How do I run two phases parallel-in-time?#
Complex models sometimes encounter state variables which are best simulated on different time scales, with some state variables changing quickly (fast variables) and some evolving slowly (slow variables).
For instance, an aircraft trajectory optimization which includes vehicle component temperatures might see relatively gradual changes in altitude over the course of a two hour flight while temperatures of some components seem to exhibit step-function-like behavior on the same scale.
To accommodate both fast and slow variables in the same ODE, one would typically need to use a dense grid (with many segments/higher order segments). This can be unnecessarily burdensome when there are many slow variables or evaluating their rates is particularly expensive.
As a solution, Dymos allows the user to run two phases over the same range of times, where one phase may have a more sparse grid to accommodate the slow variables, and one has a more dense grid for the fast variables.
To connect the two phases, state variable values are passed from the first (slow) phase to the second (fast) phase as non-optimal dynamic control variables.
These values are then used to evaluate the rates of the fast variables.
Since outputs from the first phase in general will not fall on the appropriate grid points to be used by the second phase, interpolation is necessary.
This is one application of the interpolating timeseries component.
In the following example, we solve the brachistochrone problem but do so to minimize the arclength of the resulting wire instead of the time required for the bead to travel along the wire.
This is a trivial solution which should find a straight line from the starting point to the ending point.
There are two phases involved, the first utilizes the standard ODE for the brachistochrone problem. The second integrates the arclength (𝑆) of the wire using the equation:
The ODE for the wire arclength#
class BrachistochroneArclengthODE(om.ExplicitComponent):
def initialize(self):
self.options.declare('num_nodes', types=int)
def setup(self):
nn = self.options['num_nodes']
# Inputs
self.add_input('v', val=np.zeros(nn), desc='velocity', units='m/s')
self.add_input('theta', val=np.zeros(nn), desc='angle of wire', units='rad')
self.add_output('Sdot', val=np.zeros(nn), desc='rate of change of arclength', units='m/s')
# Setup partials
arange = np.arange(nn)
self.declare_partials(of='Sdot', wrt='v', rows=arange, cols=arange)
self.declare_partials(of='Sdot', wrt='theta', rows=arange, cols=arange)
def compute(self, inputs, outputs):
theta = inputs['theta']
v = inputs['v']
outputs['Sdot'] = np.sqrt(1.0 + (1.0/np.tan(theta))**2) * v * np.sin(theta)
def compute_partials(self, inputs, jacobian):
theta = inputs['theta']
v = inputs['v']
cos_theta = np.cos(theta)
sin_theta = np.sin(theta)
tan_theta = np.tan(theta)
cot_theta = 1.0 / tan_theta
csc_theta = 1.0 / sin_theta
jacobian['Sdot', 'v'] = sin_theta * np.sqrt(1.0 + cot_theta**2)
jacobian['Sdot', 'theta'] = v * (cos_theta * (cot_theta**2 + 1) - cot_theta * csc_theta) / \
(np.sqrt(1 + cot_theta**2))
The trick is that the bead velocity (\(v\)) is a state variable solved for in the first phase,
and the wire angle (\(\theta\)) is a control variable “owned” by the first phase. In the
second phase they are used as control variables with option opt=False
so that their values are
expected as inputs for the second phase. We need to connect their values from the first phase
to the second phase, at the control_input
node subset of the second phase.
In the following example, we instantiate two phases and add an interpolating timeseries to the first phase
which provides outputs at the control_input
nodes of the second phase. Those values are
then connected and the entire problem run. The result is that the position and velocity variables
are solved on a relatively coarse grid while the arclength of the wire is solved on a much denser grid.
Show code cell outputs
import numpy as np
import openmdao.api as om
class BrachistochroneODE(om.ExplicitComponent):
def initialize(self):
self.options.declare('num_nodes', types=int)
self.options.declare('static_gravity', types=(bool,), default=False,
desc='If True, treat gravity as a static (scalar) input, rather than '
'having different values at each node.')
def setup(self):
nn = self.options['num_nodes']
# Inputs
self.add_input('v', val=np.zeros(nn), desc='velocity', units='m/s')
if self.options['static_gravity']:
self.add_input('g', val=9.80665, desc='grav. acceleration', units='m/s/s',
tags=['dymos.static_target'])
else:
self.add_input('g', val=9.80665 * np.ones(nn), desc='grav. acceleration', units='m/s/s')
self.add_input('theta', val=np.ones(nn), desc='angle of wire', units='rad')
self.add_output('xdot', val=np.zeros(nn), desc='velocity component in x', units='m/s',
tags=['dymos.state_rate_source:x', 'dymos.state_units:m'])
self.add_output('ydot', val=np.zeros(nn), desc='velocity component in y', units='m/s',
tags=['dymos.state_rate_source:y', 'dymos.state_units:m'])
self.add_output('vdot', val=np.zeros(nn), desc='acceleration magnitude', units='m/s**2',
tags=['dymos.state_rate_source:v', 'dymos.state_units:m/s'])
self.add_output('check', val=np.zeros(nn), desc='check solution: v/sin(theta) = constant',
units='m/s')
# Setup partials
arange = np.arange(self.options['num_nodes'])
self.declare_partials(of='vdot', wrt='theta', rows=arange, cols=arange)
self.declare_partials(of='xdot', wrt='v', rows=arange, cols=arange)
self.declare_partials(of='xdot', wrt='theta', rows=arange, cols=arange)
self.declare_partials(of='ydot', wrt='v', rows=arange, cols=arange)
self.declare_partials(of='ydot', wrt='theta', rows=arange, cols=arange)
self.declare_partials(of='check', wrt='v', rows=arange, cols=arange)
self.declare_partials(of='check', wrt='theta', rows=arange, cols=arange)
if self.options['static_gravity']:
c = np.zeros(self.options['num_nodes'])
self.declare_partials(of='vdot', wrt='g', rows=arange, cols=c)
else:
self.declare_partials(of='vdot', wrt='g', rows=arange, cols=arange)
def compute(self, inputs, outputs):
theta = inputs['theta']
cos_theta = np.cos(theta)
sin_theta = np.sin(theta)
g = inputs['g']
v = inputs['v']
outputs['vdot'] = g * cos_theta
outputs['xdot'] = v * sin_theta
outputs['ydot'] = -v * cos_theta
outputs['check'] = v / sin_theta
def compute_partials(self, inputs, partials):
theta = inputs['theta']
cos_theta = np.cos(theta)
sin_theta = np.sin(theta)
g = inputs['g']
v = inputs['v']
partials['vdot', 'g'] = cos_theta
partials['vdot', 'theta'] = -g * sin_theta
partials['xdot', 'v'] = sin_theta
partials['xdot', 'theta'] = v * cos_theta
partials['ydot', 'v'] = -cos_theta
partials['ydot', 'theta'] = v * sin_theta
partials['check', 'v'] = 1 / sin_theta
partials['check', 'theta'] = -v * cos_theta / sin_theta ** 2
from dymos.examples.brachistochrone.brachistochrone_ode import BrachistochroneODE
import numpy as np
import matplotlib.pyplot as plt
import openmdao.api as om
import dymos as dm
p = om.Problem(model=om.Group())
p.driver = om.pyOptSparseDriver()
p.driver.options['optimizer'] = 'SLSQP'
p.driver.declare_coloring()
# The transcription of the first phase
tx0 = dm.GaussLobatto(num_segments=10, order=3, compressed=False)
# The transcription for the second phase (and the secondary timeseries outputs from the first phase)
tx1 = dm.Radau(num_segments=20, order=9, compressed=False)
#
# First Phase: Integrate the standard brachistochrone ODE
#
phase0 = dm.Phase(ode_class=BrachistochroneODE, transcription=tx0)
p.model.add_subsystem('phase0', phase0)
phase0.set_time_options(fix_initial=True, duration_bounds=(.5, 10))
phase0.add_state('x', fix_initial=True, fix_final=False)
phase0.add_state('y', fix_initial=True, fix_final=False)
phase0.add_state('v', fix_initial=True, fix_final=False)
phase0.add_control('theta', continuity=True, rate_continuity=True,
units='deg', lower=0.01, upper=179.9)
phase0.add_parameter('g', units='m/s**2', val=9.80665)
phase0.add_boundary_constraint('x', loc='final', equals=10)
phase0.add_boundary_constraint('y', loc='final', equals=5)
# Add alternative timeseries output to provide control inputs for the next phase
phase0.add_timeseries('timeseries2', transcription=tx1, subset='control_input')
#
# Second Phase: Integration of ArcLength
#
phase1 = dm.Phase(ode_class=BrachistochroneArclengthODE, transcription=tx1)
p.model.add_subsystem('phase1', phase1)
phase1.set_time_options(fix_initial=True, input_duration=True)
phase1.add_state('S', fix_initial=True, fix_final=False,
rate_source='Sdot', units='m')
phase1.add_control('theta', opt=False, units='deg', targets='theta')
phase1.add_control('v', opt=False, units='m/s', targets='v')
#
# Connect the two phases
#
p.model.connect('phase0.t_duration_val', 'phase1.t_duration')
p.model.connect('phase0.timeseries2.theta', 'phase1.controls:theta')
p.model.connect('phase0.timeseries2.v', 'phase1.controls:v')
# Minimize arclength at the end of the second phase
phase1.add_objective('S', loc='final', ref=1)
p.model.linear_solver = om.DirectSolver()
p.setup(check=True)
p['phase0.t_initial'] = 0.0
p['phase0.t_duration'] = 2.0
p.set_val('phase0.states:x', phase0.interp('x', ys=[0, 10]))
p.set_val('phase0.states:y', phase0.interp('y', ys=[10, 5]))
p.set_val('phase0.states:v', phase0.interp('v', ys=[0, 9.9]))
p.set_val('phase0.controls:theta', phase0.interp('theta', ys=[5, 100]))
p['phase0.parameters:g'] = 9.80665
p['phase1.states:S'] = 0.0
dm.run_problem(p)
fig, (ax0, ax1) = plt.subplots(2, 1)
fig.tight_layout()
ax0.plot(p.get_val('phase0.timeseries.x'), p.get_val('phase0.timeseries.y'), '.')
ax0.set_xlabel('x (m)')
ax0.set_ylabel('y (m)')
ax1.plot(p.get_val('phase1.timeseries.time'), p.get_val('phase1.timeseries.S'), '+')
ax1.set_xlabel('t (s)')
ax1.set_ylabel('S (m)')
plt.show()
'rhs_checking' is disabled for 'DirectSolver in <model> <class Group>' but that solver has redundant adjoint solves. If it is expensive to compute derivatives for this solver, turning on 'rhs_checking' may improve performance.
INFO: checking out_of_order
INFO: checking system
INFO: checking solvers
INFO: checking dup_inputs
INFO: checking missing_recorders
INFO: checking unserializable_options
INFO: checking comp_has_no_outputs
INFO: checking auto_ivc_warnings
Model viewer data has already been recorded for Driver.
INFO: checking out_of_order
INFO: checking system
INFO: checking solvers
INFO: checking dup_inputs
INFO: checking missing_recorders
INFO: checking unserializable_options
INFO: checking comp_has_no_outputs
INFO: checking auto_ivc_warnings
/usr/share/miniconda/envs/test/lib/python3.11/site-packages/openmdao/recorders/sqlite_recorder.py:231: UserWarning:The existing case recorder file, /home/runner/work/dymos/dymos/docs/dymos_book/faq/problem_out/dymos_solution.db, is being overwritten.
Full total jacobian for problem 'problem' was computed 3 times, taking 0.1542809509999188 seconds.
Total jacobian shape: (222, 287)
Jacobian shape: (222, 287) (8.14% nonzero)
FWD solves: 29 REV solves: 0
Total colors vs. total size: 29 vs 287 (89.90% improvement)
Sparsity computed using tolerance: 1e-25
Time to compute sparsity: 0.1543 sec
Time to compute coloring: 0.2416 sec
Memory to compute coloring: 5.6484 MB
Coloring created on: 2024-12-11 19:27:28
Optimization Problem -- Optimization using pyOpt_sparse
================================================================================
Objective Function: _objfunc
Solution:
--------------------------------------------------------------------------------
Total Time: 9.1907
User Objective Time : 0.2484
User Sensitivity Time : 2.2300
Interface Time : 0.5690
Opt Solver Time: 6.1434
Calls to Objective Function : 134
Calls to Sens Function : 103
Objectives
Index Name Value
0 phase1.states:S 1.118119E+01
Variables (c - continuous, i - integer, d - discrete)
Index Name Type Lower Bound Value Upper Bound Status
0 phase0.t_duration_0 c 5.000000E-01 2.210247E+00 1.000000E+01
1 phase0.states:x_0 c -1.000000E+21 1.390924E-01 1.000000E+21
2 phase0.states:x_1 c -1.000000E+21 1.390924E-01 1.000000E+21
3 phase0.states:x_2 c -1.000000E+21 4.697012E-01 1.000000E+21
4 phase0.states:x_3 c -1.000000E+21 4.697012E-01 1.000000E+21
5 phase0.states:x_4 c -1.000000E+21 9.890058E-01 1.000000E+21
6 phase0.states:x_5 c -1.000000E+21 9.890058E-01 1.000000E+21
7 phase0.states:x_6 c -1.000000E+21 1.698475E+00 1.000000E+21
8 phase0.states:x_7 c -1.000000E+21 1.698475E+00 1.000000E+21
9 phase0.states:x_8 c -1.000000E+21 2.605083E+00 1.000000E+21
10 phase0.states:x_9 c -1.000000E+21 2.605083E+00 1.000000E+21
11 phase0.states:x_10 c -1.000000E+21 3.704224E+00 1.000000E+21
12 phase0.states:x_11 c -1.000000E+21 3.704224E+00 1.000000E+21
13 phase0.states:x_12 c -1.000000E+21 4.986862E+00 1.000000E+21
14 phase0.states:x_13 c -1.000000E+21 4.986862E+00 1.000000E+21
15 phase0.states:x_14 c -1.000000E+21 6.463732E+00 1.000000E+21
16 phase0.states:x_15 c -1.000000E+21 6.463732E+00 1.000000E+21
17 phase0.states:x_16 c -1.000000E+21 8.135741E+00 1.000000E+21
18 phase0.states:x_17 c -1.000000E+21 8.135741E+00 1.000000E+21
19 phase0.states:x_18 c -1.000000E+21 1.000000E+01 1.000000E+21
20 phase0.states:y_0 c -1.000000E+21 9.926172E+00 1.000000E+21
21 phase0.states:y_1 c -1.000000E+21 9.926172E+00 1.000000E+21
22 phase0.states:y_2 c -1.000000E+21 9.767443E+00 1.000000E+21
23 phase0.states:y_3 c -1.000000E+21 9.767443E+00 1.000000E+21
24 phase0.states:y_4 c -1.000000E+21 9.507601E+00 1.000000E+21
25 phase0.states:y_5 c -1.000000E+21 9.507601E+00 1.000000E+21
26 phase0.states:y_6 c -1.000000E+21 9.145739E+00 1.000000E+21
27 phase0.states:y_7 c -1.000000E+21 9.145739E+00 1.000000E+21
28 phase0.states:y_8 c -1.000000E+21 8.694668E+00 1.000000E+21
29 phase0.states:y_9 c -1.000000E+21 8.694668E+00 1.000000E+21
30 phase0.states:y_10 c -1.000000E+21 8.156003E+00 1.000000E+21
31 phase0.states:y_11 c -1.000000E+21 8.156003E+00 1.000000E+21
32 phase0.states:y_12 c -1.000000E+21 7.507055E+00 1.000000E+21
33 phase0.states:y_13 c -1.000000E+21 7.507055E+00 1.000000E+21
34 phase0.states:y_14 c -1.000000E+21 6.762417E+00 1.000000E+21
35 phase0.states:y_15 c -1.000000E+21 6.762417E+00 1.000000E+21
36 phase0.states:y_16 c -1.000000E+21 5.928277E+00 1.000000E+21
37 phase0.states:y_17 c -1.000000E+21 5.928277E+00 1.000000E+21
38 phase0.states:y_18 c -1.000000E+21 5.000000E+00 1.000000E+21
39 phase0.states:v_0 c -1.000000E+21 1.212937E+00 1.000000E+21
40 phase0.states:v_1 c -1.000000E+21 1.212937E+00 1.000000E+21
41 phase0.states:v_2 c -1.000000E+21 2.141310E+00 1.000000E+21
42 phase0.states:v_3 c -1.000000E+21 2.141310E+00 1.000000E+21
43 phase0.states:v_4 c -1.000000E+21 3.111522E+00 1.000000E+21
44 phase0.states:v_5 c -1.000000E+21 3.111522E+00 1.000000E+21
45 phase0.states:v_6 c -1.000000E+21 4.096205E+00 1.000000E+21
46 phase0.states:v_7 c -1.000000E+21 4.096205E+00 1.000000E+21
47 phase0.states:v_8 c -1.000000E+21 5.062201E+00 1.000000E+21
48 phase0.states:v_9 c -1.000000E+21 5.062201E+00 1.000000E+21
49 phase0.states:v_10 c -1.000000E+21 6.015885E+00 1.000000E+21
50 phase0.states:v_11 c -1.000000E+21 6.015885E+00 1.000000E+21
51 phase0.states:v_12 c -1.000000E+21 6.994205E+00 1.000000E+21
52 phase0.states:v_13 c -1.000000E+21 6.994205E+00 1.000000E+21
53 phase0.states:v_14 c -1.000000E+21 7.970176E+00 1.000000E+21
54 phase0.states:v_15 c -1.000000E+21 7.970176E+00 1.000000E+21
55 phase0.states:v_16 c -1.000000E+21 8.937781E+00 1.000000E+21
56 phase0.states:v_17 c -1.000000E+21 8.937781E+00 1.000000E+21
57 phase0.states:v_18 c -1.000000E+21 9.904065E+00 1.000000E+21
58 phase0.controls:theta_0 c 1.000000E-02 1.671533E+01 1.799000E+02
59 phase0.controls:theta_1 c 1.000000E-02 5.933426E+01 1.799000E+02
60 phase0.controls:theta_2 c 1.000000E-02 6.891623E+01 1.799000E+02
61 phase0.controls:theta_3 c 1.000000E-02 6.891623E+01 1.799000E+02
62 phase0.controls:theta_4 c 1.000000E-02 6.396317E+01 1.799000E+02
63 phase0.controls:theta_5 c 1.000000E-02 6.297700E+01 1.799000E+02
64 phase0.controls:theta_6 c 1.000000E-02 6.297700E+01 1.799000E+02
65 phase0.controls:theta_7 c 1.000000E-02 6.355032E+01 1.799000E+02
66 phase0.controls:theta_8 c 1.000000E-02 6.327570E+01 1.799000E+02
67 phase0.controls:theta_9 c 1.000000E-02 6.327570E+01 1.799000E+02
68 phase0.controls:theta_10 c 1.000000E-02 6.287968E+01 1.799000E+02
69 phase0.controls:theta_11 c 1.000000E-02 6.308877E+01 1.799000E+02
70 phase0.controls:theta_12 c 1.000000E-02 6.308877E+01 1.799000E+02
71 phase0.controls:theta_13 c 1.000000E-02 6.355024E+01 1.799000E+02
72 phase0.controls:theta_14 c 1.000000E-02 6.391133E+01 1.799000E+02
73 phase0.controls:theta_15 c 1.000000E-02 6.391133E+01 1.799000E+02
74 phase0.controls:theta_16 c 1.000000E-02 6.397805E+01 1.799000E+02
75 phase0.controls:theta_17 c 1.000000E-02 6.355640E+01 1.799000E+02
76 phase0.controls:theta_18 c 1.000000E-02 6.355640E+01 1.799000E+02
77 phase0.controls:theta_19 c 1.000000E-02 6.309950E+01 1.799000E+02
78 phase0.controls:theta_20 c 1.000000E-02 6.306048E+01 1.799000E+02
79 phase0.controls:theta_21 c 1.000000E-02 6.306048E+01 1.799000E+02
80 phase0.controls:theta_22 c 1.000000E-02 6.323669E+01 1.799000E+02
81 phase0.controls:theta_23 c 1.000000E-02 6.342548E+01 1.799000E+02
82 phase0.controls:theta_24 c 1.000000E-02 6.342548E+01 1.799000E+02
83 phase0.controls:theta_25 c 1.000000E-02 6.351981E+01 1.799000E+02
84 phase0.controls:theta_26 c 1.000000E-02 6.341262E+01 1.799000E+02
85 phase0.controls:theta_27 c 1.000000E-02 6.341262E+01 1.799000E+02
86 phase0.controls:theta_28 c 1.000000E-02 6.344499E+01 1.799000E+02
87 phase0.controls:theta_29 c 1.000000E-02 6.395797E+01 1.799000E+02
88 phase1.states:S_0 c -1.000000E+21 1.019016E-04 1.000000E+21
89 phase1.states:S_1 c -1.000000E+21 1.053653E-03 1.000000E+21
90 phase1.states:S_2 c -1.000000E+21 4.089151E-03 1.000000E+21
91 phase1.states:S_3 c -1.000000E+21 1.007433E-02 1.000000E+21
92 phase1.states:S_4 c -1.000000E+21 1.880365E-02 1.000000E+21
93 phase1.states:S_5 c -1.000000E+21 2.884538E-02 1.000000E+21
94 phase1.states:S_6 c -1.000000E+21 3.797642E-02 1.000000E+21
95 phase1.states:S_7 c -1.000000E+21 4.394894E-02 1.000000E+21
96 phase1.states:S_8 c -1.000000E+21 4.544833E-02 1.000000E+21
97 phase1.states:S_9 c -1.000000E+21 4.544833E-02 1.000000E+21
98 phase1.states:S_10 c -1.000000E+21 4.930524E-02 1.000000E+21
99 phase1.states:S_11 c -1.000000E+21 5.838955E-02 1.000000E+21
100 phase1.states:S_12 c -1.000000E+21 7.242388E-02 1.000000E+21
101 phase1.states:S_13 c -1.000000E+21 9.044517E-02 1.000000E+21
102 phase1.states:S_14 c -1.000000E+21 1.105293E-01 1.000000E+21
103 phase1.states:S_15 c -1.000000E+21 1.299675E-01 1.000000E+21
104 phase1.states:S_16 c -1.000000E+21 1.458122E-01 1.000000E+21
105 phase1.states:S_17 c -1.000000E+21 1.555406E-01 1.000000E+21
106 phase1.states:S_18 c -1.000000E+21 1.579190E-01 1.000000E+21
107 phase1.states:S_19 c -1.000000E+21 1.579190E-01 1.000000E+21
108 phase1.states:S_20 c -1.000000E+21 1.639474E-01 1.000000E+21
109 phase1.states:S_21 c -1.000000E+21 1.777488E-01 1.000000E+21
110 phase1.states:S_22 c -1.000000E+21 1.982656E-01 1.000000E+21
111 phase1.states:S_23 c -1.000000E+21 2.236927E-01 1.000000E+21
112 phase1.states:S_24 c -1.000000E+21 2.513423E-01 1.000000E+21
113 phase1.states:S_25 c -1.000000E+21 2.777794E-01 1.000000E+21
114 phase1.states:S_26 c -1.000000E+21 2.992698E-01 1.000000E+21
115 phase1.states:S_27 c -1.000000E+21 3.124872E-01 1.000000E+21
116 phase1.states:S_28 c -1.000000E+21 3.157241E-01 1.000000E+21
117 phase1.states:S_29 c -1.000000E+21 3.157241E-01 1.000000E+21
118 phase1.states:S_30 c -1.000000E+21 3.239214E-01 1.000000E+21
119 phase1.states:S_31 c -1.000000E+21 3.426097E-01 1.000000E+21
120 phase1.states:S_32 c -1.000000E+21 3.702048E-01 1.000000E+21
121 phase1.states:S_33 c -1.000000E+21 4.041265E-01 1.000000E+21
122 phase1.states:S_34 c -1.000000E+21 4.407045E-01 1.000000E+21
123 phase1.states:S_35 c -1.000000E+21 4.754121E-01 1.000000E+21
124 phase1.states:S_36 c -1.000000E+21 5.034534E-01 1.000000E+21
125 phase1.states:S_37 c -1.000000E+21 5.206290E-01 1.000000E+21
126 phase1.states:S_38 c -1.000000E+21 5.248275E-01 1.000000E+21
127 phase1.states:S_39 c -1.000000E+21 5.248275E-01 1.000000E+21
128 phase1.states:S_40 c -1.000000E+21 5.354434E-01 1.000000E+21
129 phase1.states:S_41 c -1.000000E+21 5.595519E-01 1.000000E+21
130 phase1.states:S_42 c -1.000000E+21 5.949177E-01 1.000000E+21
131 phase1.states:S_43 c -1.000000E+21 6.380243E-01 1.000000E+21
132 phase1.states:S_44 c -1.000000E+21 6.840715E-01 1.000000E+21
133 phase1.states:S_45 c -1.000000E+21 7.273651E-01 1.000000E+21
134 phase1.states:S_46 c -1.000000E+21 7.620708E-01 1.000000E+21
135 phase1.states:S_47 c -1.000000E+21 7.832121E-01 1.000000E+21
136 phase1.states:S_48 c -1.000000E+21 7.883667E-01 1.000000E+21
137 phase1.states:S_49 c -1.000000E+21 7.883667E-01 1.000000E+21
138 phase1.states:S_50 c -1.000000E+21 8.013814E-01 1.000000E+21
139 phase1.states:S_51 c -1.000000E+21 8.308479E-01 1.000000E+21
140 phase1.states:S_52 c -1.000000E+21 8.738621E-01 1.000000E+21
141 phase1.states:S_53 c -1.000000E+21 9.259807E-01 1.000000E+21
142 phase1.states:S_54 c -1.000000E+21 9.813141E-01 1.000000E+21
143 phase1.states:S_55 c -1.000000E+21 1.033049E+00 1.000000E+21
144 phase1.states:S_56 c -1.000000E+21 1.074336E+00 1.000000E+21
145 phase1.states:S_57 c -1.000000E+21 1.099411E+00 1.000000E+21
146 phase1.states:S_58 c -1.000000E+21 1.105516E+00 1.000000E+21
147 phase1.states:S_59 c -1.000000E+21 1.105516E+00 1.000000E+21
148 phase1.states:S_60 c -1.000000E+21 1.120918E+00 1.000000E+21
149 phase1.states:S_61 c -1.000000E+21 1.155723E+00 1.000000E+21
150 phase1.states:S_62 c -1.000000E+21 1.206376E+00 1.000000E+21
151 phase1.states:S_63 c -1.000000E+21 1.267521E+00 1.000000E+21
152 phase1.states:S_64 c -1.000000E+21 1.332189E+00 1.000000E+21
153 phase1.states:S_65 c -1.000000E+21 1.392440E+00 1.000000E+21
154 phase1.states:S_66 c -1.000000E+21 1.440388E+00 1.000000E+21
155 phase1.states:S_67 c -1.000000E+21 1.469454E+00 1.000000E+21
156 phase1.states:S_68 c -1.000000E+21 1.476525E+00 1.000000E+21
157 phase1.states:S_69 c -1.000000E+21 1.476525E+00 1.000000E+21
158 phase1.states:S_70 c -1.000000E+21 1.494351E+00 1.000000E+21
159 phase1.states:S_71 c -1.000000E+21 1.534576E+00 1.000000E+21
160 phase1.states:S_72 c -1.000000E+21 1.592973E+00 1.000000E+21
161 phase1.states:S_73 c -1.000000E+21 1.663254E+00 1.000000E+21
162 phase1.states:S_74 c -1.000000E+21 1.737350E+00 1.000000E+21
163 phase1.states:S_75 c -1.000000E+21 1.806183E+00 1.000000E+21
164 phase1.states:S_76 c -1.000000E+21 1.860830E+00 1.000000E+21
165 phase1.states:S_77 c -1.000000E+21 1.893903E+00 1.000000E+21
166 phase1.states:S_78 c -1.000000E+21 1.901943E+00 1.000000E+21
167 phase1.states:S_79 c -1.000000E+21 1.901943E+00 1.000000E+21
168 phase1.states:S_80 c -1.000000E+21 1.922202E+00 1.000000E+21
169 phase1.states:S_81 c -1.000000E+21 1.967859E+00 1.000000E+21
170 phase1.states:S_82 c -1.000000E+21 2.034007E+00 1.000000E+21
171 phase1.states:S_83 c -1.000000E+21 2.113417E+00 1.000000E+21
172 phase1.states:S_84 c -1.000000E+21 2.196905E+00 1.000000E+21
173 phase1.states:S_85 c -1.000000E+21 2.274261E+00 1.000000E+21
174 phase1.states:S_86 c -1.000000E+21 2.335542E+00 1.000000E+21
175 phase1.states:S_87 c -1.000000E+21 2.372573E+00 1.000000E+21
176 phase1.states:S_88 c -1.000000E+21 2.381569E+00 1.000000E+21
177 phase1.states:S_89 c -1.000000E+21 2.381569E+00 1.000000E+21
178 phase1.states:S_90 c -1.000000E+21 2.404227E+00 1.000000E+21
179 phase1.states:S_91 c -1.000000E+21 2.455239E+00 1.000000E+21
180 phase1.states:S_92 c -1.000000E+21 2.529022E+00 1.000000E+21
181 phase1.states:S_93 c -1.000000E+21 2.617415E+00 1.000000E+21
182 phase1.states:S_94 c -1.000000E+21 2.710146E+00 1.000000E+21
183 phase1.states:S_95 c -1.000000E+21 2.795891E+00 1.000000E+21
184 phase1.states:S_96 c -1.000000E+21 2.863703E+00 1.000000E+21
185 phase1.states:S_97 c -1.000000E+21 2.904635E+00 1.000000E+21
186 phase1.states:S_98 c -1.000000E+21 2.914573E+00 1.000000E+21
187 phase1.states:S_99 c -1.000000E+21 2.914573E+00 1.000000E+21
188 phase1.states:S_100 c -1.000000E+21 2.939595E+00 1.000000E+21
189 phase1.states:S_101 c -1.000000E+21 2.995883E+00 1.000000E+21
190 phase1.states:S_102 c -1.000000E+21 3.077188E+00 1.000000E+21
191 phase1.states:S_103 c -1.000000E+21 3.174433E+00 1.000000E+21
192 phase1.states:S_104 c -1.000000E+21 3.276279E+00 1.000000E+21
193 phase1.states:S_105 c -1.000000E+21 3.370306E+00 1.000000E+21
194 phase1.states:S_106 c -1.000000E+21 3.444579E+00 1.000000E+21
195 phase1.states:S_107 c -1.000000E+21 3.489374E+00 1.000000E+21
196 phase1.states:S_108 c -1.000000E+21 3.500247E+00 1.000000E+21
197 phase1.states:S_109 c -1.000000E+21 3.500247E+00 1.000000E+21
198 phase1.states:S_110 c -1.000000E+21 3.527613E+00 1.000000E+21
199 phase1.states:S_111 c -1.000000E+21 3.589143E+00 1.000000E+21
200 phase1.states:S_112 c -1.000000E+21 3.677943E+00 1.000000E+21
201 phase1.states:S_113 c -1.000000E+21 3.784033E+00 1.000000E+21
202 phase1.states:S_114 c -1.000000E+21 3.895010E+00 1.000000E+21
203 phase1.states:S_115 c -1.000000E+21 3.997355E+00 1.000000E+21
204 phase1.states:S_116 c -1.000000E+21 4.078123E+00 1.000000E+21
205 phase1.states:S_117 c -1.000000E+21 4.126805E+00 1.000000E+21
206 phase1.states:S_118 c -1.000000E+21 4.138617E+00 1.000000E+21
207 phase1.states:S_119 c -1.000000E+21 4.138617E+00 1.000000E+21
208 phase1.states:S_120 c -1.000000E+21 4.168344E+00 1.000000E+21
209 phase1.states:S_121 c -1.000000E+21 4.235155E+00 1.000000E+21
210 phase1.states:S_122 c -1.000000E+21 4.331513E+00 1.000000E+21
211 phase1.states:S_123 c -1.000000E+21 4.446541E+00 1.000000E+21
212 phase1.states:S_124 c -1.000000E+21 4.566765E+00 1.000000E+21
213 phase1.states:S_125 c -1.000000E+21 4.677548E+00 1.000000E+21
214 phase1.states:S_126 c -1.000000E+21 4.764916E+00 1.000000E+21
215 phase1.states:S_127 c -1.000000E+21 4.817552E+00 1.000000E+21
216 phase1.states:S_128 c -1.000000E+21 4.830321E+00 1.000000E+21
217 phase1.states:S_129 c -1.000000E+21 4.830321E+00 1.000000E+21
218 phase1.states:S_130 c -1.000000E+21 4.862452E+00 1.000000E+21
219 phase1.states:S_131 c -1.000000E+21 4.934635E+00 1.000000E+21
220 phase1.states:S_132 c -1.000000E+21 5.038675E+00 1.000000E+21
221 phase1.states:S_133 c -1.000000E+21 5.162774E+00 1.000000E+21
222 phase1.states:S_134 c -1.000000E+21 5.292368E+00 1.000000E+21
223 phase1.states:S_135 c -1.000000E+21 5.411686E+00 1.000000E+21
224 phase1.states:S_136 c -1.000000E+21 5.505723E+00 1.000000E+21
225 phase1.states:S_137 c -1.000000E+21 5.562350E+00 1.000000E+21
226 phase1.states:S_138 c -1.000000E+21 5.576084E+00 1.000000E+21
227 phase1.states:S_139 c -1.000000E+21 5.576084E+00 1.000000E+21
228 phase1.states:S_140 c -1.000000E+21 5.610638E+00 1.000000E+21
229 phase1.states:S_141 c -1.000000E+21 5.688237E+00 1.000000E+21
230 phase1.states:S_142 c -1.000000E+21 5.800015E+00 1.000000E+21
231 phase1.states:S_143 c -1.000000E+21 5.933238E+00 1.000000E+21
232 phase1.states:S_144 c -1.000000E+21 6.072236E+00 1.000000E+21
233 phase1.states:S_145 c -1.000000E+21 6.200104E+00 1.000000E+21
234 phase1.states:S_146 c -1.000000E+21 6.300804E+00 1.000000E+21
235 phase1.states:S_147 c -1.000000E+21 6.361412E+00 1.000000E+21
236 phase1.states:S_148 c -1.000000E+21 6.376108E+00 1.000000E+21
237 phase1.states:S_149 c -1.000000E+21 6.376108E+00 1.000000E+21
238 phase1.states:S_150 c -1.000000E+21 6.413076E+00 1.000000E+21
239 phase1.states:S_151 c -1.000000E+21 6.496067E+00 1.000000E+21
240 phase1.states:S_152 c -1.000000E+21 6.615542E+00 1.000000E+21
241 phase1.states:S_153 c -1.000000E+21 6.757831E+00 1.000000E+21
242 phase1.states:S_154 c -1.000000E+21 6.906171E+00 1.000000E+21
243 phase1.states:S_155 c -1.000000E+21 7.042528E+00 1.000000E+21
244 phase1.states:S_156 c -1.000000E+21 7.149846E+00 1.000000E+21
245 phase1.states:S_157 c -1.000000E+21 7.214408E+00 1.000000E+21
246 phase1.states:S_158 c -1.000000E+21 7.230060E+00 1.000000E+21
247 phase1.states:S_159 c -1.000000E+21 7.230060E+00 1.000000E+21
248 phase1.states:S_160 c -1.000000E+21 7.269427E+00 1.000000E+21
249 phase1.states:S_161 c -1.000000E+21 7.357776E+00 1.000000E+21
250 phase1.states:S_162 c -1.000000E+21 7.484895E+00 1.000000E+21
251 phase1.states:S_163 c -1.000000E+21 7.636190E+00 1.000000E+21
252 phase1.states:S_164 c -1.000000E+21 7.793808E+00 1.000000E+21
253 phase1.states:S_165 c -1.000000E+21 7.938600E+00 1.000000E+21
254 phase1.states:S_166 c -1.000000E+21 8.052495E+00 1.000000E+21
255 phase1.states:S_167 c -1.000000E+21 8.120990E+00 1.000000E+21
256 phase1.states:S_168 c -1.000000E+21 8.137592E+00 1.000000E+21
257 phase1.states:S_169 c -1.000000E+21 8.137592E+00 1.000000E+21
258 phase1.states:S_170 c -1.000000E+21 8.179346E+00 1.000000E+21
259 phase1.states:S_171 c -1.000000E+21 8.273026E+00 1.000000E+21
260 phase1.states:S_172 c -1.000000E+21 8.407762E+00 1.000000E+21
261 phase1.states:S_173 c -1.000000E+21 8.568038E+00 1.000000E+21
262 phase1.states:S_174 c -1.000000E+21 8.734919E+00 1.000000E+21
263 phase1.states:S_175 c -1.000000E+21 8.888139E+00 1.000000E+21
264 phase1.states:S_176 c -1.000000E+21 9.008610E+00 1.000000E+21
265 phase1.states:S_177 c -1.000000E+21 9.081037E+00 1.000000E+21
266 phase1.states:S_178 c -1.000000E+21 9.098591E+00 1.000000E+21
267 phase1.states:S_179 c -1.000000E+21 9.098591E+00 1.000000E+21
268 phase1.states:S_180 c -1.000000E+21 9.142731E+00 1.000000E+21
269 phase1.states:S_181 c -1.000000E+21 9.241748E+00 1.000000E+21
270 phase1.states:S_182 c -1.000000E+21 9.384113E+00 1.000000E+21
271 phase1.states:S_183 c -1.000000E+21 9.553393E+00 1.000000E+21
272 phase1.states:S_184 c -1.000000E+21 9.729566E+00 1.000000E+21
273 phase1.states:S_185 c -1.000000E+21 9.891243E+00 1.000000E+21
274 phase1.states:S_186 c -1.000000E+21 1.001831E+01 1.000000E+21
275 phase1.states:S_187 c -1.000000E+21 1.009469E+01 1.000000E+21
276 phase1.states:S_188 c -1.000000E+21 1.011319E+01 1.000000E+21
277 phase1.states:S_189 c -1.000000E+21 1.011319E+01 1.000000E+21
278 phase1.states:S_190 c -1.000000E+21 1.015973E+01 1.000000E+21
279 phase1.states:S_191 c -1.000000E+21 1.026409E+01 1.000000E+21
280 phase1.states:S_192 c -1.000000E+21 1.041408E+01 1.000000E+21
281 phase1.states:S_193 c -1.000000E+21 1.059234E+01 1.000000E+21
282 phase1.states:S_194 c -1.000000E+21 1.077776E+01 1.000000E+21
283 phase1.states:S_195 c -1.000000E+21 1.094784E+01 1.000000E+21
284 phase1.states:S_196 c -1.000000E+21 1.108145E+01 1.000000E+21
285 phase1.states:S_197 c -1.000000E+21 1.116174E+01 1.000000E+21
286 phase1.states:S_198 c -1.000000E+21 1.118119E+01 1.000000E+21
Constraints (i - inequality, e - equality)
Index Name Type Lower Value Upper Status Lagrange Multiplier (N/A)
0 phase0->final_boundary_constraint->x e 1.000000E+01 1.000000E+01 1.000000E+01 9.00000E+100
1 phase0->final_boundary_constraint->y e 5.000000E+00 5.000000E+00 5.000000E+00 9.00000E+100
2 phase0.collocation_constraint.defects:x e 0.000000E+00 2.755816E-10 0.000000E+00 9.00000E+100
3 phase0.collocation_constraint.defects:x e 0.000000E+00 1.409761E-10 0.000000E+00 9.00000E+100
4 phase0.collocation_constraint.defects:x e 0.000000E+00 1.105384E-11 0.000000E+00 9.00000E+100
5 phase0.collocation_constraint.defects:x e 0.000000E+00 1.603156E-11 0.000000E+00 9.00000E+100
6 phase0.collocation_constraint.defects:x e 0.000000E+00 1.546153E-11 0.000000E+00 9.00000E+100
7 phase0.collocation_constraint.defects:x e 0.000000E+00 3.141736E-11 0.000000E+00 9.00000E+100
8 phase0.collocation_constraint.defects:x e 0.000000E+00 3.950099E-11 0.000000E+00 9.00000E+100
9 phase0.collocation_constraint.defects:x e 0.000000E+00 1.601237E-11 0.000000E+00 9.00000E+100
10 phase0.collocation_constraint.defects:x e 0.000000E+00 5.979771E-11 0.000000E+00 9.00000E+100
11 phase0.collocation_constraint.defects:x e 0.000000E+00 1.328779E-11 0.000000E+00 9.00000E+100
12 phase0.collocation_constraint.defects:y e 0.000000E+00 1.210079E-10 0.000000E+00 9.00000E+100
13 phase0.collocation_constraint.defects:y e 0.000000E+00 7.331001E-11 0.000000E+00 9.00000E+100
14 phase0.collocation_constraint.defects:y e 0.000000E+00 -1.323573E-10 0.000000E+00 9.00000E+100
15 phase0.collocation_constraint.defects:y e 0.000000E+00 -3.740524E-11 0.000000E+00 9.00000E+100
16 phase0.collocation_constraint.defects:y e 0.000000E+00 -7.183501E-12 0.000000E+00 9.00000E+100
17 phase0.collocation_constraint.defects:y e 0.000000E+00 -5.728701E-12 0.000000E+00 9.00000E+100
18 phase0.collocation_constraint.defects:y e 0.000000E+00 -1.960287E-11 0.000000E+00 9.00000E+100
19 phase0.collocation_constraint.defects:y e 0.000000E+00 1.141147E-12 0.000000E+00 9.00000E+100
20 phase0.collocation_constraint.defects:y e 0.000000E+00 1.458260E-11 0.000000E+00 9.00000E+100
21 phase0.collocation_constraint.defects:y e 0.000000E+00 -4.961014E-11 0.000000E+00 9.00000E+100
22 phase0.collocation_constraint.defects:v e 0.000000E+00 1.339416E-09 0.000000E+00 9.00000E+100
23 phase0.collocation_constraint.defects:v e 0.000000E+00 1.610132E-10 0.000000E+00 9.00000E+100
24 phase0.collocation_constraint.defects:v e 0.000000E+00 -5.227523E-12 0.000000E+00 9.00000E+100
25 phase0.collocation_constraint.defects:v e 0.000000E+00 -1.358834E-11 0.000000E+00 9.00000E+100
26 phase0.collocation_constraint.defects:v e 0.000000E+00 4.582745E-12 0.000000E+00 9.00000E+100
27 phase0.collocation_constraint.defects:v e 0.000000E+00 3.323705E-11 0.000000E+00 9.00000E+100
28 phase0.collocation_constraint.defects:v e 0.000000E+00 -1.354604E-11 0.000000E+00 9.00000E+100
29 phase0.collocation_constraint.defects:v e 0.000000E+00 -9.883590E-12 0.000000E+00 9.00000E+100
30 phase0.collocation_constraint.defects:v e 0.000000E+00 -1.244238E-11 0.000000E+00 9.00000E+100
31 phase0.collocation_constraint.defects:v e 0.000000E+00 4.786858E-11 0.000000E+00 9.00000E+100
32 phase0.continuity_comp.defect_states:x e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
33 phase0.continuity_comp.defect_states:x e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
34 phase0.continuity_comp.defect_states:x e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
35 phase0.continuity_comp.defect_states:x e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
36 phase0.continuity_comp.defect_states:x e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
37 phase0.continuity_comp.defect_states:x e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
38 phase0.continuity_comp.defect_states:x e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
39 phase0.continuity_comp.defect_states:x e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
40 phase0.continuity_comp.defect_states:x e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
41 phase0.continuity_comp.defect_states:y e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
42 phase0.continuity_comp.defect_states:y e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
43 phase0.continuity_comp.defect_states:y e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
44 phase0.continuity_comp.defect_states:y e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
45 phase0.continuity_comp.defect_states:y e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
46 phase0.continuity_comp.defect_states:y e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
47 phase0.continuity_comp.defect_states:y e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
48 phase0.continuity_comp.defect_states:y e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
49 phase0.continuity_comp.defect_states:y e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
50 phase0.continuity_comp.defect_states:v e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
51 phase0.continuity_comp.defect_states:v e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
52 phase0.continuity_comp.defect_states:v e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
53 phase0.continuity_comp.defect_states:v e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
54 phase0.continuity_comp.defect_states:v e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
55 phase0.continuity_comp.defect_states:v e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
56 phase0.continuity_comp.defect_states:v e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
57 phase0.continuity_comp.defect_states:v e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
58 phase0.continuity_comp.defect_states:v e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
59 phase0.continuity_comp.defect_control_rates:theta_rate e 0.000000E+00 -1.963094E-13 0.000000E+00 9.00000E+100
60 phase0.continuity_comp.defect_control_rates:theta_rate e 0.000000E+00 -7.067139E-14 0.000000E+00 9.00000E+100
61 phase0.continuity_comp.defect_control_rates:theta_rate e 0.000000E+00 -1.452690E-13 0.000000E+00 9.00000E+100
62 phase0.continuity_comp.defect_control_rates:theta_rate e 0.000000E+00 1.423243E-13 0.000000E+00 9.00000E+100
63 phase0.continuity_comp.defect_control_rates:theta_rate e 0.000000E+00 -2.522576E-13 0.000000E+00 9.00000E+100
64 phase0.continuity_comp.defect_control_rates:theta_rate e 0.000000E+00 1.344719E-13 0.000000E+00 9.00000E+100
65 phase0.continuity_comp.defect_control_rates:theta_rate e 0.000000E+00 7.116216E-14 0.000000E+00 9.00000E+100
66 phase0.continuity_comp.defect_control_rates:theta_rate e 0.000000E+00 -2.844033E-13 0.000000E+00 9.00000E+100
67 phase0.continuity_comp.defect_control_rates:theta_rate e 0.000000E+00 -3.197390E-13 0.000000E+00 9.00000E+100
68 phase0.continuity_comp.defect_controls:theta e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
69 phase0.continuity_comp.defect_controls:theta e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
70 phase0.continuity_comp.defect_controls:theta e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
71 phase0.continuity_comp.defect_controls:theta e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
72 phase0.continuity_comp.defect_controls:theta e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
73 phase0.continuity_comp.defect_controls:theta e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
74 phase0.continuity_comp.defect_controls:theta e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
75 phase0.continuity_comp.defect_controls:theta e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
76 phase0.continuity_comp.defect_controls:theta e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
77 phase1.collocation_constraint.defects:S e 0.000000E+00 2.775558E-17 0.000000E+00 9.00000E+100
78 phase1.collocation_constraint.defects:S e 0.000000E+00 -3.823801E-13 0.000000E+00 9.00000E+100
79 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.123312E-12 0.000000E+00 9.00000E+100
80 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.909805E-12 0.000000E+00 9.00000E+100
81 phase1.collocation_constraint.defects:S e 0.000000E+00 -2.426148E-12 0.000000E+00 9.00000E+100
82 phase1.collocation_constraint.defects:S e 0.000000E+00 -2.492860E-12 0.000000E+00 9.00000E+100
83 phase1.collocation_constraint.defects:S e 0.000000E+00 -2.142502E-12 0.000000E+00 9.00000E+100
84 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.597186E-12 0.000000E+00 9.00000E+100
85 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.157103E-12 0.000000E+00 9.00000E+100
86 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.037827E-12 0.000000E+00 9.00000E+100
87 phase1.collocation_constraint.defects:S e 0.000000E+00 -7.168483E-13 0.000000E+00 9.00000E+100
88 phase1.collocation_constraint.defects:S e 0.000000E+00 1.143134E-13 0.000000E+00 9.00000E+100
89 phase1.collocation_constraint.defects:S e 0.000000E+00 1.573076E-12 0.000000E+00 9.00000E+100
90 phase1.collocation_constraint.defects:S e 0.000000E+00 3.704579E-12 0.000000E+00 9.00000E+100
91 phase1.collocation_constraint.defects:S e 0.000000E+00 6.368596E-12 0.000000E+00 9.00000E+100
92 phase1.collocation_constraint.defects:S e 0.000000E+00 9.203734E-12 0.000000E+00 9.00000E+100
93 phase1.collocation_constraint.defects:S e 0.000000E+00 1.168699E-11 0.000000E+00 9.00000E+100
94 phase1.collocation_constraint.defects:S e 0.000000E+00 1.328575E-11 0.000000E+00 9.00000E+100
95 phase1.collocation_constraint.defects:S e 0.000000E+00 1.368614E-11 0.000000E+00 9.00000E+100
96 phase1.collocation_constraint.defects:S e 0.000000E+00 1.458342E-11 0.000000E+00 9.00000E+100
97 phase1.collocation_constraint.defects:S e 0.000000E+00 1.644516E-11 0.000000E+00 9.00000E+100
98 phase1.collocation_constraint.defects:S e 0.000000E+00 1.876301E-11 0.000000E+00 9.00000E+100
99 phase1.collocation_constraint.defects:S e 0.000000E+00 2.097980E-11 0.000000E+00 9.00000E+100
100 phase1.collocation_constraint.defects:S e 0.000000E+00 2.267977E-11 0.000000E+00 9.00000E+100
101 phase1.collocation_constraint.defects:S e 0.000000E+00 2.371030E-11 0.000000E+00 9.00000E+100
102 phase1.collocation_constraint.defects:S e 0.000000E+00 2.417222E-11 0.000000E+00 9.00000E+100
103 phase1.collocation_constraint.defects:S e 0.000000E+00 2.430434E-11 0.000000E+00 9.00000E+100
104 phase1.collocation_constraint.defects:S e 0.000000E+00 2.432218E-11 0.000000E+00 9.00000E+100
105 phase1.collocation_constraint.defects:S e 0.000000E+00 2.433386E-11 0.000000E+00 9.00000E+100
106 phase1.collocation_constraint.defects:S e 0.000000E+00 2.421960E-11 0.000000E+00 9.00000E+100
107 phase1.collocation_constraint.defects:S e 0.000000E+00 2.371425E-11 0.000000E+00 9.00000E+100
108 phase1.collocation_constraint.defects:S e 0.000000E+00 2.260201E-11 0.000000E+00 9.00000E+100
109 phase1.collocation_constraint.defects:S e 0.000000E+00 2.086942E-11 0.000000E+00 9.00000E+100
110 phase1.collocation_constraint.defects:S e 0.000000E+00 1.877520E-11 0.000000E+00 9.00000E+100
111 phase1.collocation_constraint.defects:S e 0.000000E+00 1.680124E-11 0.000000E+00 9.00000E+100
112 phase1.collocation_constraint.defects:S e 0.000000E+00 1.547637E-11 0.000000E+00 9.00000E+100
113 phase1.collocation_constraint.defects:S e 0.000000E+00 1.514292E-11 0.000000E+00 9.00000E+100
114 phase1.collocation_constraint.defects:S e 0.000000E+00 1.500795E-11 0.000000E+00 9.00000E+100
115 phase1.collocation_constraint.defects:S e 0.000000E+00 1.471062E-11 0.000000E+00 9.00000E+100
116 phase1.collocation_constraint.defects:S e 0.000000E+00 1.428747E-11 0.000000E+00 9.00000E+100
117 phase1.collocation_constraint.defects:S e 0.000000E+00 1.378725E-11 0.000000E+00 9.00000E+100
118 phase1.collocation_constraint.defects:S e 0.000000E+00 1.327260E-11 0.000000E+00 9.00000E+100
119 phase1.collocation_constraint.defects:S e 0.000000E+00 1.280296E-11 0.000000E+00 9.00000E+100
120 phase1.collocation_constraint.defects:S e 0.000000E+00 1.243728E-11 0.000000E+00 9.00000E+100
121 phase1.collocation_constraint.defects:S e 0.000000E+00 1.221987E-11 0.000000E+00 9.00000E+100
122 phase1.collocation_constraint.defects:S e 0.000000E+00 1.215999E-11 0.000000E+00 9.00000E+100
123 phase1.collocation_constraint.defects:S e 0.000000E+00 1.203372E-11 0.000000E+00 9.00000E+100
124 phase1.collocation_constraint.defects:S e 0.000000E+00 1.173638E-11 0.000000E+00 9.00000E+100
125 phase1.collocation_constraint.defects:S e 0.000000E+00 1.131316E-11 0.000000E+00 9.00000E+100
126 phase1.collocation_constraint.defects:S e 0.000000E+00 1.081169E-11 0.000000E+00 9.00000E+100
127 phase1.collocation_constraint.defects:S e 0.000000E+00 1.029672E-11 0.000000E+00 9.00000E+100
128 phase1.collocation_constraint.defects:S e 0.000000E+00 9.825384E-12 0.000000E+00 9.00000E+100
129 phase1.collocation_constraint.defects:S e 0.000000E+00 9.461426E-12 0.000000E+00 9.00000E+100
130 phase1.collocation_constraint.defects:S e 0.000000E+00 9.239989E-12 0.000000E+00 9.00000E+100
131 phase1.collocation_constraint.defects:S e 0.000000E+00 9.184508E-12 0.000000E+00 9.00000E+100
132 phase1.collocation_constraint.defects:S e 0.000000E+00 9.084684E-12 0.000000E+00 9.00000E+100
133 phase1.collocation_constraint.defects:S e 0.000000E+00 8.855517E-12 0.000000E+00 9.00000E+100
134 phase1.collocation_constraint.defects:S e 0.000000E+00 8.544612E-12 0.000000E+00 9.00000E+100
135 phase1.collocation_constraint.defects:S e 0.000000E+00 8.197390E-12 0.000000E+00 9.00000E+100
136 phase1.collocation_constraint.defects:S e 0.000000E+00 7.861137E-12 0.000000E+00 9.00000E+100
137 phase1.collocation_constraint.defects:S e 0.000000E+00 7.574574E-12 0.000000E+00 9.00000E+100
138 phase1.collocation_constraint.defects:S e 0.000000E+00 7.362707E-12 0.000000E+00 9.00000E+100
139 phase1.collocation_constraint.defects:S e 0.000000E+00 7.251571E-12 0.000000E+00 9.00000E+100
140 phase1.collocation_constraint.defects:S e 0.000000E+00 7.214027E-12 0.000000E+00 9.00000E+100
141 phase1.collocation_constraint.defects:S e 0.000000E+00 7.145172E-12 0.000000E+00 9.00000E+100
142 phase1.collocation_constraint.defects:S e 0.000000E+00 6.996885E-12 0.000000E+00 9.00000E+100
143 phase1.collocation_constraint.defects:S e 0.000000E+00 6.792453E-12 0.000000E+00 9.00000E+100
144 phase1.collocation_constraint.defects:S e 0.000000E+00 6.571458E-12 0.000000E+00 9.00000E+100
145 phase1.collocation_constraint.defects:S e 0.000000E+00 6.367492E-12 0.000000E+00 9.00000E+100
146 phase1.collocation_constraint.defects:S e 0.000000E+00 6.198838E-12 0.000000E+00 9.00000E+100
147 phase1.collocation_constraint.defects:S e 0.000000E+00 6.089567E-12 0.000000E+00 9.00000E+100
148 phase1.collocation_constraint.defects:S e 0.000000E+00 6.011190E-12 0.000000E+00 9.00000E+100
149 phase1.collocation_constraint.defects:S e 0.000000E+00 6.008982E-12 0.000000E+00 9.00000E+100
150 phase1.collocation_constraint.defects:S e 0.000000E+00 5.923440E-12 0.000000E+00 9.00000E+100
151 phase1.collocation_constraint.defects:S e 0.000000E+00 5.743866E-12 0.000000E+00 9.00000E+100
152 phase1.collocation_constraint.defects:S e 0.000000E+00 5.517276E-12 0.000000E+00 9.00000E+100
153 phase1.collocation_constraint.defects:S e 0.000000E+00 5.280821E-12 0.000000E+00 9.00000E+100
154 phase1.collocation_constraint.defects:S e 0.000000E+00 5.082254E-12 0.000000E+00 9.00000E+100
155 phase1.collocation_constraint.defects:S e 0.000000E+00 4.937672E-12 0.000000E+00 9.00000E+100
156 phase1.collocation_constraint.defects:S e 0.000000E+00 4.849677E-12 0.000000E+00 9.00000E+100
157 phase1.collocation_constraint.defects:S e 0.000000E+00 4.803004E-12 0.000000E+00 9.00000E+100
158 phase1.collocation_constraint.defects:S e 0.000000E+00 4.789263E-12 0.000000E+00 9.00000E+100
159 phase1.collocation_constraint.defects:S e 0.000000E+00 4.782343E-12 0.000000E+00 9.00000E+100
160 phase1.collocation_constraint.defects:S e 0.000000E+00 4.739891E-12 0.000000E+00 9.00000E+100
161 phase1.collocation_constraint.defects:S e 0.000000E+00 4.715499E-12 0.000000E+00 9.00000E+100
162 phase1.collocation_constraint.defects:S e 0.000000E+00 4.720162E-12 0.000000E+00 9.00000E+100
163 phase1.collocation_constraint.defects:S e 0.000000E+00 4.764282E-12 0.000000E+00 9.00000E+100
164 phase1.collocation_constraint.defects:S e 0.000000E+00 4.844573E-12 0.000000E+00 9.00000E+100
165 phase1.collocation_constraint.defects:S e 0.000000E+00 4.931538E-12 0.000000E+00 9.00000E+100
166 phase1.collocation_constraint.defects:S e 0.000000E+00 4.992197E-12 0.000000E+00 9.00000E+100
167 phase1.collocation_constraint.defects:S e 0.000000E+00 5.009031E-12 0.000000E+00 9.00000E+100
168 phase1.collocation_constraint.defects:S e 0.000000E+00 5.081862E-12 0.000000E+00 9.00000E+100
169 phase1.collocation_constraint.defects:S e 0.000000E+00 5.232971E-12 0.000000E+00 9.00000E+100
170 phase1.collocation_constraint.defects:S e 0.000000E+00 5.434924E-12 0.000000E+00 9.00000E+100
171 phase1.collocation_constraint.defects:S e 0.000000E+00 5.630399E-12 0.000000E+00 9.00000E+100
172 phase1.collocation_constraint.defects:S e 0.000000E+00 5.798097E-12 0.000000E+00 9.00000E+100
173 phase1.collocation_constraint.defects:S e 0.000000E+00 5.910582E-12 0.000000E+00 9.00000E+100
174 phase1.collocation_constraint.defects:S e 0.000000E+00 5.976689E-12 0.000000E+00 9.00000E+100
175 phase1.collocation_constraint.defects:S e 0.000000E+00 6.004418E-12 0.000000E+00 9.00000E+100
176 phase1.collocation_constraint.defects:S e 0.000000E+00 6.009865E-12 0.000000E+00 9.00000E+100
177 phase1.collocation_constraint.defects:S e 0.000000E+00 6.032294E-12 0.000000E+00 9.00000E+100
178 phase1.collocation_constraint.defects:S e 0.000000E+00 6.052170E-12 0.000000E+00 9.00000E+100
179 phase1.collocation_constraint.defects:S e 0.000000E+00 6.057667E-12 0.000000E+00 9.00000E+100
180 phase1.collocation_constraint.defects:S e 0.000000E+00 6.038036E-12 0.000000E+00 9.00000E+100
181 phase1.collocation_constraint.defects:S e 0.000000E+00 5.970800E-12 0.000000E+00 9.00000E+100
182 phase1.collocation_constraint.defects:S e 0.000000E+00 5.876424E-12 0.000000E+00 9.00000E+100
183 phase1.collocation_constraint.defects:S e 0.000000E+00 5.784060E-12 0.000000E+00 9.00000E+100
184 phase1.collocation_constraint.defects:S e 0.000000E+00 5.693709E-12 0.000000E+00 9.00000E+100
185 phase1.collocation_constraint.defects:S e 0.000000E+00 5.690470E-12 0.000000E+00 9.00000E+100
186 phase1.collocation_constraint.defects:S e 0.000000E+00 5.643405E-12 0.000000E+00 9.00000E+100
187 phase1.collocation_constraint.defects:S e 0.000000E+00 5.527484E-12 0.000000E+00 9.00000E+100
188 phase1.collocation_constraint.defects:S e 0.000000E+00 5.350560E-12 0.000000E+00 9.00000E+100
189 phase1.collocation_constraint.defects:S e 0.000000E+00 5.134669E-12 0.000000E+00 9.00000E+100
190 phase1.collocation_constraint.defects:S e 0.000000E+00 4.896889E-12 0.000000E+00 9.00000E+100
191 phase1.collocation_constraint.defects:S e 0.000000E+00 4.663625E-12 0.000000E+00 9.00000E+100
192 phase1.collocation_constraint.defects:S e 0.000000E+00 4.470407E-12 0.000000E+00 9.00000E+100
193 phase1.collocation_constraint.defects:S e 0.000000E+00 4.357480E-12 0.000000E+00 9.00000E+100
194 phase1.collocation_constraint.defects:S e 0.000000E+00 4.320427E-12 0.000000E+00 9.00000E+100
195 phase1.collocation_constraint.defects:S e 0.000000E+00 4.251129E-12 0.000000E+00 9.00000E+100
196 phase1.collocation_constraint.defects:S e 0.000000E+00 4.087211E-12 0.000000E+00 9.00000E+100
197 phase1.collocation_constraint.defects:S e 0.000000E+00 3.835935E-12 0.000000E+00 9.00000E+100
198 phase1.collocation_constraint.defects:S e 0.000000E+00 3.533422E-12 0.000000E+00 9.00000E+100
199 phase1.collocation_constraint.defects:S e 0.000000E+00 3.203671E-12 0.000000E+00 9.00000E+100
200 phase1.collocation_constraint.defects:S e 0.000000E+00 2.884620E-12 0.000000E+00 9.00000E+100
201 phase1.collocation_constraint.defects:S e 0.000000E+00 2.645564E-12 0.000000E+00 9.00000E+100
202 phase1.collocation_constraint.defects:S e 0.000000E+00 2.471781E-12 0.000000E+00 9.00000E+100
203 phase1.collocation_constraint.defects:S e 0.000000E+00 2.443758E-12 0.000000E+00 9.00000E+100
204 phase1.collocation_constraint.defects:S e 0.000000E+00 2.420593E-12 0.000000E+00 9.00000E+100
205 phase1.collocation_constraint.defects:S e 0.000000E+00 2.356498E-12 0.000000E+00 9.00000E+100
206 phase1.collocation_constraint.defects:S e 0.000000E+00 2.256528E-12 0.000000E+00 9.00000E+100
207 phase1.collocation_constraint.defects:S e 0.000000E+00 2.148508E-12 0.000000E+00 9.00000E+100
208 phase1.collocation_constraint.defects:S e 0.000000E+00 2.019926E-12 0.000000E+00 9.00000E+100
209 phase1.collocation_constraint.defects:S e 0.000000E+00 1.910827E-12 0.000000E+00 9.00000E+100
210 phase1.collocation_constraint.defects:S e 0.000000E+00 1.820917E-12 0.000000E+00 9.00000E+100
211 phase1.collocation_constraint.defects:S e 0.000000E+00 1.757411E-12 0.000000E+00 9.00000E+100
212 phase1.collocation_constraint.defects:S e 0.000000E+00 1.753583E-12 0.000000E+00 9.00000E+100
213 phase1.collocation_constraint.defects:S e 0.000000E+00 1.721241E-12 0.000000E+00 9.00000E+100
214 phase1.collocation_constraint.defects:S e 0.000000E+00 1.646447E-12 0.000000E+00 9.00000E+100
215 phase1.collocation_constraint.defects:S e 0.000000E+00 1.532538E-12 0.000000E+00 9.00000E+100
216 phase1.collocation_constraint.defects:S e 0.000000E+00 1.408373E-12 0.000000E+00 9.00000E+100
217 phase1.collocation_constraint.defects:S e 0.000000E+00 1.271447E-12 0.000000E+00 9.00000E+100
218 phase1.collocation_constraint.defects:S e 0.000000E+00 1.134325E-12 0.000000E+00 9.00000E+100
219 phase1.collocation_constraint.defects:S e 0.000000E+00 1.050206E-12 0.000000E+00 9.00000E+100
220 phase1.collocation_constraint.defects:S e 0.000000E+00 9.660877E-13 0.000000E+00 9.00000E+100
221 phase1.collocation_constraint.defects:S e 0.000000E+00 9.754615E-13 0.000000E+00 9.00000E+100
222 phase1.collocation_constraint.defects:S e 0.000000E+00 8.821654E-13 0.000000E+00 9.00000E+100
223 phase1.collocation_constraint.defects:S e 0.000000E+00 6.662741E-13 0.000000E+00 9.00000E+100
224 phase1.collocation_constraint.defects:S e 0.000000E+00 3.803495E-13 0.000000E+00 9.00000E+100
225 phase1.collocation_constraint.defects:S e 0.000000E+00 5.987437E-14 0.000000E+00 9.00000E+100
226 phase1.collocation_constraint.defects:S e 0.000000E+00 -2.285042E-13 0.000000E+00 9.00000E+100
227 phase1.collocation_constraint.defects:S e 0.000000E+00 -4.929329E-13 0.000000E+00 9.00000E+100
228 phase1.collocation_constraint.defects:S e 0.000000E+00 -6.714763E-13 0.000000E+00 9.00000E+100
229 phase1.collocation_constraint.defects:S e 0.000000E+00 -8.288183E-13 0.000000E+00 9.00000E+100
230 phase1.collocation_constraint.defects:S e 0.000000E+00 -7.858266E-13 0.000000E+00 9.00000E+100
231 phase1.collocation_constraint.defects:S e 0.000000E+00 -8.598352E-13 0.000000E+00 9.00000E+100
232 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.005693E-12 0.000000E+00 9.00000E+100
233 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.191696E-12 0.000000E+00 9.00000E+100
234 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.378288E-12 0.000000E+00 9.00000E+100
235 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.553004E-12 0.000000E+00 9.00000E+100
236 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.698960E-12 0.000000E+00 9.00000E+100
237 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.810365E-12 0.000000E+00 9.00000E+100
238 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.880153E-12 0.000000E+00 9.00000E+100
239 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.884472E-12 0.000000E+00 9.00000E+100
240 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.890165E-12 0.000000E+00 9.00000E+100
241 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.923832E-12 0.000000E+00 9.00000E+100
242 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.934433E-12 0.000000E+00 9.00000E+100
243 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.923243E-12 0.000000E+00 9.00000E+100
244 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.885257E-12 0.000000E+00 9.00000E+100
245 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.790146E-12 0.000000E+00 9.00000E+100
246 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.714468E-12 0.000000E+00 9.00000E+100
247 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.655085E-12 0.000000E+00 9.00000E+100
248 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.611308E-12 0.000000E+00 9.00000E+100
249 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.584708E-12 0.000000E+00 9.00000E+100
250 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.487240E-12 0.000000E+00 9.00000E+100
251 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.307126E-12 0.000000E+00 9.00000E+100
252 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.078818E-12 0.000000E+00 9.00000E+100
253 phase1.collocation_constraint.defects:S e 0.000000E+00 -7.948568E-13 0.000000E+00 9.00000E+100
254 phase1.collocation_constraint.defects:S e 0.000000E+00 -5.072635E-13 0.000000E+00 9.00000E+100
255 phase1.collocation_constraint.defects:S e 0.000000E+00 -2.542207E-13 0.000000E+00 9.00000E+100
256 phase1.collocation_constraint.defects:S e 0.000000E+00 -1.103259E-13 0.000000E+00 9.00000E+100
257 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
258 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
259 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
260 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
261 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
262 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
263 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
264 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
265 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
266 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
267 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
268 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
269 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
270 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
271 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
272 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
273 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
274 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
275 phase1.continuity_comp.defect_states:S e 0.000000E+00 0.000000E+00 0.000000E+00 9.00000E+100
Exit Status
Inform Description
0 Optimization terminated successfully.
--------------------------------------------------------------------------------
/usr/share/miniconda/envs/test/lib/python3.11/site-packages/openmdao/visualization/opt_report/opt_report.py:625: UserWarning: Attempting to set identical low and high ylims makes transformation singular; automatically expanding.
ax.set_ylim([ymin_plot, ymax_plot])
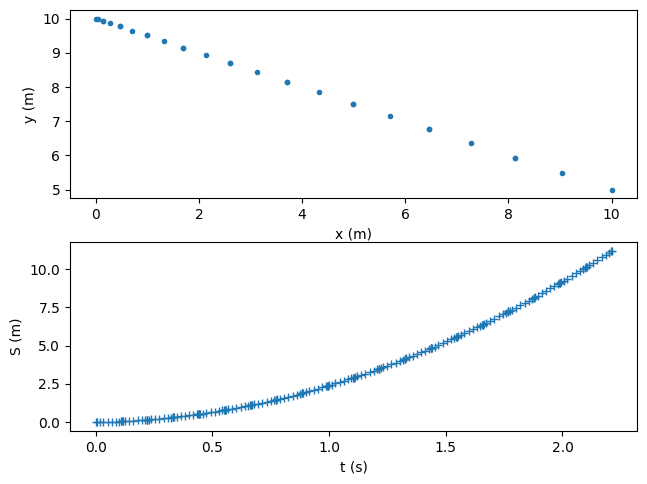