21 -2.429594E+00 1.000000E+21
191 traj.descent.states:gam_2 c -1.000000E+21 -5.827208E+00 1.000000E+21
192 traj.descent.states:gam_3 c -1.000000E+21 -6.910034E+00 1.000000E+21
193 traj.descent.states:gam_4 c -1.000000E+21 -9.396378E+00 1.000000E+21
194 traj.descent.states:gam_5 c -1.000000E+21 -1.282741E+01 1.000000E+21
195 traj.descent.states:gam_6 c -1.000000E+21 -1.390973E+01 1.000000E+21
196 traj.descent.states:gam_7 c -1.000000E+21 -1.637513E+01 1.000000E+21
197 traj.descent.states:gam_8 c -1.000000E+21 -1.973335E+01 1.000000E+21
198 traj.descent.states:gam_9 c -1.000000E+21 -2.078240E+01 1.000000E+21
199 traj.descent.states:gam_10 c -1.000000E+21 -2.315455E+01 1.000000E+21
200 traj.descent.states:gam_11 c -1.000000E+21 -2.634838E+01 1.000000E+21
201 traj.descent.states:gam_12 c -1.000000E+21 -2.733766E+01 1.000000E+21
202 traj.descent.states:gam_13 c -1.000000E+21 -2.956081E+01 1.000000E+21
203 traj.descent.states:gam_14 c -1.000000E+21 -3.252558E+01 1.000000E+21
204 traj.descent.states:gam_15 c -1.000000E+21 -3.343774E+01 1.000000E+21
205 traj.descent.states:gam_16 c -1.000000E+21 -3.547777E+01 1.000000E+21
206 traj.descent.states:gam_17 c -1.000000E+21 -3.817886E+01 1.000000E+21
207 traj.descent.states:gam_18 c -1.000000E+21 -3.900583E+01 1.000000E+21
208 traj.descent.states:gam_19 c -1.000000E+21 -4.084906E+01 1.000000E+21
209 traj.descent.states:gam_20 c -1.000000E+21 -4.327760E+01 1.000000E+21
210 traj.descent.states:gam_21 c -1.000000E+21 -4.401874E+01 1.000000E+21
211 traj.descent.states:gam_22 c -1.000000E+21 -4.566709E+01 1.000000E+21
212 traj.descent.states:gam_23 c -1.000000E+21 -4.783236E+01 1.000000E+21
213 traj.descent.states:gam_24 c -1.000000E+21 -4.849194E+01 1.000000E+21
214 traj.descent.states:gam_25 c -1.000000E+21 -4.995719E+01 1.000000E+21
215 traj.descent.states:gam_26 c -1.000000E+21 -5.187907E+01 1.000000E+21
216 traj.descent.states:gam_27 c -1.000000E+21 -5.246405E+01 1.000000E+21
217 traj.descent.states:gam_28 c -1.000000E+21 -5.376301E+01 1.000000E+21
218 traj.descent.states:gam_29 c -1.000000E+21 -5.546613E+01 1.000000E+21
219 traj.descent.states:gam_30 c -1.000000E+21 -5.598449E+01 1.000000E+21
220 traj.descent.states:v_0 c -1.000000E+21 1.925012E+01 1.000000E+21
221 traj.descent.states:v_1 c -1.000000E+21 1.897038E+01 1.000000E+21
222 traj.descent.states:v_2 c -1.000000E+21 1.865397E+01 1.000000E+21
223 traj.descent.states:v_3 c -1.000000E+21 1.857022E+01 1.000000E+21
224 traj.descent.states:v_4 c -1.000000E+21 1.840725E+01 1.000000E+21
225 traj.descent.states:v_5 c -1.000000E+21 1.824609E+01 1.000000E+21
226 traj.descent.states:v_6 c -1.000000E+21 1.820993E+01 1.000000E+21
227 traj.descent.states:v_7 c -1.000000E+21 1.815288E+01 1.000000E+21
228 traj.descent.states:v_8 c -1.000000E+21 1.813033E+01 1.000000E+21
229 traj.descent.states:v_9 c -1.000000E+21 1.813609E+01 1.000000E+21
230 traj.descent.states:v_10 c -1.000000E+21 1.817131E+01 1.000000E+21
231 traj.descent.states:v_11 c -1.000000E+21 1.826717E+01 1.000000E+21
232 traj.descent.states:v_12 c -1.000000E+21 1.830818E+01 1.000000E+21
233 traj.descent.states:v_13 c -1.000000E+21 1.841999E+01 1.000000E+21
234 traj.descent.states:v_14 c -1.000000E+21 1.861200E+01 1.000000E+21
235 traj.descent.states:v_15 c -1.000000E+21 1.868112E+01 1.000000E+21
236 traj.descent.states:v_16 c -1.000000E+21 1.885314E+01 1.000000E+21
237 traj.descent.states:v_17 c -1.000000E+21 1.911888E+01 1.000000E+21
238 traj.descent.states:v_18 c -1.000000E+21 1.920912E+01 1.000000E+21
239 traj.descent.states:v_19 c -1.000000E+21 1.942560E+01 1.000000E+21
240 traj.descent.states:v_20 c -1.000000E+21 1.974412E+01 1.000000E+21
241 traj.descent.states:v_21 c -1.000000E+21 1.984907E+01 1.000000E+21
242 traj.descent.states:v_22 c -1.000000E+21 2.009583E+01 1.000000E+21
243 traj.descent.states:v_23 c -1.000000E+21 2.044870E+01 1.000000E+21
244 traj.descent.states:v_24 c -1.000000E+21 2.056284E+01 1.000000E+21
245 traj.descent.states:v_25 c -1.000000E+21 2.082776E+01 1.000000E+21
246 traj.descent.states:v_26 c -1.000000E+21 2.119958E+01 1.000000E+21
247 traj.descent.states:v_27 c -1.000000E+21 2.131832E+01 1.000000E+21
248 traj.descent.states:v_28 c -1.000000E+21 2.159151E+01 1.000000E+21
249 traj.descent.states:v_29 c -1.000000E+21 2.196983E+01 1.000000E+21
250 traj.descent.states:v_30 c -1.000000E+21 2.208956E+01 1.000000E+21
251 traj.propelled_ascent.t_duration_0 c 1.000000E-02 1.127359E+00 5.000000E+00
252 traj.propelled_ascent.states:r_0 c -1.000000E+19 6.330634E-06 1.000000E+19
253 traj.propelled_ascent.states:r_1 c -1.000000E+19 3.105194E-05 1.000000E+19
254 traj.propelled_ascent.states:r_2 c -1.000000E+19 4.265111E-05 1.000000E+19
255 traj.propelled_ascent.states:r_3 c -1.000000E+19 7.644391E-05 1.000000E+19
256 traj.propelled_ascent.states:r_4 c -1.000000E+19 1.389788E-04 1.000000E+19
257 traj.propelled_ascent.states:r_5 c -1.000000E+19 1.625912E-04 1.000000E+19
258 traj.propelled_ascent.states:r_6 c -1.000000E+19 2.235462E-04 1.000000E+19
259 traj.propelled_ascent.states:r_7 c -1.000000E+19 3.233054E-04 1.000000E+19
260 traj.propelled_ascent.states:r_8 c -1.000000E+19 3.586422E-04 1.000000E+19
261 traj.propelled_ascent.states:r_9 c -1.000000E+19 4.463509E-04 1.000000E+19
262 traj.propelled_ascent.states:r_10 c -1.000000E+19 5.828386E-04 1.000000E+19
263 traj.propelled_ascent.states:r_11 c -1.000000E+19 6.297614E-04 1.000000E+19
264 traj.propelled_ascent.states:r_12 c -1.000000E+19 7.439434E-04 1.000000E+19
265 traj.propelled_ascent.states:r_13 c -1.000000E+19 9.168562E-04 1.000000E+19
266 traj.propelled_ascent.states:r_14 c -1.000000E+19 9.752894E-04 1.000000E+19
267 traj.propelled_ascent.states:r_15 c -1.000000E+19 1.115809E-03 1.000000E+19
268 traj.propelled_ascent.states:r_16 c -1.000000E+19 1.325043E-03 1.000000E+19
269 traj.propelled_ascent.states:r_17 c -1.000000E+19 1.394974E-03 1.000000E+19
270 traj.propelled_ascent.states:r_18 c -1.000000E+19 1.561838E-03 1.000000E+19
271 traj.propelled_ascent.states:r_19 c -1.000000E+19 1.807488E-03 1.000000E+19
272 traj.propelled_ascent.states:r_20 c -1.000000E+19 1.888967E-03 1.000000E+19
273 traj.propelled_ascent.states:r_21 c -1.000000E+19 2.082327E-03 1.000000E+19
274 traj.propelled_ascent.states:r_22 c -1.000000E+19 2.364686E-03 1.000000E+19
275 traj.propelled_ascent.states:r_23 c -1.000000E+19 2.457826E-03 1.000000E+19
276 traj.propelled_ascent.states:r_24 c -1.000000E+19 2.677980E-03 1.000000E+19
277 traj.propelled_ascent.states:r_25 c -1.000000E+19 2.997545E-03 1.000000E+19
278 traj.propelled_ascent.states:r_26 c -1.000000E+19 3.102525E-03 1.000000E+19
279 traj.propelled_ascent.states:r_27 c -1.000000E+19 3.349923E-03 1.000000E+19
280 traj.propelled_ascent.states:r_28 c -1.000000E+19 3.707410E-03 1.000000E+19
281 traj.propelled_ascent.states:r_29 c -1.000000E+19 3.824480E-03 1.000000E+19
282 traj.propelled_ascent.states:r_30 c -1.000000E+19 4.099737E-03 1.000000E+19
283 traj.propelled_ascent.states:r_31 c -1.000000E+19 4.496099E-03 1.000000E+19
284 traj.propelled_ascent.states:r_32 c -1.000000E+19 4.625587E-03 1.000000E+19
285 traj.propelled_ascent.states:r_33 c -1.000000E+19 4.929503E-03 1.000000E+19
286 traj.propelled_ascent.states:r_34 c -1.000000E+19 5.365962E-03 1.000000E+19
287 traj.propelled_ascent.states:r_35 c -1.000000E+19 5.508285E-03 1.000000E+19
288 traj.propelled_ascent.states:r_36 c -1.000000E+19 5.841872E-03 1.000000E+19
289 traj.propelled_ascent.states:r_37 c -1.000000E+19 6.319963E-03 1.000000E+19
290 traj.propelled_ascent.states:r_38 c -1.000000E+19 6.475641E-03 1.000000E+19
291 traj.propelled_ascent.states:r_39 c -1.000000E+19 6.840162E-03 1.000000E+19
292 traj.propelled_ascent.states:r_40 c -1.000000E+19 7.361794E-03 1.000000E+19
293 traj.propelled_ascent.states:r_41 c -1.000000E+19 7.531476E-03 1.000000E+19
294 traj.propelled_ascent.states:r_42 c -1.000000E+19 7.928497E-03 1.000000E+19
295 traj.propelled_ascent.states:r_43 c -1.000000E+19 8.496043E-03 1.000000E+19
296 traj.propelled_ascent.states:r_44 c -1.000000E+19 8.680534E-03 1.000000E+19
297 traj.propelled_ascent.states:r_45 c -1.000000E+19 9.112006E-03 1.000000E+19
298 traj.propelled_ascent.states:r_46 c -1.000000E+19 9.728424E-03 1.000000E+19
299 traj.propelled_ascent.states:r_47 c -1.000000E+19 9.928729E-03 1.000000E+19
300 traj.propelled_ascent.states:r_48 c -1.000000E+19 1.039710E-02 1.000000E+19
301 traj.propelled_ascent.states:r_49 c -1.000000E+19 1.106612E-02 1.000000E+19
302 traj.propelled_ascent.states:r_50 c -1.000000E+19 1.128351E-02 1.000000E+19
303 traj.propelled_ascent.states:r_51 c -1.000000E+19 1.179189E-02 1.000000E+19
304 traj.propelled_ascent.states:r_52 c -1.000000E+19 1.251828E-02 1.000000E+19
305 traj.propelled_ascent.states:r_53 c -1.000000E+19 1.275441E-02 1.000000E+19
306 traj.propelled_ascent.states:r_54 c -1.000000E+19 1.330682E-02 1.000000E+19
307 traj.propelled_ascent.states:r_55 c -1.000000E+19 1.409686E-02 1.000000E+19
308 traj.propelled_ascent.states:r_56 c -1.000000E+19 1.435389E-02 1.000000E+19
309 traj.propelled_ascent.states:r_57 c -1.000000E+19 1.495572E-02 1.000000E+19
310 traj.propelled_ascent.states:r_58 c -1.000000E+19 1.581790E-02 1.000000E+19
311 traj.propelled_ascent.states:r_59 c -1.000000E+19 1.609883E-02 1.000000E+19
312 traj.propelled_ascent.states:h_0 c -1.000000E+20 5.955631E-05 1.000000E+20
313 traj.propelled_ascent.states:h_1 c -1.000000E+20 2.889796E-04 1.000000E+20
314 traj.propelled_ascent.states:h_2 c -1.000000E+20 3.970521E-04 1.000000E+20
315 traj.propelled_ascent.states:h_3 c -1.000000E+20 7.028056E-04 1.000000E+20
316 traj.propelled_ascent.states:h_4 c -1.000000E+20 1.261242E-03 1.000000E+20
317 traj.propelled_ascent.states:h_5 c -1.000000E+20 1.470426E-03 1.000000E+20
318 traj.propelled_ascent.states:h_6 c -1.000000E+20 2.007407E-03 1.000000E+20
319 traj.propelled_ascent.states:h_7 c -1.000000E+20 2.879226E-03 1.000000E+20
320 traj.propelled_ascent.states:h_8 c -1.000000E+20 3.186408E-03 1.000000E+20
321 traj.propelled_ascent.states:h_9 c -1.000000E+20 3.945910E-03 1.000000E+20
322 traj.propelled_ascent.states:h_10 c -1.000000E+20 5.120959E-03 1.000000E+20
323 traj.propelled_ascent.states:h_11 c -1.000000E+20 5.523314E-03 1.000000E+20
324 traj.propelled_ascent.states:h_12 c -1.000000E+20 6.499486E-03 1.000000E+20
325 traj.propelled_ascent.states:h_13 c -1.000000E+20 7.971004E-03 1.000000E+20
326 traj.propelled_ascent.states:h_14 c -1.000000E+20 8.466679E-03 1.000000E+20
327 traj.propelled_ascent.states:h_15 c -1.000000E+20 9.655772E-03 1.000000E+20
328 traj.propelled_ascent.states:h_16 c -1.000000E+20 1.141963E-02 1.000000E+20
329 traj.propelled_ascent.states:h_17 c -1.000000E+20 1.200757E-02 1.000000E+20
330 traj.propelled_ascent.states:h_18 c -1.000000E+20 1.340756E-02 1.000000E+20
331 traj.propelled_ascent.states:h_19 c -1.000000E+20 1.546189E-02 1.000000E+20
332 traj.propelled_ascent.states:h_20 c -1.000000E+20 1.614170E-02 1.000000E+20
333 traj.propelled_ascent.states:h_21 c -1.000000E+20 1.775210E-02 1.000000E+20
334 traj.propelled_ascent.states:h_22 c -1.000000E+20 2.009707E-02 1.000000E+20
335 traj.propelled_ascent.states:h_23 c -1.000000E+20 2.086901E-02 1.000000E+20
336 traj.propelled_ascent.states:h_24 c -1.000000E+20 2.269076E-02 1.000000E+20
337 traj.propelled_ascent.states:h_25 c -1.000000E+20 2.532851E-02 1.000000E+20
338 traj.propelled_ascent.states:h_26 c -1.000000E+20 2.619345E-02 1.000000E+20
339 traj.propelled_ascent.states:h_27 c -1.000000E+20 2.822892E-02 1.000000E+20
340 traj.propelled_ascent.states:h_28 c -1.000000E+20 3.116354E-02 1.000000E+20
341 traj.propelled_ascent.states:h_29 c -1.000000E+20 3.212299E-02 1.000000E+20
342 traj.propelled_ascent.states:h_30 c -1.000000E+20 3.437601E-02 1.000000E+20
343 traj.propelled_ascent.states:h_31 c -1.000000E+20 3.761369E-02 1.000000E+20
344 traj.propelled_ascent.states:h_32 c -1.000000E+20 3.866983E-02 1.000000E+20
345 traj.propelled_ascent.states:h_33 c -1.000000E+20 4.114581E-02 1.000000E+20
346 traj.propelled_ascent.states:h_34 c -1.000000E+20 4.469500E-02 1.000000E+20
347 traj.propelled_ascent.states:h_35 c -1.000000E+20 4.585077E-02 1.000000E+20
348 traj.propelled_ascent.states:h_36 c -1.000000E+20 4.855687E-02 1.000000E+20
349 traj.propelled_ascent.states:h_37 c -1.000000E+20 5.242864E-02 1.000000E+20
350 traj.propelled_ascent.states:h_38 c -1.000000E+20 5.368781E-02 1.000000E+20
351 traj.propelled_ascent.states:h_39 c -1.000000E+20 5.663329E-02 1.000000E+20
352 traj.propelled_ascent.states:h_40 c -1.000000E+20 6.084173E-02 1.000000E+20
353 traj.propelled_ascent.states:h_41 c -1.000000E+20 6.220912E-02 1.000000E+20
354 traj.propelled_ascent.states:h_42 c -1.000000E+20 6.540567E-02 1.000000E+20
355 traj.propelled_ascent.states:h_43 c -1.000000E+20 6.996861E-02 1.000000E+20
356 traj.propelled_ascent.states:h_44 c -1.000000E+20 7.145029E-02 1.000000E+20
357 traj.propelled_ascent.states:h_45 c -1.000000E+20 7.491269E-02 1.000000E+20
358 traj.propelled_ascent.states:h_46 c -1.000000E+20 7.985264E-02 1.000000E+20
359 traj.propelled_ascent.states:h_47 c -1.000000E+20 8.145630E-02 1.000000E+20
360 traj.propelled_ascent.states:h_48 c -1.000000E+20 8.520325E-02 1.000000E+20
361 traj.propelled_ascent.states:h_49 c -1.000000E+20 9.054882E-02 1.000000E+20
362 traj.propelled_ascent.states:h_50 c -1.000000E+20 9.228427E-02 1.000000E+20
363 traj.propelled_ascent.states:h_51 c -1.000000E+20 9.633973E-02 1.000000E+20
364 traj.propelled_ascent.states:h_52 c -1.000000E+20 1.021278E-01 1.000000E+20
365 traj.propelled_ascent.states:h_53 c -1.000000E+20 1.040078E-01 1.000000E+20
366 traj.propelled_ascent.states:h_54 c -1.000000E+20 1.084030E-01 1.000000E+20
367 traj.propelled_ascent.states:h_55 c -1.000000E+20 1.146823E-01 1.000000E+20
368 traj.propelled_ascent.states:h_56 c -1.000000E+20 1.167237E-01 1.000000E+20
369 traj.propelled_ascent.states:h_57 c -1.000000E+20 1.215006E-01 1.000000E+20
370 traj.propelled_ascent.states:h_58 c -1.000000E+20 1.283373E-01 1.000000E+20
371 traj.propelled_ascent.states:h_59 c -1.000000E+20 1.305634E-01 1.000000E+20
372 traj.propelled_ascent.states:gam_0 c 0.000000E+00 5.202544E-01 9.444444E-01
373 traj.propelled_ascent.states:gam_1 c 0.000000E+00 4.763626E-01 9.444444E-01
374 traj.propelled_ascent.states:gam_2 c 0.000000E+00 4.770257E-01 9.444444E-01
375 traj.propelled_ascent.states:gam_3 c 0.000000E+00 4.704726E-01 9.444444E-01
376 traj.propelled_ascent.states:gam_4 c 0.000000E+00 4.663584E-01 9.444444E-01
377 traj.propelled_ascent.states:gam_5 c 0.000000E+00 4.621277E-01 9.444444E-01
378 traj.propelled_ascent.states:gam_6 c 0.000000E+00 4.609797E-01 9.444444E-01
379 traj.propelled_ascent.states:gam_7 c 0.000000E+00 4.586634E-01 9.444444E-01
380 traj.propelled_ascent.states:gam_8 c 0.000000E+00 4.559549E-01 9.444444E-01
381 traj.propelled_ascent.states:gam_9 c 0.000000E+00 4.551840E-01 9.444444E-01
382 traj.propelled_ascent.states:gam_10 c 0.000000E+00 4.535527E-01 9.444444E-01
383 traj.propelled_ascent.states:gam_11 c 0.000000E+00 4.515478E-01 9.444444E-01
384 traj.propelled_ascent.states:gam_12 c 0.000000E+00 4.509615E-01 9.444444E-01
385 traj.propelled_ascent.states:gam_13 c 0.000000E+00 4.496955E-01 9.444444E-01
386 traj.propelled_ascent.states:gam_14 c 0.000000E+00 4.480977E-01 9.444444E-01
387 traj.propelled_ascent.states:gam_15 c 0.000000E+00 4.476229E-01 9.444444E-01
388 traj.propelled_ascent.states:gam_16 c 0.000000E+00 4.465855E-01 9.444444E-01
389 traj.propelled_ascent.states:gam_17 c 0.000000E+00 4.452548E-01 9.444444E-01
390 traj.propelled_ascent.states:gam_18 c 0.000000E+00 4.448552E-01 9.444444E-01
391 traj.propelled_ascent.states:gam_19 c 0.000000E+00 4.439754E-01 9.444444E-01
392 traj.propelled_ascent.states:gam_20 c 0.000000E+00 4.428346E-01 9.444444E-01
393 traj.propelled_ascent.states:gam_21 c 0.000000E+00 4.424895E-01 9.444444E-01
394 traj.propelled_ascent.states:gam_22 c 0.000000E+00 4.417257E-01 9.444444E-01
395 traj.propelled_ascent.states:gam_23 c 0.000000E+00 4.407276E-01 9.444444E-01
396 traj.propelled_ascent.states:gam_24 c 0.000000E+00 4.404241E-01 9.444444E-01
397 traj.propelled_ascent.states:gam_25 c 0.000000E+00 4.397497E-01 9.444444E-01
398 traj.propelled_ascent.states:gam_26 c 0.000000E+00 4.388635E-01 9.444444E-01
399 traj.propelled_ascent.states:gam_27 c 0.000000E+00 4.385929E-01 9.444444E-01
400 traj.propelled_ascent.states:gam_28 c 0.000000E+00 4.379900E-01 9.444444E-01
401 traj.propelled_ascent.states:gam_29 c 0.000000E+00 4.371943E-01 9.444444E-01
402 traj.propelled_ascent.states:gam_30 c 0.000000E+00 4.369506E-01 9.444444E-01
403 traj.propelled_ascent.states:gam_31 c 0.000000E+00 4.364065E-01 9.444444E-01
404 traj.propelled_ascent.states:gam_32 c 0.000000E+00 4.356860E-01 9.444444E-01
405 traj.propelled_ascent.states:gam_33 c 0.000000E+00 4.354648E-01 9.444444E-01
406 traj.propelled_ascent.states:gam_34 c 0.000000E+00 4.349702E-01 9.444444E-01
407 traj.propelled_ascent.states:gam_35 c 0.000000E+00 4.343135E-01 9.444444E-01
408 traj.propelled_ascent.states:gam_36 c 0.000000E+00 4.341116E-01 9.444444E-01
409 traj.propelled_ascent.states:gam_37 c 0.000000E+00 4.336595E-01 9.444444E-01
410 traj.propelled_ascent.states:gam_38 c 0.000000E+00 4.330580E-01 9.444444E-01
411 traj.propelled_ascent.states:gam_39 c 0.000000E+00 4.328728E-01 9.444444E-01
412 traj.propelled_ascent.states:gam_40 c 0.000000E+00 4.324578E-01 9.444444E-01
413 traj.propelled_ascent.states:gam_41 c 0.000000E+00 4.319049E-01 9.444444E-01
414 traj.propelled_ascent.states:gam_42 c 0.000000E+00 4.317345E-01 9.444444E-01
415 traj.propelled_ascent.states:gam_43 c 0.000000E+00 4.313523E-01 9.444444E-01
416 traj.propelled_ascent.states:gam_44 c 0.000000E+00 4.308427E-01 9.444444E-01
417 traj.propelled_ascent.states:gam_45 c 0.000000E+00 4.306856E-01 9.444444E-01
418 traj.propelled_ascent.states:gam_46 c 0.000000E+00 4.303330E-01 9.444444E-01
419 traj.propelled_ascent.states:gam_47 c 0.000000E+00 4.298627E-01 9.444444E-01
420 traj.propelled_ascent.states:gam_48 c 0.000000E+00 4.297176E-01 9.444444E-01
421 traj.propelled_ascent.states:gam_49 c 0.000000E+00 4.293920E-01 9.444444E-01
422 traj.propelled_ascent.states:gam_50 c 0.000000E+00 4.289577E-01 9.444444E-01
423 traj.propelled_ascent.states:gam_51 c 0.000000E+00 4.288237E-01 9.444444E-01
424 traj.propelled_ascent.states:gam_52 c 0.000000E+00 4.285231E-01 9.444444E-01
425 traj.propelled_ascent.states:gam_53 c 0.000000E+00 4.281221E-01 9.444444E-01
426 traj.propelled_ascent.states:gam_54 c 0.000000E+00 4.279985E-01 9.444444E-01
427 traj.propelled_ascent.states:gam_55 c 0.000000E+00 4.277213E-01 9.444444E-01
428 traj.propelled_ascent.states:gam_56 c 0.000000E+00 4.273520E-01 9.444444E-01
429 traj.propelled_ascent.states:gam_57 c 0.000000E+00 4.272382E-01 9.444444E-01
430 traj.propelled_ascent.states:gam_58 c 0.000000E+00 4.269833E-01 9.444444E-01
431 traj.propelled_ascent.states:gam_59 c 0.000000E+00 4.266442E-01 9.444444E-01
432 traj.propelled_ascent.states:gam_60 c 0.000000E+00 4.265399E-01 9.444444E-01
433 traj.propelled_ascent.states:v_0 c 1.000000E-04 7.672107E-03 1.000000E+19
434 traj.propelled_ascent.states:v_1 c 1.000000E-04 1.672431E-02 1.000000E+19
435 traj.propelled_ascent.states:v_2 c 1.000000E-04 1.955302E-02 1.000000E+19
436 traj.propelled_ascent.states:v_3 c 1.000000E-04 2.597644E-02 1.000000E+19
437 traj.propelled_ascent.states:v_4 c 1.000000E-04 3.472261E-02 1.000000E+19
438 traj.propelled_ascent.states:v_5 c 1.000000E-04 3.746552E-02 1.000000E+19
439 traj.propelled_ascent.states:v_6 c 1.000000E-04 4.370553E-02 1.000000E+19
440 traj.propelled_ascent.states:v_7 c 1.000000E-04 5.223242E-02 1.000000E+19
441 traj.propelled_ascent.states:v_8 c 1.000000E-04 5.491356E-02 1.000000E+19
442 traj.propelled_ascent.states:v_9 c 1.000000E-04 6.102522E-02 1.000000E+19
443 traj.propelled_ascent.states:v_10 c 1.000000E-04 6.940334E-02 1.000000E+19
444 traj.propelled_ascent.states:v_11 c 1.000000E-04 7.204388E-02 1.000000E+19
445 traj.propelled_ascent.states:v_12 c 1.000000E-04 7.807383E-02 1.000000E+19
446 traj.propelled_ascent.states:v_13 c 1.000000E-04 8.636392E-02 1.000000E+19
447 traj.propelled_ascent.states:v_14 c 1.000000E-04 8.898233E-02 1.000000E+19
448 traj.propelled_ascent.states:v_15 c 1.000000E-04 9.497173E-02 1.000000E+19
449 traj.propelled_ascent.states:v_16 c 1.000000E-04 1.032283E-01 1.000000E+19
450 traj.propelled_ascent.states:v_17 c 1.000000E-04 1.058414E-01 1.000000E+19
451 traj.propelled_ascent.states:v_18 c 1.000000E-04 1.118281E-01 1.000000E+19
452 traj.propelled_ascent.states:v_19 c 1.000000E-04 1.201024E-01 1.000000E+19
453 traj.propelled_ascent.states:v_20 c 1.000000E-04 1.227261E-01 1.000000E+19
454 traj.propelled_ascent.states:v_21 c 1.000000E-04 1.287465E-01 1.000000E+19
455 traj.propelled_ascent.states:v_22 c 1.000000E-04 1.370882E-01 1.000000E+19
456 traj.propelled_ascent.states:v_23 c 1.000000E-04 1.397385E-01 1.000000E+19
457 traj.propelled_ascent.states:v_24 c 1.000000E-04 1.458289E-01 1.000000E+19
458 traj.propelled_ascent.states:v_25 c 1.000000E-04 1.542889E-01 1.000000E+19
459 traj.propelled_ascent.states:v_26 c 1.000000E-04 1.569819E-01 1.000000E+19
460 traj.propelled_ascent.states:v_27 c 1.000000E-04 1.631801E-01 1.000000E+19
461 traj.propelled_ascent.states:v_28 c 1.000000E-04 1.718120E-01 1.000000E+19
462 traj.propelled_ascent.states:v_29 c 1.000000E-04 1.745653E-01 1.000000E+19
463 traj.propelled_ascent.states:v_30 c 1.000000E-04 1.809121E-01 1.000000E+19
464 traj.propelled_ascent.states:v_31 c 1.000000E-04 1.897748E-01 1.000000E+19
465 traj.propelled_ascent.states:v_32 c 1.000000E-04 1.926075E-01 1.000000E+19
466 traj.propelled_ascent.states:v_33 c 1.000000E-04 1.991486E-01 1.000000E+19
467 traj.propelled_ascent.states:v_34 c 1.000000E-04 2.083088E-01 1.000000E+19
468 traj.propelled_ascent.states:v_35 c 1.000000E-04 2.112431E-01 1.000000E+19
469 traj.propelled_ascent.states:v_36 c 1.000000E-04 2.180312E-01 1.000000E+19
470 traj.propelled_ascent.states:v_37 c 1.000000E-04 2.275671E-01 1.000000E+19
471 traj.propelled_ascent.states:v_38 c 1.000000E-04 2.306292E-01 1.000000E+19
472 traj.propelled_ascent.states:v_39 c 1.000000E-04 2.377271E-01 1.000000E+19
473 traj.propelled_ascent.states:v_40 c 1.000000E-04 2.477329E-01 1.000000E+19
474 traj.propelled_ascent.states:v_41 c 1.000000E-04 2.509547E-01 1.000000E+19
475 traj.propelled_ascent.states:v_42 c 1.000000E-04 2.584398E-01 1.000000E+19
476 traj.propelled_ascent.states:v_43 c 1.000000E-04 2.690329E-01 1.000000E+19
477 traj.propelled_ascent.states:v_44 c 1.000000E-04 2.724545E-01 1.000000E+19
478 traj.propelled_ascent.states:v_45 c 1.000000E-04 2.804248E-01 1.000000E+19
479 traj.propelled_ascent.states:v_46 c 1.000000E-04 2.917565E-01 1.000000E+19
480 traj.propelled_ascent.states:v_47 c 1.000000E-04 2.954301E-01 1.000000E+19
481 traj.propelled_ascent.states:v_48 c 1.000000E-04 3.040140E-01 1.000000E+19
482 traj.propelled_ascent.states:v_49 c 1.000000E-04 3.162854E-01 1.000000E+19
483 traj.propelled_ascent.states:v_50 c 1.000000E-04 3.202813E-01 1.000000E+19
484 traj.propelled_ascent.states:v_51 c 1.000000E-04 3.296537E-01 1.000000E+19
485 traj.propelled_ascent.states:v_52 c 1.000000E-04 3.431436E-01 1.000000E+19
486 traj.propelled_ascent.states:v_53 c 1.000000E-04 3.475604E-01 1.000000E+19
487 traj.propelled_ascent.states:v_54 c 1.000000E-04 3.579701E-01 1.000000E+19
488 traj.propelled_ascent.states:v_55 c 1.000000E-04 3.730835E-01 1.000000E+19
489 traj.propelled_ascent.states:v_56 c 1.000000E-04 3.780671E-01 1.000000E+19
490 traj.propelled_ascent.states:v_57 c 1.000000E-04 3.898870E-01 1.000000E+19
491 traj.propelled_ascent.states:v_58 c 1.000000E-04 4.072489E-01 1.000000E+19
492 traj.propelled_ascent.states:v_59 c 1.000000E-04 4.130291E-01 1.000000E+19
493 traj.propelled_ascent.states:p_0 c 1.020000E+00 6.305226E+00 1.000000E+21
494 traj.propelled_ascent.states:p_1 c 1.020000E+00 6.058536E+00 1.000000E+21
495 traj.propelled_ascent.states:p_2 c 1.020000E+00 5.985224E+00 1.000000E+21
496 traj.propelled_ascent.states:p_3 c 1.020000E+00 5.825217E+00 1.000000E+21
497 traj.propelled_ascent.states:p_4 c 1.020000E+00 5.620757E+00 1.000000E+21
498 traj.propelled_ascent.states:p_5 c 1.020000E+00 5.559612E+00 1.000000E+21
499 traj.propelled_ascent.states:p_6 c 1.020000E+00 5.425540E+00 1.000000E+21
500 traj.propelled_ascent.states:p_7 c 1.020000E+00 5.252980E+00 1.000000E+21
501 traj.propelled_ascent.states:p_8 c 1.020000E+00 5.201111E+00 1.000000E+21
502 traj.propelled_ascent.states:p_9 c 1.020000E+00 5.086938E+00 1.000000E+21
503 traj.propelled_ascent.states:p_10 c 1.020000E+00 4.939114E+00 1.000000E+21
504 traj.propelled_ascent.states:p_11 c 1.020000E+00 4.894489E+00 1.000000E+21
505 traj.propelled_ascent.states:p_12 c 1.020000E+00 4.795947E+00 1.000000E+21
506 traj.propelled_ascent.states:p_13 c 1.020000E+00 4.667720E+00 1.000000E+21
507 traj.propelled_ascent.states:p_14 c 1.020000E+00 4.628871E+00 1.000000E+21
508 traj.propelled_ascent.states:p_15 c 1.020000E+00 4.542850E+00 1.000000E+21
509 traj.propelled_ascent.states:p_16 c 1.020000E+00 4.430435E+00 1.000000E+21
510 traj.propelled_ascent.states:p_17 c 1.020000E+00 4.396270E+00 1.000000E+21
511 traj.propelled_ascent.states:p_18 c 1.020000E+00 4.320446E+00 1.000000E+21
512 traj.propelled_ascent.states:p_19 c 1.020000E+00 4.220990E+00 1.000000E+21
513 traj.propelled_ascent.states:p_20 c 1.020000E+00 4.190682E+00 1.000000E+21
514 traj.propelled_ascent.states:p_21 c 1.020000E+00 4.123281E+00 1.000000E+21
515 traj.propelled_ascent.states:p_22 c 1.020000E+00 4.034589E+00 1.000000E+21
516 traj.propelled_ascent.states:p_23 c 1.020000E+00 4.007498E+00 1.000000E+21
517 traj.propelled_ascent.states:p_24 c 1.020000E+00 3.947142E+00 1.000000E+21
518 traj.propelled_ascent.states:p_25 c 1.020000E+00 3.867497E+00 1.000000E+21
519 traj.propelled_ascent.states:p_26 c 1.020000E+00 3.843118E+00 1.000000E+21
520 traj.propelled_ascent.states:p_27 c 1.020000E+00 3.788721E+00 1.000000E+21
521 traj.propelled_ascent.states:p_28 c 1.020000E+00 3.716757E+00 1.000000E+21
522 traj.propelled_ascent.states:p_29 c 1.020000E+00 3.694690E+00 1.000000E+21
523 traj.propelled_ascent.states:p_30 c 1.020000E+00 3.645379E+00 1.000000E+21
524 traj.propelled_ascent.states:p_31 c 1.020000E+00 3.580000E+00 1.000000E+21
525 traj.propelled_ascent.states:p_32 c 1.020000E+00 3.559918E+00 1.000000E+21
526 traj.propelled_ascent.states:p_33 c 1.020000E+00 3.514988E+00 1.000000E+21
527 traj.propelled_ascent.states:p_34 c 1.020000E+00 3.455298E+00 1.000000E+21
528 traj.propelled_ascent.states:p_35 c 1.020000E+00 3.436936E+00 1.000000E+21
529 traj.propelled_ascent.states:p_36 c 1.020000E+00 3.395809E+00 1.000000E+21
530 traj.propelled_ascent.states:p_37 c 1.020000E+00 3.341071E+00 1.000000E+21
531 traj.propelled_ascent.states:p_38 c 1.020000E+00 3.324210E+00 1.000000E+21
532 traj.propelled_ascent.states:p_39 c 1.020000E+00 3.286405E+00 1.000000E+21
533 traj.propelled_ascent.states:p_40 c 1.020000E+00 3.236007E+00 1.000000E+21
534 traj.propelled_ascent.states:p_41 c 1.020000E+00 3.220464E+00 1.000000E+21
535 traj.propelled_ascent.states:p_42 c 1.020000E+00 3.185581E+00 1.000000E+21
536 traj.propelled_ascent.states:p_43 c 1.020000E+00 3.139009E+00 1.000000E+21
537 traj.propelled_ascent.states:p_44 c 1.020000E+00 3.124630E+00 1.000000E+21
538 traj.propelled_ascent.states:p_45 c 1.020000E+00 3.092332E+00 1.000000E+21
539 traj.propelled_ascent.states:p_46 c 1.020000E+00 3.049151E+00 1.000000E+21
540 traj.propelled_ascent.states:p_47 c 1.020000E+00 3.035806E+00 1.000000E+21
541 traj.propelled_ascent.states:p_48 c 1.020000E+00 3.005807E+00 1.000000E+21
542 traj.propelled_ascent.states:p_49 c 1.020000E+00 2.965648E+00 1.000000E+21
543 traj.propelled_ascent.states:p_50 c 1.020000E+00 2.953225E+00 1.000000E+21
544 traj.propelled_ascent.states:p_51 c 1.020000E+00 2.925280E+00 1.000000E+21
545 traj.propelled_ascent.states:p_52 c 1.020000E+00 2.887826E+00 1.000000E+21
546 traj.propelled_ascent.states:p_53 c 1.020000E+00 2.876230E+00 1.000000E+21
547 traj.propelled_ascent.states:p_54 c 1.020000E+00 2.850127E+00 1.000000E+21
548 traj.propelled_ascent.states:p_55 c 1.020000E+00 2.815106E+00 1.000000E+21
549 traj.propelled_ascent.states:p_56 c 1.020000E+00 2.804255E+00 1.000000E+21
550 traj.propelled_ascent.states:p_57 c 1.020000E+00 2.779812E+00 1.000000E+21
551 traj.propelled_ascent.states:p_58 c 1.020000E+00 2.746987E+00 1.000000E+21
552 traj.propelled_ascent.states:p_59 c 1.020000E+00 2.736808E+00 1.000000E+21
553 traj.propelled_ascent.states:V_w_0 c 0.000000E+00 1.027314E-01 1.000000E+20
554 traj.propelled_ascent.states:V_w_1 c 0.000000E+00 1.002340E-01 1.000000E+20
555 traj.propelled_ascent.states:V_w_2 c 0.000000E+00 9.685978E-02 1.000000E+20
556 traj.propelled_ascent.states:V_w_3 c 0.000000E+00 9.580827E-02 1.000000E+20
557 traj.propelled_ascent.states:V_w_4 c 0.000000E+00 9.342884E-02 1.000000E+20
558 traj.propelled_ascent.states:V_w_5 c 0.000000E+00 9.020771E-02 1.000000E+20
559 traj.propelled_ascent.states:V_w_6 c 0.000000E+00 8.920254E-02 1.000000E+20
560 traj.propelled_ascent.states:V_w_7 c 0.000000E+00 8.692563E-02 1.000000E+20
561 traj.propelled_ascent.states:V_w_8 c 0.000000E+00 8.383843E-02 1.000000E+20
562 traj.propelled_ascent.states:V_w_9 c 0.000000E+00 8.287395E-02 1.000000E+20
563 traj.propelled_ascent.states:V_w_10 c 0.000000E+00 8.068740E-02 1.000000E+20
564 traj.propelled_ascent.states:V_w_11 c 0.000000E+00 7.771881E-02 1.000000E+20
565 traj.propelled_ascent.states:V_w_12 c 0.000000E+00 7.679051E-02 1.000000E+20
566 traj.propelled_ascent.states:V_w_13 c 0.000000E+00 7.468451E-02 1.000000E+20
567 traj.propelled_ascent.states:V_w_14 c 0.000000E+00 7.182212E-02 1.000000E+20
568 traj.propelled_ascent.states:V_w_15 c 0.000000E+00 7.092632E-02 1.000000E+20
569 traj.propelled_ascent.states:V_w_16 c 0.000000E+00 6.889281E-02 1.000000E+20
570 traj.propelled_ascent.states:V_w_17 c 0.000000E+00 6.612636E-02 1.000000E+20
571 traj.propelled_ascent.states:V_w_18 c 0.000000E+00 6.525999E-02 1.000000E+20
572 traj.propelled_ascent.states:V_w_19 c 0.000000E+00 6.329228E-02 1.000000E+20
573 traj.propelled_ascent.states:V_w_20 c 0.000000E+00 6.061316E-02 1.000000E+20
574 traj.propelled_ascent.states:V_w_21 c 0.000000E+00 5.977365E-02 1.000000E+20
575 traj.propelled_ascent.states:V_w_22 c 0.000000E+00 5.786608E-02 1.000000E+20
576 traj.propelled_ascent.states:V_w_23 c 0.000000E+00 5.526699E-02 1.000000E+20
577 traj.propelled_ascent.states:V_w_24 c 0.000000E+00 5.445213E-02 1.000000E+20
578 traj.propelled_ascent.states:V_w_25 c 0.000000E+00 5.259986E-02 1.000000E+20
579 traj.propelled_ascent.states:V_w_26 c 0.000000E+00 5.007455E-02 1.000000E+20
580 traj.propelled_ascent.states:V_w_27 c 0.000000E+00 4.928246E-02 1.000000E+20
581 traj.propelled_ascent.states:V_w_28 c 0.000000E+00 4.748132E-02 1.000000E+20
582 traj.propelled_ascent.states:V_w_29 c 0.000000E+00 4.502435E-02 1.000000E+20
583 traj.propelled_ascent.states:V_w_30 c 0.000000E+00 4.425338E-02 1.000000E+20
584 traj.propelled_ascent.states:V_w_31 c 0.000000E+00 4.249974E-02 1.000000E+20
585 traj.propelled_ascent.states:V_w_32 c 0.000000E+00 4.010638E-02 1.000000E+20
586 traj.propelled_ascent.states:V_w_33 c 0.000000E+00 3.935510E-02 1.000000E+20
587 traj.propelled_ascent.states:V_w_34 c 0.000000E+00 3.764576E-02 1.000000E+20
588 traj.propelled_ascent.states:V_w_35 c 0.000000E+00 3.531184E-02 1.000000E+20
589 traj.propelled_ascent.states:V_w_36 c 0.000000E+00 3.457898E-02 1.000000E+20
590 traj.propelled_ascent.states:V_w_37 c 0.000000E+00 3.291114E-02 1.000000E+20
591 traj.propelled_ascent.states:V_w_38 c 0.000000E+00 3.063296E-02 1.000000E+20
592 traj.propelled_ascent.states:V_w_39 c 0.000000E+00 2.991740E-02 1.000000E+20
593 traj.propelled_ascent.states:V_w_40 c 0.000000E+00 2.828854E-02 1.000000E+20
594 traj.propelled_ascent.states:V_w_41 c 0.000000E+00 2.606282E-02 1.000000E+20
595 traj.propelled_ascent.states:V_w_42 c 0.000000E+00 2.536354E-02 1.000000E+20
596 traj.propelled_ascent.states:V_w_43 c 0.000000E+00 2.377144E-02 1.000000E+20
597 traj.propelled_ascent.states:V_w_44 c 0.000000E+00 2.159522E-02 1.000000E+20
598 traj.propelled_ascent.states:V_w_45 c 0.000000E+00 2.091133E-02 1.000000E+20
599 traj.propelled_ascent.states:V_w_46 c 0.000000E+00 1.935397E-02 1.000000E+20
600 traj.propelled_ascent.states:V_w_47 c 0.000000E+00 1.722459E-02 1.000000E+20
601 traj.propelled_ascent.states:V_w_48 c 0.000000E+00 1.655528E-02 1.000000E+20
602 traj.propelled_ascent.states:V_w_49 c 0.000000E+00 1.503084E-02 1.000000E+20
603 traj.propelled_ascent.states:V_w_50 c 0.000000E+00 1.294591E-02 1.000000E+20
604 traj.propelled_ascent.states:V_w_51 c 0.000000E+00 1.229042E-02 1.000000E+20
605 traj.propelled_ascent.states:V_w_52 c 0.000000E+00 1.079726E-02 1.000000E+20
606 traj.propelled_ascent.states:V_w_53 c 0.000000E+00 8.754589E-03 1.000000E+20
607 traj.propelled_ascent.states:V_w_54 c 0.000000E+00 8.112271E-03 1.000000E+20
608 traj.propelled_ascent.states:V_w_55 c 0.000000E+00 6.648883E-03 1.000000E+20
609 traj.propelled_ascent.states:V_w_56 c 0.000000E+00 4.646480E-03 1.000000E+20
610 traj.propelled_ascent.states:V_w_57 c 0.000000E+00 4.016715E-03 1.000000E+20
611 traj.propelled_ascent.states:V_w_58 c 0.000000E+00 2.581733E-03 1.000000E+20
612 traj.propelled_ascent.states:V_w_59 c 0.000000E+00 6.177737E-04 1.000000E+20
Constraints (i - inequality, e - equality)
Index Name Type Lower Value Upper Status Lagrange Multiplier (N/A)
0 traj.linkages.propelled_ascent:time_final|ballistic_ascent:time_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
1 traj.linkages.propelled_ascent:r_final|ballistic_ascent:r_initial e 0.000000E+00 -2.220446E-16 0.000000E+00 9.00000E+100
2 traj.linkages.propelled_ascent:h_final|ballistic_ascent:h_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
3 traj.linkages.propelled_ascent:gam_final|ballistic_ascent:gam_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
4 traj.linkages.propelled_ascent:v_final|ballistic_ascent:v_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
5 traj.linkages.ballistic_ascent:time_final|descent:time_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
6 traj.linkages.ballistic_ascent:r_final|descent:r_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
7 traj.linkages.ballistic_ascent:h_final|descent:h_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
8 traj.linkages.ballistic_ascent:gam_final|descent:gam_initial e 0.000000E+00 4.203342E-32 0.000000E+00 9.00000E+100
9 traj.linkages.ballistic_ascent:v_final|descent:v_initial e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
10 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 7.537093E-15 0.000000E+00 9.00000E+100
11 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 7.879688E-15 0.000000E+00 9.00000E+100
12 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 5.824117E-15 0.000000E+00 9.00000E+100
13 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 4.111141E-15 0.000000E+00 9.00000E+100
14 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 8.222283E-15 0.000000E+00 9.00000E+100
15 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 8.222283E-15 0.000000E+00 9.00000E+100
16 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 9.592663E-15 0.000000E+00 9.00000E+100
17 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 4.111141E-15 0.000000E+00 9.00000E+100
18 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 1.027785E-14 0.000000E+00 9.00000E+100
19 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 2.432425E-14 0.000000E+00 9.00000E+100
20 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.851902E-15 0.000000E+00 9.00000E+100
21 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 8.222283E-15 0.000000E+00 9.00000E+100
22 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 5.138927E-15 0.000000E+00 9.00000E+100
23 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 3.083356E-15 0.000000E+00 9.00000E+100
24 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 5.481522E-15 0.000000E+00 9.00000E+100
25 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 1.370380E-14 0.000000E+00 9.00000E+100
26 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 4.453736E-15 0.000000E+00 9.00000E+100
27 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -8.222283E-15 0.000000E+00 9.00000E+100
28 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.021311E-14 0.000000E+00 9.00000E+100
29 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.509307E-15 0.000000E+00 9.00000E+100
30 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 9.935258E-15 0.000000E+00 9.00000E+100
31 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 2.740761E-14 0.000000E+00 9.00000E+100
32 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -7.537093E-15 0.000000E+00 9.00000E+100
33 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.851902E-16 0.000000E+00 9.00000E+100
34 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 6.509307E-15 0.000000E+00 9.00000E+100
35 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.370380E-15 0.000000E+00 9.00000E+100
36 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 1.062045E-14 0.000000E+00 9.00000E+100
37 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.432425E-14 0.000000E+00 9.00000E+100
38 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 3.425951E-15 0.000000E+00 9.00000E+100
39 traj.ballistic_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.027785E-15 0.000000E+00 9.00000E+100
40 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 3.425951E-16 0.000000E+00 9.00000E+100
41 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.370380E-15 0.000000E+00 9.00000E+100
42 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
43 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.851902E-16 0.000000E+00 9.00000E+100
44 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
45 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 3.425951E-15 0.000000E+00 9.00000E+100
46 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 6.851902E-16 0.000000E+00 9.00000E+100
47 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 1.712976E-15 0.000000E+00 9.00000E+100
48 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 3.425951E-16 0.000000E+00 9.00000E+100
49 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.096304E-14 0.000000E+00 9.00000E+100
50 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.370380E-15 0.000000E+00 9.00000E+100
51 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 1.712976E-15 0.000000E+00 9.00000E+100
52 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.425951E-15 0.000000E+00 9.00000E+100
53 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.027785E-15 0.000000E+00 9.00000E+100
54 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 5.310224E-15 0.000000E+00 9.00000E+100
55 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.124090E-14 0.000000E+00 9.00000E+100
56 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 3.083356E-15 0.000000E+00 9.00000E+100
57 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 1.524548E-14 0.000000E+00 9.00000E+100
58 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.233342E-14 0.000000E+00 9.00000E+100
59 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 4.282439E-15 0.000000E+00 9.00000E+100
60 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 3.254654E-15 0.000000E+00 9.00000E+100
61 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 1.627327E-15 0.000000E+00 9.00000E+100
62 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.597249E-15 0.000000E+00 9.00000E+100
63 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -4.710683E-15 0.000000E+00 9.00000E+100
64 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -6.851902E-15 0.000000E+00 9.00000E+100
65 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -4.710683E-16 0.000000E+00 9.00000E+100
66 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 2.697937E-15 0.000000E+00 9.00000E+100
67 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 -4.453736E-15 0.000000E+00 9.00000E+100
68 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 8.372168E-15 0.000000E+00 9.00000E+100
69 traj.ballistic_ascent.collocation_constraint.defects:h e 0.000000E+00 9.689018E-16 0.000000E+00 9.00000E+100
70 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
71 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -4.377604E-17 0.000000E+00 9.00000E+100
72 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.417199E-16 0.000000E+00 9.00000E+100
73 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.522645E-16 0.000000E+00 9.00000E+100
74 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -5.709919E-18 0.000000E+00 9.00000E+100
75 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.235621E-17 0.000000E+00 9.00000E+100
76 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 9.706862E-17 0.000000E+00 9.00000E+100
77 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.045290E-17 0.000000E+00 9.00000E+100
78 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -5.900249E-17 0.000000E+00 9.00000E+100
79 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 7.422894E-17 0.000000E+00 9.00000E+100
80 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -8.374547E-17 0.000000E+00 9.00000E+100
81 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -6.851902E-17 0.000000E+00 9.00000E+100
82 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 4.948596E-17 0.000000E+00 9.00000E+100
83 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.806612E-18 0.000000E+00 9.00000E+100
84 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.522645E-17 0.000000E+00 9.00000E+100
85 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.540150E-16 0.000000E+00 9.00000E+100
86 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 5.329257E-17 0.000000E+00 9.00000E+100
87 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 4.187274E-17 0.000000E+00 9.00000E+100
88 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -6.471241E-17 0.000000E+00 9.00000E+100
89 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 5.329257E-17 0.000000E+00 9.00000E+100
90 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.425951E-17 0.000000E+00 9.00000E+100
91 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.903306E-17 0.000000E+00 9.00000E+100
92 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.903306E-17 0.000000E+00 9.00000E+100
93 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 4.948596E-17 0.000000E+00 9.00000E+100
94 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.806612E-17 0.000000E+00 9.00000E+100
95 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.806612E-17 0.000000E+00 9.00000E+100
96 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 5.329257E-17 0.000000E+00 9.00000E+100
97 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 6.090580E-17 0.000000E+00 9.00000E+100
98 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 4.567935E-17 0.000000E+00 9.00000E+100
99 traj.ballistic_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.045290E-17 0.000000E+00 9.00000E+100
100 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 2.637982E-14 0.000000E+00 9.00000E+100
101 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.425951E-16 0.000000E+00 9.00000E+100
102 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 2.912058E-14 0.000000E+00 9.00000E+100
103 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 3.768546E-15 0.000000E+00 9.00000E+100
104 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 1.507419E-14 0.000000E+00 9.00000E+100
105 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 2.398166E-15 0.000000E+00 9.00000E+100
106 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 3.768546E-15 0.000000E+00 9.00000E+100
107 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 3.768546E-15 0.000000E+00 9.00000E+100
108 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.055571E-15 0.000000E+00 9.00000E+100
109 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -8.050985E-15 0.000000E+00 9.00000E+100
110 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 6.851902E-16 0.000000E+00 9.00000E+100
111 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 5.481522E-15 0.000000E+00 9.00000E+100
112 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 8.393580E-15 0.000000E+00 9.00000E+100
113 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 4.111141E-15 0.000000E+00 9.00000E+100
114 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 1.404640E-14 0.000000E+00 9.00000E+100
115 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.310224E-15 0.000000E+00 9.00000E+100
116 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 4.967629E-15 0.000000E+00 9.00000E+100
117 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 1.267602E-14 0.000000E+00 9.00000E+100
118 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -6.338010E-15 0.000000E+00 9.00000E+100
119 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 3.254654E-15 0.000000E+00 9.00000E+100
120 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 2.997707E-15 0.000000E+00 9.00000E+100
121 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.012746E-14 0.000000E+00 9.00000E+100
122 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 9.507014E-15 0.000000E+00 9.00000E+100
123 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 5.738468E-15 0.000000E+00 9.00000E+100
124 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 1.481724E-14 0.000000E+00 9.00000E+100
125 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.138927E-16 0.000000E+00 9.00000E+100
126 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 2.655112E-15 0.000000E+00 9.00000E+100
127 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 5.395873E-15 0.000000E+00 9.00000E+100
128 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.027785E-15 0.000000E+00 9.00000E+100
129 traj.ballistic_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.997707E-16 0.000000E+00 9.00000E+100
130 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -2.108638E-14 0.000000E+00 9.00000E+100
131 traj.descent.collocation_constraint.defects:r e 0.000000E+00 1.323067E-14 0.000000E+00 9.00000E+100
132 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -5.374960E-15 0.000000E+00 9.00000E+100
133 traj.descent.collocation_constraint.defects:r e 0.000000E+00 3.886509E-14 0.000000E+00 9.00000E+100
134 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -4.548043E-15 0.000000E+00 9.00000E+100
135 traj.descent.collocation_constraint.defects:r e 0.000000E+00 2.480751E-14 0.000000E+00 9.00000E+100
136 traj.descent.collocation_constraint.defects:r e 0.000000E+00 1.653834E-14 0.000000E+00 9.00000E+100
137 traj.descent.collocation_constraint.defects:r e 0.000000E+00 4.961501E-15 0.000000E+00 9.00000E+100
138 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -2.480751E-15 0.000000E+00 9.00000E+100
139 traj.descent.collocation_constraint.defects:r e 0.000000E+00 8.682627E-15 0.000000E+00 9.00000E+100
140 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -9.923002E-15 0.000000E+00 9.00000E+100
141 traj.descent.collocation_constraint.defects:r e 0.000000E+00 1.364413E-14 0.000000E+00 9.00000E+100
142 traj.descent.collocation_constraint.defects:r e 0.000000E+00 2.067292E-15 0.000000E+00 9.00000E+100
143 traj.descent.collocation_constraint.defects:r e 0.000000E+00 1.860563E-14 0.000000E+00 9.00000E+100
144 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -3.452378E-14 0.000000E+00 9.00000E+100
145 traj.descent.collocation_constraint.defects:r e 0.000000E+00 2.480751E-15 0.000000E+00 9.00000E+100
146 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -2.212003E-14 0.000000E+00 9.00000E+100
147 traj.descent.collocation_constraint.defects:r e 0.000000E+00 3.100938E-14 0.000000E+00 9.00000E+100
148 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -3.121611E-14 0.000000E+00 9.00000E+100
149 traj.descent.collocation_constraint.defects:r e 0.000000E+00 2.005273E-14 0.000000E+00 9.00000E+100
150 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -1.385086E-14 0.000000E+00 9.00000E+100
151 traj.descent.collocation_constraint.defects:r e 0.000000E+00 5.147558E-14 0.000000E+00 9.00000E+100
152 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -1.819217E-14 0.000000E+00 9.00000E+100
153 traj.descent.collocation_constraint.defects:r e 0.000000E+00 3.721126E-15 0.000000E+00 9.00000E+100
154 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -1.488450E-14 0.000000E+00 9.00000E+100
155 traj.descent.collocation_constraint.defects:r e 0.000000E+00 2.025946E-14 0.000000E+00 9.00000E+100
156 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -6.636008E-14 0.000000E+00 9.00000E+100
157 traj.descent.collocation_constraint.defects:r e 0.000000E+00 2.811517E-14 0.000000E+00 9.00000E+100
158 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -1.509123E-14 0.000000E+00 9.00000E+100
159 traj.descent.collocation_constraint.defects:r e 0.000000E+00 -1.033646E-15 0.000000E+00 9.00000E+100
160 traj.descent.collocation_constraint.defects:h e 0.000000E+00 1.643528E-33 0.000000E+00 9.00000E+100
161 traj.descent.collocation_constraint.defects:h e 0.000000E+00 1.015557E-14 0.000000E+00 9.00000E+100
162 traj.descent.collocation_constraint.defects:h e 0.000000E+00 5.581689E-15 0.000000E+00 9.00000E+100
163 traj.descent.collocation_constraint.defects:h e 0.000000E+00 8.630945E-15 0.000000E+00 9.00000E+100
164 traj.descent.collocation_constraint.defects:h e 0.000000E+00 5.530007E-15 0.000000E+00 9.00000E+100
165 traj.descent.collocation_constraint.defects:h e 0.000000E+00 1.757198E-15 0.000000E+00 9.00000E+100
166 traj.descent.collocation_constraint.defects:h e 0.000000E+00 -4.548043E-15 0.000000E+00 9.00000E+100
167 traj.descent.collocation_constraint.defects:h e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
168 traj.descent.collocation_constraint.defects:h e 0.000000E+00 -1.137011E-15 0.000000E+00 9.00000E+100
169 traj.descent.collocation_constraint.defects:h e 0.000000E+00 2.749499E-14 0.000000E+00 9.00000E+100
170 traj.descent.collocation_constraint.defects:h e 0.000000E+00 -2.067292E-15 0.000000E+00 9.00000E+100
171 traj.descent.collocation_constraint.defects:h e 0.000000E+00 7.235523E-15 0.000000E+00 9.00000E+100
172 traj.descent.collocation_constraint.defects:h e 0.000000E+00 5.168230E-15 0.000000E+00 9.00000E+100
173 traj.descent.collocation_constraint.defects:h e 0.000000E+00 4.548043E-15 0.000000E+00 9.00000E+100
174 traj.descent.collocation_constraint.defects:h e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
175 traj.descent.collocation_constraint.defects:h e 0.000000E+00 -1.777871E-14 0.000000E+00 9.00000E+100
176 traj.descent.collocation_constraint.defects:h e 0.000000E+00 6.201877E-16 0.000000E+00 9.00000E+100
177 traj.descent.collocation_constraint.defects:h e 0.000000E+00 2.274021E-15 0.000000E+00 9.00000E+100
178 traj.descent.collocation_constraint.defects:h e 0.000000E+00 -9.716273E-15 0.000000E+00 9.00000E+100
179 traj.descent.collocation_constraint.defects:h e 0.000000E+00 8.889356E-15 0.000000E+00 9.00000E+100
180 traj.descent.collocation_constraint.defects:h e 0.000000E+00 1.860563E-15 0.000000E+00 9.00000E+100
181 traj.descent.collocation_constraint.defects:h e 0.000000E+00 5.788418E-15 0.000000E+00 9.00000E+100
182 traj.descent.collocation_constraint.defects:h e 0.000000E+00 2.894209E-15 0.000000E+00 9.00000E+100
183 traj.descent.collocation_constraint.defects:h e 0.000000E+00 6.201877E-15 0.000000E+00 9.00000E+100
184 traj.descent.collocation_constraint.defects:h e 0.000000E+00 4.134584E-15 0.000000E+00 9.00000E+100
185 traj.descent.collocation_constraint.defects:h e 0.000000E+00 1.860563E-15 0.000000E+00 9.00000E+100
186 traj.descent.collocation_constraint.defects:h e 0.000000E+00 2.894209E-15 0.000000E+00 9.00000E+100
187 traj.descent.collocation_constraint.defects:h e 0.000000E+00 2.480751E-15 0.000000E+00 9.00000E+100
188 traj.descent.collocation_constraint.defects:h e 0.000000E+00 2.894209E-15 0.000000E+00 9.00000E+100
189 traj.descent.collocation_constraint.defects:h e 0.000000E+00 3.721126E-15 0.000000E+00 9.00000E+100
190 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 1.653834E-15 0.000000E+00 9.00000E+100
191 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 2.894209E-15 0.000000E+00 9.00000E+100
192 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
193 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 7.855710E-15 0.000000E+00 9.00000E+100
194 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 1.653834E-15 0.000000E+00 9.00000E+100
195 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 8.269169E-16 0.000000E+00 9.00000E+100
196 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 1.199029E-14 0.000000E+00 9.00000E+100
197 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 4.134584E-16 0.000000E+00 9.00000E+100
198 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 -8.269169E-15 0.000000E+00 9.00000E+100
199 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 4.548043E-15 0.000000E+00 9.00000E+100
200 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 -8.269169E-15 0.000000E+00 9.00000E+100
201 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 -4.134584E-15 0.000000E+00 9.00000E+100
202 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 1.984600E-14 0.000000E+00 9.00000E+100
203 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 4.134584E-16 0.000000E+00 9.00000E+100
204 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 4.134584E-16 0.000000E+00 9.00000E+100
205 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 -2.935555E-14 0.000000E+00 9.00000E+100
206 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 3.721126E-15 0.000000E+00 9.00000E+100
207 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 8.269169E-16 0.000000E+00 9.00000E+100
208 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 1.157684E-14 0.000000E+00 9.00000E+100
209 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 -6.201877E-15 0.000000E+00 9.00000E+100
210 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 4.961501E-15 0.000000E+00 9.00000E+100
211 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 1.157684E-14 0.000000E+00 9.00000E+100
212 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 -1.653834E-14 0.000000E+00 9.00000E+100
213 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 -4.961501E-15 0.000000E+00 9.00000E+100
214 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 -2.480751E-15 0.000000E+00 9.00000E+100
215 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 4.961501E-15 0.000000E+00 9.00000E+100
216 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 -1.033646E-14 0.000000E+00 9.00000E+100
217 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 -7.442252E-15 0.000000E+00 9.00000E+100
218 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 1.426432E-14 0.000000E+00 9.00000E+100
219 traj.descent.collocation_constraint.defects:gam e 0.000000E+00 8.889356E-15 0.000000E+00 9.00000E+100
220 traj.descent.collocation_constraint.defects:v e 0.000000E+00 9.251132E-15 0.000000E+00 9.00000E+100
221 traj.descent.collocation_constraint.defects:v e 0.000000E+00 2.429068E-15 0.000000E+00 9.00000E+100
222 traj.descent.collocation_constraint.defects:v e 0.000000E+00 4.909819E-15 0.000000E+00 9.00000E+100
223 traj.descent.collocation_constraint.defects:v e 0.000000E+00 8.269169E-15 0.000000E+00 9.00000E+100
224 traj.descent.collocation_constraint.defects:v e 0.000000E+00 -9.302815E-15 0.000000E+00 9.00000E+100
225 traj.descent.collocation_constraint.defects:v e 0.000000E+00 -5.685053E-16 0.000000E+00 9.00000E+100
226 traj.descent.collocation_constraint.defects:v e 0.000000E+00 1.834722E-15 0.000000E+00 9.00000E+100
227 traj.descent.collocation_constraint.defects:v e 0.000000E+00 2.480751E-15 0.000000E+00 9.00000E+100
228 traj.descent.collocation_constraint.defects:v e 0.000000E+00 5.885322E-15 0.000000E+00 9.00000E+100
229 traj.descent.collocation_constraint.defects:v e 0.000000E+00 2.060832E-15 0.000000E+00 9.00000E+100
230 traj.descent.collocation_constraint.defects:v e 0.000000E+00 -3.746967E-16 0.000000E+00 9.00000E+100
231 traj.descent.collocation_constraint.defects:v e 0.000000E+00 -2.299863E-15 0.000000E+00 9.00000E+100
232 traj.descent.collocation_constraint.defects:v e 0.000000E+00 4.057061E-15 0.000000E+00 9.00000E+100
233 traj.descent.collocation_constraint.defects:v e 0.000000E+00 5.426642E-15 0.000000E+00 9.00000E+100
234 traj.descent.collocation_constraint.defects:v e 0.000000E+00 5.943465E-16 0.000000E+00 9.00000E+100
235 traj.descent.collocation_constraint.defects:v e 0.000000E+00 -3.695285E-15 0.000000E+00 9.00000E+100
236 traj.descent.collocation_constraint.defects:v e 0.000000E+00 -1.705516E-15 0.000000E+00 9.00000E+100
237 traj.descent.collocation_constraint.defects:v e 0.000000E+00 2.997574E-15 0.000000E+00 9.00000E+100
238 traj.descent.collocation_constraint.defects:v e 0.000000E+00 1.488450E-14 0.000000E+00 9.00000E+100
239 traj.descent.collocation_constraint.defects:v e 0.000000E+00 -9.457862E-15 0.000000E+00 9.00000E+100
240 traj.descent.collocation_constraint.defects:v e 0.000000E+00 -7.235523E-16 0.000000E+00 9.00000E+100
241 traj.descent.collocation_constraint.defects:v e 0.000000E+00 7.700663E-15 0.000000E+00 9.00000E+100
242 traj.descent.collocation_constraint.defects:v e 0.000000E+00 2.584115E-16 0.000000E+00 9.00000E+100
243 traj.descent.collocation_constraint.defects:v e 0.000000E+00 -4.858137E-15 0.000000E+00 9.00000E+100
244 traj.descent.collocation_constraint.defects:v e 0.000000E+00 9.147768E-15 0.000000E+00 9.00000E+100
245 traj.descent.collocation_constraint.defects:v e 0.000000E+00 -3.721126E-15 0.000000E+00 9.00000E+100
246 traj.descent.collocation_constraint.defects:v e 0.000000E+00 1.374749E-14 0.000000E+00 9.00000E+100
247 traj.descent.collocation_constraint.defects:v e 0.000000E+00 -1.085328E-15 0.000000E+00 9.00000E+100
248 traj.descent.collocation_constraint.defects:v e 0.000000E+00 3.049256E-15 0.000000E+00 9.00000E+100
249 traj.descent.collocation_constraint.defects:v e 0.000000E+00 9.871320E-15 0.000000E+00 9.00000E+100
250 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.134281E-20 0.000000E+00 9.00000E+100
251 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.877430E-20 0.000000E+00 9.00000E+100
252 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.258101E-21 0.000000E+00 9.00000E+100
253 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.001296E-19 0.000000E+00 9.00000E+100
254 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -7.509721E-20 0.000000E+00 9.00000E+100
255 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.006481E-20 0.000000E+00 9.00000E+100
256 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.504536E-19 0.000000E+00 9.00000E+100
257 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.752268E-19 0.000000E+00 9.00000E+100
258 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.752268E-19 0.000000E+00 9.00000E+100
259 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.504536E-19 0.000000E+00 9.00000E+100
260 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.503240E-20 0.000000E+00 9.00000E+100
261 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
262 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -9.011665E-19 0.000000E+00 9.00000E+100
263 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.254212E-19 0.000000E+00 9.00000E+100
264 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 2.753564E-19 0.000000E+00 9.00000E+100
265 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.677171E-18 0.000000E+00 9.00000E+100
266 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.254212E-19 0.000000E+00 9.00000E+100
267 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 6.007777E-19 0.000000E+00 9.00000E+100
268 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -7.009073E-19 0.000000E+00 9.00000E+100
269 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
270 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.501944E-19 0.000000E+00 9.00000E+100
271 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.251620E-18 0.000000E+00 9.00000E+100
272 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.007777E-19 0.000000E+00 9.00000E+100
273 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 8.511017E-19 0.000000E+00 9.00000E+100
274 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.504536E-19 0.000000E+00 9.00000E+100
275 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -4.505833E-19 0.000000E+00 9.00000E+100
276 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.001296E-18 0.000000E+00 9.00000E+100
277 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 8.010369E-19 0.000000E+00 9.00000E+100
278 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.006481E-19 0.000000E+00 9.00000E+100
279 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 4.005185E-19 0.000000E+00 9.00000E+100
280 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -9.512313E-19 0.000000E+00 9.00000E+100
281 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.151491E-18 0.000000E+00 9.00000E+100
282 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.202852E-18 0.000000E+00 9.00000E+100
283 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -4.756157E-18 0.000000E+00 9.00000E+100
284 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.501944E-19 0.000000E+00 9.00000E+100
285 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.702203E-18 0.000000E+00 9.00000E+100
286 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 7.009073E-18 0.000000E+00 9.00000E+100
287 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -4.005185E-19 0.000000E+00 9.00000E+100
288 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.702203E-18 0.000000E+00 9.00000E+100
289 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -9.011665E-19 0.000000E+00 9.00000E+100
290 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.602074E-18 0.000000E+00 9.00000E+100
291 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.001296E-18 0.000000E+00 9.00000E+100
292 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.001296E-19 0.000000E+00 9.00000E+100
293 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.301685E-18 0.000000E+00 9.00000E+100
294 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.003888E-19 0.000000E+00 9.00000E+100
295 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 5.006481E-19 0.000000E+00 9.00000E+100
296 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -9.011665E-19 0.000000E+00 9.00000E+100
297 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.002592E-19 0.000000E+00 9.00000E+100
298 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.907647E-18 0.000000E+00 9.00000E+100
299 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.802333E-18 0.000000E+00 9.00000E+100
300 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.902463E-18 0.000000E+00 9.00000E+100
301 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.181529E-17 0.000000E+00 9.00000E+100
302 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -3.003888E-19 0.000000E+00 9.00000E+100
303 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -6.007777E-19 0.000000E+00 9.00000E+100
304 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 3.204148E-18 0.000000E+00 9.00000E+100
305 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -2.603370E-18 0.000000E+00 9.00000E+100
306 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.071387E-17 0.000000E+00 9.00000E+100
307 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -1.011309E-17 0.000000E+00 9.00000E+100
308 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 1.802333E-18 0.000000E+00 9.00000E+100
309 traj.propelled_ascent.collocation_constraint.defects:r e 0.000000E+00 -5.006481E-19 0.000000E+00 9.00000E+100
310 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.642751E-19 0.000000E+00 9.00000E+100
311 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.816145E-19 0.000000E+00 9.00000E+100
312 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 5.006481E-19 0.000000E+00 9.00000E+100
313 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.063877E-18 0.000000E+00 9.00000E+100
314 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 5.006481E-19 0.000000E+00 9.00000E+100
315 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 8.761341E-19 0.000000E+00 9.00000E+100
316 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.878726E-18 0.000000E+00 9.00000E+100
317 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.001296E-18 0.000000E+00 9.00000E+100
318 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.754861E-19 0.000000E+00 9.00000E+100
319 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 4.505833E-18 0.000000E+00 9.00000E+100
320 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.877430E-18 0.000000E+00 9.00000E+100
321 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.252916E-18 0.000000E+00 9.00000E+100
322 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 7.760045E-18 0.000000E+00 9.00000E+100
323 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
324 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 6.758749E-18 0.000000E+00 9.00000E+100
325 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -3.754861E-18 0.000000E+00 9.00000E+100
326 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 7.009073E-18 0.000000E+00 9.00000E+100
327 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.251620E-18 0.000000E+00 9.00000E+100
328 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 9.261989E-18 0.000000E+00 9.00000E+100
329 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
330 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.003888E-18 0.000000E+00 9.00000E+100
331 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.503240E-18 0.000000E+00 9.00000E+100
332 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 5.006481E-19 0.000000E+00 9.00000E+100
333 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 7.009073E-18 0.000000E+00 9.00000E+100
334 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 9.011665E-18 0.000000E+00 9.00000E+100
335 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 6.007777E-18 0.000000E+00 9.00000E+100
336 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.501944E-18 0.000000E+00 9.00000E+100
337 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.503240E-18 0.000000E+00 9.00000E+100
338 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 9.512313E-18 0.000000E+00 9.00000E+100
339 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.401815E-17 0.000000E+00 9.00000E+100
340 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.401815E-17 0.000000E+00 9.00000E+100
341 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.852398E-17 0.000000E+00 9.00000E+100
342 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.251620E-17 0.000000E+00 9.00000E+100
343 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.552009E-17 0.000000E+00 9.00000E+100
344 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.451879E-17 0.000000E+00 9.00000E+100
345 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.001296E-18 0.000000E+00 9.00000E+100
346 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.301685E-17 0.000000E+00 9.00000E+100
347 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.852398E-17 0.000000E+00 9.00000E+100
348 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 2.553305E-17 0.000000E+00 9.00000E+100
349 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.301685E-17 0.000000E+00 9.00000E+100
350 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 5.507129E-18 0.000000E+00 9.00000E+100
351 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.251620E-17 0.000000E+00 9.00000E+100
352 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 9.512313E-18 0.000000E+00 9.00000E+100
353 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 8.010369E-18 0.000000E+00 9.00000E+100
354 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.201555E-17 0.000000E+00 9.00000E+100
355 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.101426E-17 0.000000E+00 9.00000E+100
356 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.101426E-17 0.000000E+00 9.00000E+100
357 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.702203E-17 0.000000E+00 9.00000E+100
358 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.102722E-17 0.000000E+00 9.00000E+100
359 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -1.001296E-18 0.000000E+00 9.00000E+100
360 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.504536E-17 0.000000E+00 9.00000E+100
361 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -9.111795E-17 0.000000E+00 9.00000E+100
362 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.604666E-17 0.000000E+00 9.00000E+100
363 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -2.202852E-17 0.000000E+00 9.00000E+100
364 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 -8.010369E-18 0.000000E+00 9.00000E+100
365 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 4.906351E-17 0.000000E+00 9.00000E+100
366 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 1.501944E-17 0.000000E+00 9.00000E+100
367 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.304277E-17 0.000000E+00 9.00000E+100
368 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 3.204148E-17 0.000000E+00 9.00000E+100
369 traj.propelled_ascent.collocation_constraint.defects:h e 0.000000E+00 5.406999E-17 0.000000E+00 9.00000E+100
370 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.424066E-17 0.000000E+00 9.00000E+100
371 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.204148E-16 0.000000E+00 9.00000E+100
372 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.925081E-16 0.000000E+00 9.00000E+100
373 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 7.209332E-17 0.000000E+00 9.00000E+100
374 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.225103E-17 0.000000E+00 9.00000E+100
375 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.244199E-16 0.000000E+00 9.00000E+100
376 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.247354E-16 0.000000E+00 9.00000E+100
377 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.695528E-16 0.000000E+00 9.00000E+100
378 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.112551E-17 0.000000E+00 9.00000E+100
379 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -6.221387E-16 0.000000E+00 9.00000E+100
380 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.803629E-17 0.000000E+00 9.00000E+100
381 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -7.921365E-17 0.000000E+00 9.00000E+100
382 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.286109E-16 0.000000E+00 9.00000E+100
383 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -8.232879E-18 0.000000E+00 9.00000E+100
384 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.388464E-16 0.000000E+00 9.00000E+100
385 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.315403E-17 0.000000E+00 9.00000E+100
386 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -6.408295E-17 0.000000E+00 9.00000E+100
387 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.651026E-16 0.000000E+00 9.00000E+100
388 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 8.878159E-17 0.000000E+00 9.00000E+100
389 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.639901E-16 0.000000E+00 9.00000E+100
390 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.762281E-16 0.000000E+00 9.00000E+100
391 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -3.066191E-16 0.000000E+00 9.00000E+100
392 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 6.764312E-17 0.000000E+00 9.00000E+100
393 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.352862E-16 0.000000E+00 9.00000E+100
394 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.976075E-16 0.000000E+00 9.00000E+100
395 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.580935E-16 0.000000E+00 9.00000E+100
396 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -8.477641E-17 0.000000E+00 9.00000E+100
397 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.689036E-16 0.000000E+00 9.00000E+100
398 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.140365E-16 0.000000E+00 9.00000E+100
399 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.745593E-16 0.000000E+00 9.00000E+100
400 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.474130E-16 0.000000E+00 9.00000E+100
401 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.221581E-16 0.000000E+00 9.00000E+100
402 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 8.377511E-17 0.000000E+00 9.00000E+100
403 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.169475E-17 0.000000E+00 9.00000E+100
404 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 8.789155E-18 0.000000E+00 9.00000E+100
405 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.457442E-17 0.000000E+00 9.00000E+100
406 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -5.939911E-16 0.000000E+00 9.00000E+100
407 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.391802E-16 0.000000E+00 9.00000E+100
408 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 7.665478E-17 0.000000E+00 9.00000E+100
409 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.279434E-16 0.000000E+00 9.00000E+100
410 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.935839E-17 0.000000E+00 9.00000E+100
411 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.480989E-17 0.000000E+00 9.00000E+100
412 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.759127E-16 0.000000E+00 9.00000E+100
413 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.112551E-19 0.000000E+00 9.00000E+100
414 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.615424E-16 0.000000E+00 9.00000E+100
415 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 3.434446E-16 0.000000E+00 9.00000E+100
416 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.667898E-16 0.000000E+00 9.00000E+100
417 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.099757E-16 0.000000E+00 9.00000E+100
418 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.514366E-17 0.000000E+00 9.00000E+100
419 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.873536E-16 0.000000E+00 9.00000E+100
420 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.644907E-16 0.000000E+00 9.00000E+100
421 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 1.741143E-17 0.000000E+00 9.00000E+100
422 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -2.664560E-17 0.000000E+00 9.00000E+100
423 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -1.796770E-17 0.000000E+00 9.00000E+100
424 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -4.229364E-16 0.000000E+00 9.00000E+100
425 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 5.206740E-17 0.000000E+00 9.00000E+100
426 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 6.786563E-18 0.000000E+00 9.00000E+100
427 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 9.284240E-17 0.000000E+00 9.00000E+100
428 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 2.553305E-17 0.000000E+00 9.00000E+100
429 traj.propelled_ascent.collocation_constraint.defects:gam e 0.000000E+00 -5.323558E-17 0.000000E+00 9.00000E+100
430 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -9.612443E-18 0.000000E+00 9.00000E+100
431 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.806221E-18 0.000000E+00 9.00000E+100
432 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -8.010369E-18 0.000000E+00 9.00000E+100
433 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.762281E-17 0.000000E+00 9.00000E+100
434 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.204148E-18 0.000000E+00 9.00000E+100
435 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.806221E-18 0.000000E+00 9.00000E+100
436 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 9.612443E-18 0.000000E+00 9.00000E+100
437 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
438 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.806221E-18 0.000000E+00 9.00000E+100
439 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.204148E-17 0.000000E+00 9.00000E+100
440 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.602074E-17 0.000000E+00 9.00000E+100
441 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 8.010369E-18 0.000000E+00 9.00000E+100
442 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 7.850162E-17 0.000000E+00 9.00000E+100
443 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.563318E-17 0.000000E+00 9.00000E+100
444 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.364355E-17 0.000000E+00 9.00000E+100
445 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.204148E-18 0.000000E+00 9.00000E+100
446 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.082696E-17 0.000000E+00 9.00000E+100
447 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.121452E-17 0.000000E+00 9.00000E+100
448 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 7.049125E-17 0.000000E+00 9.00000E+100
449 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.043940E-17 0.000000E+00 9.00000E+100
450 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.325599E-17 0.000000E+00 9.00000E+100
451 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.521970E-16 0.000000E+00 9.00000E+100
452 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.485807E-17 0.000000E+00 9.00000E+100
453 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.524562E-17 0.000000E+00 9.00000E+100
454 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -6.888917E-17 0.000000E+00 9.00000E+100
455 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -8.010369E-18 0.000000E+00 9.00000E+100
456 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 8.490991E-17 0.000000E+00 9.00000E+100
457 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.842385E-16 0.000000E+00 9.00000E+100
458 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.684770E-17 0.000000E+00 9.00000E+100
459 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -8.811406E-17 0.000000E+00 9.00000E+100
460 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -9.452236E-17 0.000000E+00 9.00000E+100
461 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.485807E-17 0.000000E+00 9.00000E+100
462 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.922489E-17 0.000000E+00 9.00000E+100
463 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -3.524562E-17 0.000000E+00 9.00000E+100
464 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -6.408295E-18 0.000000E+00 9.00000E+100
465 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.922489E-17 0.000000E+00 9.00000E+100
466 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.524562E-17 0.000000E+00 9.00000E+100
467 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -9.452236E-17 0.000000E+00 9.00000E+100
468 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 1.121452E-16 0.000000E+00 9.00000E+100
469 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -8.971613E-17 0.000000E+00 9.00000E+100
470 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -7.689954E-17 0.000000E+00 9.00000E+100
471 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.883733E-17 0.000000E+00 9.00000E+100
472 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.130758E-16 0.000000E+00 9.00000E+100
473 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 3.364355E-17 0.000000E+00 9.00000E+100
474 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.607258E-17 0.000000E+00 9.00000E+100
475 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 9.932858E-17 0.000000E+00 9.00000E+100
476 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 6.408295E-18 0.000000E+00 9.00000E+100
477 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.403111E-17 0.000000E+00 9.00000E+100
478 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.441866E-16 0.000000E+00 9.00000E+100
479 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 7.209332E-17 0.000000E+00 9.00000E+100
480 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -5.607258E-17 0.000000E+00 9.00000E+100
481 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 4.085288E-16 0.000000E+00 9.00000E+100
482 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -1.602074E-16 0.000000E+00 9.00000E+100
483 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 2.339028E-16 0.000000E+00 9.00000E+100
484 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -2.082696E-16 0.000000E+00 9.00000E+100
485 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.806221E-17 0.000000E+00 9.00000E+100
486 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -4.485807E-17 0.000000E+00 9.00000E+100
487 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 9.612443E-17 0.000000E+00 9.00000E+100
488 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -8.651199E-17 0.000000E+00 9.00000E+100
489 traj.propelled_ascent.collocation_constraint.defects:v e 0.000000E+00 -9.292028E-17 0.000000E+00 9.00000E+100
490 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.143633E-09 0.000000E+00 9.00000E+100
491 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.990606E-09 0.000000E+00 9.00000E+100
492 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.792825E-09 0.000000E+00 9.00000E+100
493 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.733707E-09 0.000000E+00 9.00000E+100
494 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.604772E-09 0.000000E+00 9.00000E+100
495 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.441410E-09 0.000000E+00 9.00000E+100
496 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.393111E-09 0.000000E+00 9.00000E+100
497 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.288419E-09 0.000000E+00 9.00000E+100
498 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.156732E-09 0.000000E+00 9.00000E+100
499 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.117953E-09 0.000000E+00 9.00000E+100
500 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.034063E-09 0.000000E+00 9.00000E+100
501 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -9.287664E-10 0.000000E+00 9.00000E+100
502 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -8.977854E-10 0.000000E+00 9.00000E+100
503 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -8.307813E-10 0.000000E+00 9.00000E+100
504 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -7.466587E-10 0.000000E+00 9.00000E+100
505 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -7.219000E-10 0.000000E+00 9.00000E+100
506 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -6.683037E-10 0.000000E+00 9.00000E+100
507 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -6.009295E-10 0.000000E+00 9.00000E+100
508 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.810733E-10 0.000000E+00 9.00000E+100
509 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.380579E-10 0.000000E+00 9.00000E+100
510 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.838840E-10 0.000000E+00 9.00000E+100
511 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.678935E-10 0.000000E+00 9.00000E+100
512 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.332124E-10 0.000000E+00 9.00000E+100
513 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.894477E-10 0.000000E+00 9.00000E+100
514 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.765086E-10 0.000000E+00 9.00000E+100
515 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.484148E-10 0.000000E+00 9.00000E+100
516 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.128934E-10 0.000000E+00 9.00000E+100
517 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.023734E-10 0.000000E+00 9.00000E+100
518 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.795096E-10 0.000000E+00 9.00000E+100
519 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.505425E-10 0.000000E+00 9.00000E+100
520 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.419511E-10 0.000000E+00 9.00000E+100
521 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.232586E-10 0.000000E+00 9.00000E+100
522 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.995328E-10 0.000000E+00 9.00000E+100
523 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.924905E-10 0.000000E+00 9.00000E+100
524 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.771422E-10 0.000000E+00 9.00000E+100
525 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.576330E-10 0.000000E+00 9.00000E+100
526 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.518324E-10 0.000000E+00 9.00000E+100
527 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.391891E-10 0.000000E+00 9.00000E+100
528 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.230888E-10 0.000000E+00 9.00000E+100
529 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.183003E-10 0.000000E+00 9.00000E+100
530 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.078444E-10 0.000000E+00 9.00000E+100
531 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -9.451713E-11 0.000000E+00 9.00000E+100
532 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -9.054899E-11 0.000000E+00 9.00000E+100
533 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -8.187785E-11 0.000000E+00 9.00000E+100
534 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -7.081756E-11 0.000000E+00 9.00000E+100
535 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -6.751831E-11 0.000000E+00 9.00000E+100
536 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -6.031535E-11 0.000000E+00 9.00000E+100
537 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.111400E-11 0.000000E+00 9.00000E+100
538 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 1.819106E-09 0.000000E+00 9.00000E+100
539 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -4.250381E-11 0.000000E+00 9.00000E+100
540 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.511373E-11 0.000000E+00 9.00000E+100
541 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -3.284703E-11 0.000000E+00 9.00000E+100
542 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.797325E-11 0.000000E+00 9.00000E+100
543 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -2.174317E-11 0.000000E+00 9.00000E+100
544 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.988219E-11 0.000000E+00 9.00000E+100
545 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.581256E-11 0.000000E+00 9.00000E+100
546 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.060597E-11 0.000000E+00 9.00000E+100
547 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -9.052538E-12 0.000000E+00 9.00000E+100
548 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -5.649508E-12 0.000000E+00 9.00000E+100
549 traj.propelled_ascent.collocation_constraint.defects:p e 0.000000E+00 -1.298896E-12 0.000000E+00 9.00000E+100
550 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.401815E-15 0.000000E+00 9.00000E+100
551 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.752268E-15 0.000000E+00 9.00000E+100
552 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.154083E-15 0.000000E+00 9.00000E+100
553 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 7.059138E-15 0.000000E+00 9.00000E+100
554 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 2.653435E-15 0.000000E+00 9.00000E+100
555 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.706092E-15 0.000000E+00 9.00000E+100
556 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.507129E-15 0.000000E+00 9.00000E+100
557 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.802333E-15 0.000000E+00 9.00000E+100
558 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -5.957712E-15 0.000000E+00 9.00000E+100
559 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.104018E-15 0.000000E+00 9.00000E+100
560 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.802333E-15 0.000000E+00 9.00000E+100
561 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.652139E-15 0.000000E+00 9.00000E+100
562 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 5.657323E-15 0.000000E+00 9.00000E+100
563 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.955120E-15 0.000000E+00 9.00000E+100
564 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.002592E-15 0.000000E+00 9.00000E+100
565 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 8.010369E-16 0.000000E+00 9.00000E+100
566 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.451879E-15 0.000000E+00 9.00000E+100
567 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.504536E-16 0.000000E+00 9.00000E+100
568 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.001296E-15 0.000000E+00 9.00000E+100
569 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.002592E-16 0.000000E+00 9.00000E+100
570 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.005185E-16 0.000000E+00 9.00000E+100
571 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.202852E-15 0.000000E+00 9.00000E+100
572 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.007777E-16 0.000000E+00 9.00000E+100
573 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.905055E-15 0.000000E+00 9.00000E+100
574 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.151491E-15 0.000000E+00 9.00000E+100
575 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.802333E-15 0.000000E+00 9.00000E+100
576 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.903759E-15 0.000000E+00 9.00000E+100
577 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.702203E-15 0.000000E+00 9.00000E+100
578 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.501944E-16 0.000000E+00 9.00000E+100
579 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.503240E-16 0.000000E+00 9.00000E+100
580 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.301685E-15 0.000000E+00 9.00000E+100
581 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.007777E-16 0.000000E+00 9.00000E+100
582 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 9.011665E-16 0.000000E+00 9.00000E+100
583 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -3.104018E-15 0.000000E+00 9.00000E+100
584 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -7.509721E-16 0.000000E+00 9.00000E+100
585 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.001296E-15 0.000000E+00 9.00000E+100
586 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.501944E-15 0.000000E+00 9.00000E+100
587 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.101426E-15 0.000000E+00 9.00000E+100
588 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.001296E-15 0.000000E+00 9.00000E+100
589 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.301685E-15 0.000000E+00 9.00000E+100
590 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -8.010369E-16 0.000000E+00 9.00000E+100
591 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 3.003888E-16 0.000000E+00 9.00000E+100
592 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -6.258101E-16 0.000000E+00 9.00000E+100
593 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 4.005185E-16 0.000000E+00 9.00000E+100
594 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.001296E-16 0.000000E+00 9.00000E+100
595 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 7.760045E-16 0.000000E+00 9.00000E+100
596 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 3.003888E-16 0.000000E+00 9.00000E+100
597 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 5.006481E-17 0.000000E+00 9.00000E+100
598 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.753564E-16 0.000000E+00 9.00000E+100
599 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.001296E-16 0.000000E+00 9.00000E+100
600 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
601 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.476912E-15 0.000000E+00 9.00000E+100
602 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -2.503240E-16 0.000000E+00 9.00000E+100
603 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.752268E-16 0.000000E+00 9.00000E+100
604 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -4.756157E-16 0.000000E+00 9.00000E+100
605 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.752268E-16 0.000000E+00 9.00000E+100
606 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.752268E-16 0.000000E+00 9.00000E+100
607 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 1.752268E-16 0.000000E+00 9.00000E+100
608 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -1.001296E-16 0.000000E+00 9.00000E+100
609 traj.propelled_ascent.collocation_constraint.defects:V_w e 0.000000E+00 -7.509721E-17 0.000000E+00 9.00000E+100
Exit Status
Inform Description
0 Solve Succeeded
--------------------------------------------------------------------------------
Simulating trajectory traj
Done simulating trajectory traj
Launch angle: 46.8229 deg
Flight angle at end of propulsion: 38.3886 deg
Empty mass: 0.1891 kg
Water volume: 1.0273 L
Maximum range: 85.0967 m
Maximum height: 23.0818 m
Maximum velocity: 41.3029 m/s
Maximum Range Solution: Propulsive Phase#
plot_propelled_ascent(sol_out, sim_out)
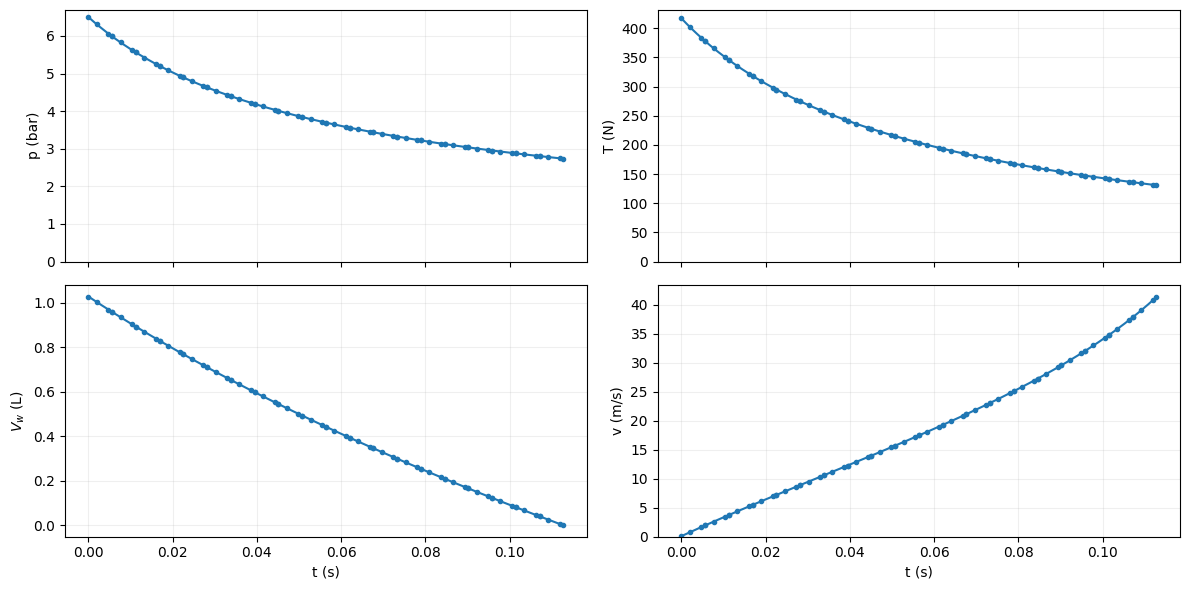
Maximum Range Solution: Height vs. Range#
plot_trajectory(sol_out, sim_out, legend_loc='center')
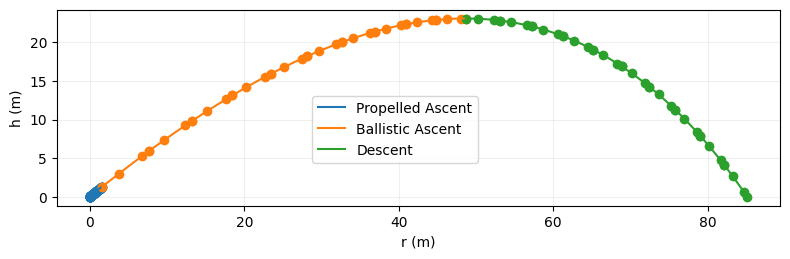
Maximum Range Solution: State History#
plot_states(sol_out, sim_out, legend_loc='lower center')
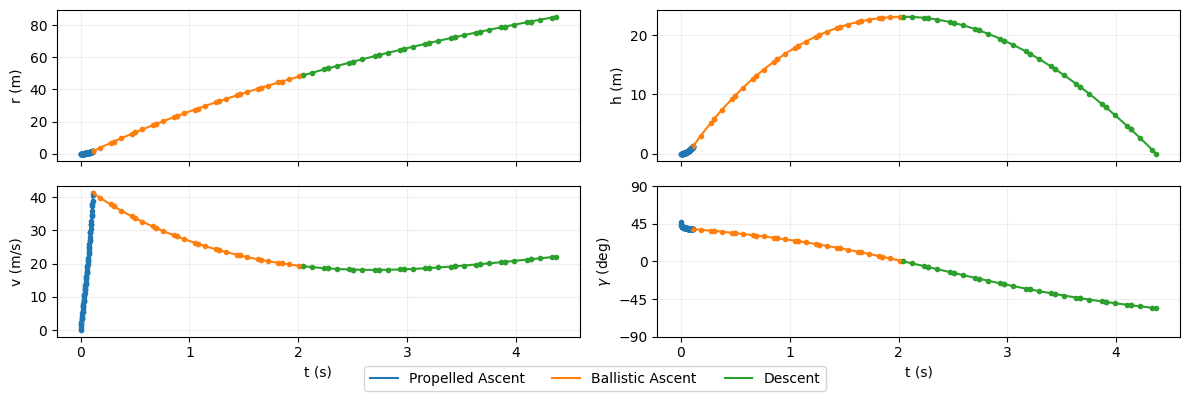
References#
Nozzles. 2013. URL: http://www.aircommandrockets.com/nozzles.htm.
Bernardo Bahia Monteiro. Optimization of a water rocket in openmdao/dymos. Aug 2020. URL: engrxiv.org/nqta8, doi:10.31224/osf.io/nqta8.
R Barrio-Perotti, E Blanco-Marigorta, K Argüelles-D\'ıaz, and J Fernández-Oro. Experimental evaluation of the drag coefficient of water rockets by a simple free-fall test. European Journal of Physics, 30(5):1039–1048, jul 2009. doi:10.1088/0143-0807/30/5/012.
Laura Fischer, Thomas Günther, Linda Herzig, Tobias Jarzina, Frank Klinker, Sabine Knipper, Franz-Georg Schürmann, and Marvin Wollek. On the approximation of D.I.Y. water rocket dynamics including air drag. arXiv:2001.08828, 2020. arXiv:2001.08828.
Alejandro Romanelli, Italo Bove, and Federico González Madina. Air expansion in a water rocket. American Journal of Physics, 81(10):762–766, oct 2013. doi:10.1119/1.4811116.
Glen E. Thorncroft, John R. Ridgely, and Christopher C. Pascual. Hydrodynamics and thrust characteristics of a water-propelled rocket. International Journal of Mechanical Engineering Education, 37(3):241–261, jul 2009. doi:10.7227/ijmee.37.3.9.